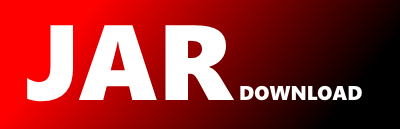
com.jamieswhiteshirt.rtree3i.Util Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rtree-3i-lite Show documentation
Show all versions of rtree-3i-lite Show documentation
Immutable map applying a spatial index to keys based on R-Trees
The newest version!
package com.jamieswhiteshirt.rtree3i;
import java.util.ArrayList;
import java.util.Collection;
import java.util.List;
import com.google.common.base.Preconditions;
/**
* @author dxm
*
*/
final class Util {
private Util() {
// prevent instantiation
}
/**
* Returns the minimum bounding box of a number of items. Benchmarks
* below indicate that when the number of items is >1 this method is more
* performant than one using {@link Box#add(Box)}.
*
* @param items
* items to bound
* @return the minimum bounding box containings items
*/
static Box mbb(Collection items) {
Preconditions.checkArgument(!items.isEmpty());
int minX1 = Integer.MAX_VALUE;
int minY1 = Integer.MAX_VALUE;
int minZ1 = Integer.MAX_VALUE;
int maxX2 = -Integer.MAX_VALUE;
int maxY2 = -Integer.MAX_VALUE;
int maxZ2 = -Integer.MAX_VALUE;
for (final Box r : items) {
if (r.x1() < minX1)
minX1 = r.x1();
if (r.y1() < minY1)
minY1 = r.y1();
if (r.z1() < minZ1)
minZ1 = r.z1();
if (r.x2() > maxX2)
maxX2 = r.x2();
if (r.y2() > maxY2)
maxY2 = r.y2();
if (r.z2() > maxZ2)
maxZ2 = r.z2();
}
return Box.create(minX1, minY1, minZ1, maxX2, maxY2, maxZ2);
}
static List add(List list, T element) {
final ArrayList result = new ArrayList<>(list.size() + 1);
result.addAll(list);
result.add(element);
return result;
}
static List remove(List extends T> list, List extends T> elements) {
final ArrayList result = new ArrayList<>(list);
result.removeAll(elements);
return result;
}
static List remove(List extends T> list, T element) {
final ArrayList result = new ArrayList<>(list);
result.remove(element);
return result;
}
static List replace(List extends T> list, T toReplace, T replacement) {
List list2 = new ArrayList<>(list.size());
for (T element : list) {
list2.add(element != toReplace ? element : replacement);
}
return list2;
}
static List replace(List list, T toReplace, List replacements) {
List list2 = new ArrayList<>(list.size() - 1 + replacements.size());
for (T node : list) {
if (node != toReplace) {
list2.add(node);
}
}
list2.addAll(replacements);
return list2;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy