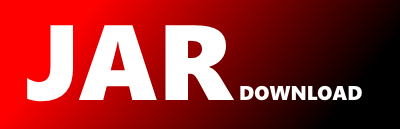
cn.afterturn.easypoi.word.parse.excel.ExcelMapParse Maven / Gradle / Ivy
/**
* Copyright 2013-2015 JueYue ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License"); you may not use this file except
* in compliance with the License. You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software distributed under the License
* is distributed on an "AS IS" BASIS, WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express
* or implied. See the License for the specific language governing permissions and limitations under
* the License.
*/
package cn.afterturn.easypoi.word.parse.excel;
import cn.afterturn.easypoi.entity.ImageEntity;
import cn.afterturn.easypoi.excel.entity.params.ExcelForEachParams;
import cn.afterturn.easypoi.util.PoiPublicUtil;
import cn.afterturn.easypoi.util.PoiWordStyleUtil;
import cn.afterturn.easypoi.word.entity.MyXWPFDocument;
import com.google.common.collect.Maps;
import org.apache.poi.xwpf.usermodel.*;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.math.BigInteger;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import static cn.afterturn.easypoi.util.PoiElUtil.*;
/**
* 处理和生成Map 类型的数据变成表格
*
* @author JueYue
* 2014年8月9日 下午10:28:46
*/
public final class ExcelMapParse {
private static final Logger LOGGER = LoggerFactory.getLogger(ExcelMapParse.class);
/**
* 添加图片
*
* @param obj
* @param currentRun
* @throws Exception
* @author JueYue
* 2013-11-20
*/
public static void addAnImage(ImageEntity obj, XWPFRun currentRun) {
try {
Object[] isAndType = PoiPublicUtil.getIsAndType(obj);
String picId;
picId = currentRun.getDocument().addPictureData((byte[]) isAndType[0],
(Integer) isAndType[1]);
if (obj.getLocationType() == ImageEntity.EMBED) {
((MyXWPFDocument) currentRun.getDocument()).createPicture(currentRun,
picId, currentRun.getDocument()
.getNextPicNameNumber((Integer) isAndType[1]),
obj.getWidth(), obj.getHeight());
} else if (obj.getLocationType() == ImageEntity.ABOVE) {
((MyXWPFDocument) currentRun.getDocument()).createPicture(currentRun,
picId, currentRun.getDocument()
.getNextPicNameNumber((Integer) isAndType[1]),
obj.getWidth(), obj.getHeight(), false);
} else if (obj.getLocationType() == ImageEntity.BEHIND) {
((MyXWPFDocument) currentRun.getDocument()).createPicture(currentRun,
picId, currentRun.getDocument()
.getNextPicNameNumber((Integer) isAndType[1]),
obj.getWidth(), obj.getHeight(), true);
}
} catch (Exception e) {
LOGGER.error(e.getMessage(), e);
}
}
private static void addAnImage(ImageEntity obj, XWPFTableCell cell) {
List paragraphs = cell.getParagraphs();
XWPFParagraph newPara = paragraphs.get(0);
XWPFRun imageCellRun = newPara.createRun();
addAnImage(obj,imageCellRun);
}
/**
* 解析参数行,获取参数列表
*
* @param currentRow
* @return
* @author JueYue
* 2013-11-18
*/
private static String[] parseCurrentRowGetParams(XWPFTableRow currentRow) {
List cells = currentRow.getTableCells();
String[] params = new String[cells.size()];
String text;
for (int i = 0; i < cells.size(); i++) {
text = cells.get(i).getText();
params[i] = text == null ? ""
: text.trim().replace(START_STR, EMPTY).replace(END_STR, EMPTY);
}
return params;
}
/**
* 解析参数行,获取参数列表
*
* @param currentRow
* @return
* @author JueYue
* 2013-11-18
*/
private static List parseCurrentRowGetParamsEntity(XWPFTableRow currentRow) {
List cells = currentRow.getTableCells();
List params = new ArrayList<>();
String text;
for (int i = 0; i < cells.size(); i++) {
ExcelForEachParams param = new ExcelForEachParams();
text = cells.get(i).getText();
param.setName(text == null ? ""
: text.trim().replace(START_STR, EMPTY).replace(END_STR, EMPTY));
if (cells.get(i).getCTTc().getTcPr().getGridSpan() != null) {
if (cells.get(i).getCTTc().getTcPr().getGridSpan().getVal() != null) {
param.setColspan(cells.get(i).getCTTc().getTcPr().getGridSpan().getVal().intValue());
}
}
param.setHeight((short) currentRow.getHeight());
params.add(param);
}
return params;
}
/**
* 解析下一行,并且生成更多的行
*
* @param table
* @param index
* @param list
*/
public static void parseNextRowAndAddRow(XWPFTable table, int index,
List