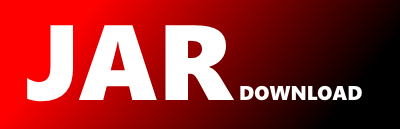
com.ja.smarkdown.location.file.FileListingProvider Maven / Gradle / Ivy
package com.ja.smarkdown.location.file;
import java.io.IOException;
import java.nio.file.FileVisitResult;
import java.nio.file.Files;
import java.nio.file.Path;
import java.nio.file.Paths;
import java.nio.file.SimpleFileVisitor;
import java.nio.file.attribute.BasicFileAttributes;
import java.util.ArrayList;
import java.util.List;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.StringUtils;
import com.ja.smarkdown.load.AbstractListingProvider;
import com.ja.smarkdown.load.MountPointUtil;
import com.ja.smarkdown.model.config.Location;
@Slf4j
public class FileListingProvider extends AbstractListingProvider {
private static final String PROTOCOL = "file://";
@Override
protected List getDocuments(final Location location) {
final List result = new ArrayList();
try {
final String base = StringUtils.removeStart(location.getUrl(),
PROTOCOL);
Files.walkFileTree(Paths.get(base), new SimpleFileVisitor() {
@Override
public FileVisitResult visitFile(final Path file,
final BasicFileAttributes attrs) throws IOException {
log.debug("Visiting {}", file);
if (file.toString().endsWith(".md")) {
log.debug("accepting file={}", file);
result.add(MountPointUtil.apply(location,
StringUtils.removeStart(file.toString(), base)));
}
return super.visitFile(file, attrs);
}
});
} catch (final IOException e) {
log.error("failed", e);
}
return result;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy