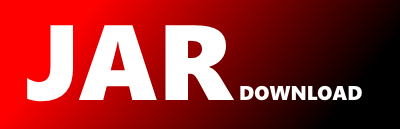
com.jashmore.sqs.extensions.registry.SpringCloudSchemaArgumentResolver Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of spring-cloud-schema-registry-extension-api Show documentation
Show all versions of spring-cloud-schema-registry-extension-api Show documentation
API for building a payload parser that has been serialized via the schema registered in the Spring Cloud Schema Registry
package com.jashmore.sqs.extensions.registry;
import com.jashmore.sqs.QueueProperties;
import com.jashmore.sqs.argument.ArgumentResolutionException;
import com.jashmore.sqs.argument.ArgumentResolver;
import com.jashmore.sqs.argument.MethodParameter;
import com.jashmore.sqs.util.annotation.AnnotationUtils;
import org.springframework.cloud.schema.registry.SchemaReference;
import software.amazon.awssdk.services.sqs.model.Message;
import java.lang.annotation.Annotation;
/**
* Argument resolver for taking messages that were serialized using a schema versioning tool like Apache Avro.
*
* This will obtain the schema of the object that the producer used to serialize the object and the schema that the
* consumer can consume and serialize the message payload between these versions.
*
* @param the spring cloud registry schema type used to resolve this argument
*/
public class SpringCloudSchemaArgumentResolver implements ArgumentResolver
© 2015 - 2025 Weber Informatics LLC | Privacy Policy