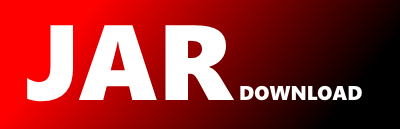
com.janeluo.jfinalplus.kit.ModelKit Maven / Gradle / Ivy
/**
* Copyright (c) 2011-2013, kidzhou 周磊 ([email protected])
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.janeluo.jfinalplus.kit;
import com.google.common.base.Preconditions;
import com.google.common.collect.Lists;
import com.google.common.collect.Maps;
import com.jfinal.log.Log;
import com.jfinal.plugin.activerecord.*;
import java.util.List;
import java.util.Map;
import java.util.Map.Entry;
import java.util.Set;
public class ModelKit {
protected final static Log logger = Log.getLog(ModelKit.class);
public static Record toRecord(Model> model) {
return model.toRecord();
}
@SuppressWarnings("rawtypes")
public static Model set(Model model, Object... attrsAndValues) {
int length = attrsAndValues.length;
Preconditions.checkArgument(length % 2 == 0, "attrsAndValues length must be even number", length);
for (int i = 0; i < length; i = i + 2) {
Object attr = attrsAndValues[i];
Preconditions.checkArgument(attr instanceof String, "the odd number of attrsAndValues must be String");
model.set((String) attr, attrsAndValues[i + 1]);
}
return model;
}
@SuppressWarnings({ "rawtypes", "unchecked" })
public static Map toMap(Model model) {
Map map = Maps.newHashMap();
Set> attrs = model._getAttrsEntrySet();
for (Entry entry : attrs) {
map.put(entry.getKey(), entry.getValue());
}
return map;
}
public static Model> fromBean(Class extends Model>> clazz, Object bean) {
Model> model = null;
try {
model = clazz.newInstance();
} catch (Exception e) {
logger.error(e.getMessage(), e);
return model;
}
return model;
}
@SuppressWarnings("rawtypes")
public static int[] batchSave(List extends Model> data) {
return batchSave(data, data.size());
}
@SuppressWarnings("rawtypes")
public static int[] batchSave(List extends Model> data, int batchSize) {
Model model = data.get(0);
Map attrs = Reflect.on(model).field("attrs").get();
Class extends Model> modelClass = model.getClass();
Table tableInfo = TableMapping.me().getTable(modelClass);
StringBuilder sql = new StringBuilder();
List
© 2015 - 2024 Weber Informatics LLC | Privacy Policy