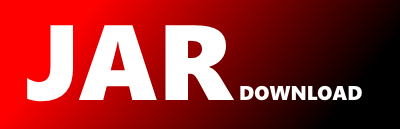
com.jamieswhiteshirt.rtree3i.Bucket Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rtree-3i-lite Show documentation
Show all versions of rtree-3i-lite Show documentation
Immutable map applying a spatial index to keys based on R-Trees
The newest version!
package com.jamieswhiteshirt.rtree3i;
import java.util.Collections;
import java.util.List;
import java.util.function.BiFunction;
import java.util.function.Consumer;
import java.util.function.Predicate;
final class Bucket {
private final Box box;
private final List> entries;
static Bucket of(Box box, Entry entry) {
return of(box, Collections.singletonList(entry));
}
static Bucket of(Box box, List> entries) {
return new Bucket<>(box, entries);
}
private Bucket(Box box, List> entries) {
this.box = box;
this.entries = entries;
}
public Box getBox() {
return box;
}
public Bucket put(Entry entry) {
for (Entry existingEntry : entries) {
if (existingEntry.getKey().equals(entry.getKey())) {
return new Bucket<>(box, Util.replace(entries, existingEntry, entry));
}
}
return new Bucket<>(box, Util.add(entries, entry));
}
public Bucket remove(Entry entry) {
if (entries.size() == 1) {
if (entries.get(0).equals(entry)) return null;
} else {
return new Bucket<>(box, Util.remove(entries, entry));
}
return this;
}
public Bucket remove(K key) {
if (entries.size() == 1) {
if (entries.get(0).getKey().equals(key)) return null;
} else {
for (Entry entry : entries) {
if (entry.getKey().equals(key)) {
return new Bucket<>(box, Util.remove(entries, entry));
}
}
}
return this;
}
public Entry get(K key) {
for (Entry entry : entries) {
if (entry.getKey().equals(key)) {
return entry;
}
}
return null;
}
public void forEach(Consumer super Entry> action) {
entries.forEach(action);
}
public boolean anyMatch(Predicate super Entry> entryPredicate) {
for (final Entry entry : entries) {
if (entryPredicate.test(entry)) {
return true;
}
}
return false;
}
public boolean allMatch(Predicate super Entry> entryPredicate) {
for (final Entry entry : entries) {
if (!entryPredicate.test(entry)) {
return false;
}
}
return true;
}
public T reduce(T identity, BiFunction, T> operator) {
T acc = identity;
for (final Entry entry : entries) {
acc = operator.apply(acc, entry);
}
return acc;
}
public int count(Predicate super Entry> entryPredicate) {
int count = 0;
for (final Entry entry : entries) {
if (entryPredicate.test(entry)) {
count++;
}
}
return count;
}
public boolean contains(Entry entry) {
return entries.contains(entry);
}
public int size() {
return entries.size();
}
@Override
public String toString() {
return "Bucket{" +
"box=" + box +
", entries=" + entries +
'}';
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy