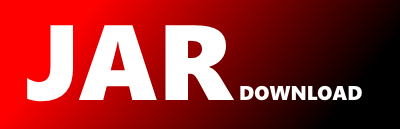
com.javabaas.JBQuery Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of javabaas_android Show documentation
Show all versions of javabaas_android Show documentation
JavaBaas, which based on Java, is a development framework for mobile backend.
package com.javabaas;
import java.util.Collection;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.Set;
import com.javabaas.callback.CountCallback;
import com.javabaas.callback.DeleteCallback;
import com.javabaas.callback.FindCallback;
import com.javabaas.exception.JBException;
import com.javabaas.util.Utils;
public class JBQuery {
private Class clazz;
private String className;
private JBQuery.CachePolicy cachePolicy = CachePolicy.NETWORK_ONLY;
private String queryPath;
private String externalQueryPath;
QueryConditions conditions;
private IObjectManager manager;
public JBQuery(String theClassName) {
this(theClassName, (Class) null);
this.className = theClassName;
}
public static JBQuery getInstance(String theClassName) {
return new JBQuery<>(theClassName, JBObject.class);
}
public JBQuery(String theClassName, Class clazz) {
this.cachePolicy = JBQuery.CachePolicy.IGNORE_CACHE;
Utils.checkClassName(theClassName);
this.className = theClassName;
this.clazz = clazz;
this.conditions = new QueryConditions();
manager = JBCloud.getObjectManager(theClassName);
}
Class getClazz() {
return this.clazz;
}
void setClazz(Class clazz) {
this.clazz = clazz;
}
List getInclude() {
return this.conditions.getInclude();
}
void setInclude(List include) {
this.conditions.setInclude(include);
}
Set getSelectedKeys() {
return this.conditions.getSelectedKeys();
}
void setSelectedKeys(Set selectedKeys) {
this.conditions.setSelectedKeys(selectedKeys);
}
Map getParameters() {
return this.conditions.getParameters();
}
void setParameters(Map parameters) {
this.conditions.setParameters(parameters);
}
String getQueryPath() {
return this.queryPath;
}
void setQueryPath(String queryPath) {
this.queryPath = queryPath;
}
String getExternalQueryPath() {
return this.externalQueryPath;
}
void setExternalQueryPath(String path) {
this.externalQueryPath = path;
}
static String getTag() {
return "com.parse.JBQuery";
}
Map> getWhere() {
return this.conditions.getWhere();
}
public String getClassName() {
return this.className;
}
public JBQuery setClassName(String className) {
this.className = className;
return this;
}
public JBQuery.CachePolicy getCachePolicy() {
return this.cachePolicy;
}
public JBQuery setCachePolicy(JBQuery.CachePolicy cachePolicy) {
this.cachePolicy = cachePolicy;
return this;
}
public JBQuery.CachePolicy getPolicy() {
return this.cachePolicy;
}
public JBQuery setPolicy(JBQuery.CachePolicy policy) {
this.cachePolicy = policy;
return this;
}
public boolean isTrace() {
return this.conditions.isTrace();
}
public JBQuery setTrace(boolean trace) {
this.conditions.setTrace(trace);
return this;
}
public int getLimit() {
return this.conditions.getLimit();
}
public JBQuery setLimit(int limit) {
this.conditions.setLimit(limit);
return this;
}
public JBQuery limit(int limit) {
this.setLimit(limit);
return this;
}
public JBQuery skip(int skip) {
this.setSkip(skip);
return this;
}
public int getSkip() {
return this.conditions.getSkip();
}
public JBQuery setSkip(int skip) {
this.conditions.setSkip(skip);
return this;
}
public String getOrder() {
return this.conditions.getOrder();
}
public JBQuery setOrder(String order) {
this.conditions.setOrder(order);
return this;
}
public JBQuery order(String order) {
this.setOrder(order);
return this;
}
public JBQuery addAscendingOrder(String key) {
this.conditions.addAscendingOrder(key);
return this;
}
public JBQuery addDescendingOrder(String key) {
this.conditions.addDescendingOrder(key);
return this;
}
public JBQuery include(String key) {
this.conditions.include(key);
return this;
}
public JBQuery selectKeys(Collection keys) {
this.conditions.selectKeys(keys);
return this;
}
public JBQuery orderByAscending(String key) {
this.conditions.orderByAscending(key);
return this;
}
public JBQuery orderByDescending(String key) {
this.conditions.orderByDescending(key);
return this;
}
public JBQuery whereContainedIn(String key, Collection extends Object> values) {
this.conditions.whereContainedIn(key, values);
return this;
}
public JBQuery whereContains(String key, String substring) {
this.conditions.whereContains(key, substring);
return this;
}
public JBQuery whereSizeEqual(String key, int size) {
this.conditions.whereSizeEqual(key, size);
return this;
}
public JBQuery whereContainsAll(String key, Collection> values) {
this.conditions.whereContainsAll(key, values);
return this;
}
public JBQuery whereDoesNotExist(String key) {
this.conditions.whereDoesNotExist(key);
return this;
}
public JBQuery whereEndsWith(String key, String suffix) {
this.conditions.whereEndsWith(key, suffix);
return this;
}
public JBQuery whereEqualTo(String key, Object value) {
this.conditions.whereEqualTo(key, value);
return this;
}
private JBQuery addWhereItem(QueryOperation op) {
this.conditions.addWhereItem(op);
return this;
}
private JBQuery addOrItems(QueryOperation op) {
this.conditions.addOrItems(op);
return this;
}
protected JBQuery addWhereItem(String key, String op, Object value) {
this.conditions.addWhereItem(key, op, value);
return this;
}
public JBQuery whereExists(String key) {
this.conditions.whereExists(key);
return this;
}
public JBQuery whereGreaterThan(String key, Object value) {
this.conditions.whereGreaterThan(key, value);
return this;
}
public JBQuery whereGreaterThanOrEqualTo(String key, Object value) {
this.conditions.whereGreaterThanOrEqualTo(key, value);
return this;
}
public JBQuery whereLessThan(String key, Object value) {
this.conditions.whereLessThan(key, value);
return this;
}
public JBQuery whereLessThanOrEqualTo(String key, Object value) {
this.conditions.whereLessThanOrEqualTo(key, value);
return this;
}
public JBQuery whereMatches(String key, String regex) {
this.conditions.whereMatches(key, regex);
return this;
}
public JBQuery whereMatches(String key, String regex, String modifiers) {
this.conditions.whereMatches(key, regex, modifiers);
return this;
}
public JBQuery whereNotContainedIn(String key, Collection extends Object> values) {
this.conditions.whereNotContainedIn(key, values);
return this;
}
public JBQuery whereNotEqualTo(String key, Object value) {
this.conditions.whereNotEqualTo(key, value);
return this;
}
public JBQuery whereStartsWith(String key, String prefix) {
this.conditions.whereStartsWith(key, prefix);
return this;
}
public static JBQuery or(List> queries) {
String className = null;
if (queries.size() > 0) {
className = ((JBQuery) queries.get(0)).getClassName();
}
JBQuery result = JBQuery.getInstance(className);
if (queries.size() > 1) {
for (Object query1 : queries) {
JBQuery query = (JBQuery) query1;
if (!className.equals(query.getClassName())) {
throw new IllegalArgumentException("All queries must be for the same class");
}
result.addOrItems(new QueryOperation("$or", "$or", query.conditions.compileWhereOperationMap()));
}
} else {
result.setWhere(((JBQuery) queries.get(0)).conditions.getWhere());
}
return result;
}
public JBQuery whereMatchesKeyInQuery(String key, String searchKey, String targetClass, JBQuery> query) {
HashMap inner = new HashMap<>();
inner.put("searchClass", query.getClassName());
inner.put("where", query.conditions.compileWhereOperationMap());
if (query.conditions.getSkip() > 0) {
inner.put("skip", query.conditions.getSkip());
}
if (query.conditions.getLimit() > 0) {
inner.put("limit", query.conditions.getLimit());
}
if (!Utils.isBlankContent(query.getOrder())) {
inner.put("order", query.getOrder());
}
inner.put("searchKey", searchKey);
inner.put("targetClass", targetClass);
return this.addWhereItem(key, "$sub", inner);
}
public JBQuery whereMatchesQuery(String key, JBQuery> query) {
Map map = Utils.createMap("where", query.conditions.compileWhereOperationMap());
map.put("className", query.className);
if (query.conditions.getSkip() > 0) {
map.put("skip", Integer.valueOf(query.conditions.getSkip()));
}
if (query.conditions.getLimit() > 0) {
map.put("limit", Integer.valueOf(query.conditions.getLimit()));
}
if (!Utils.isBlankContent(query.getOrder())) {
map.put("order", query.getOrder());
}
this.addWhereItem(key, "$inQuery", map);
return this;
}
public JBQuery whereDoesNotMatchKeyInQuery(String key, String keyInQuery, JBQuery> query) {
Map map = Utils.createMap("className", query.className);
map.put("where", query.conditions.compileWhereOperationMap());
Map queryMap = Utils.createMap("query", map);
queryMap.put("key", keyInQuery);
this.addWhereItem(key, "$dontSelect", queryMap);
return this;
}
public JBQuery whereDoesNotMatchQuery(String key, JBQuery> query) {
Map map = Utils.createMap("className", query.className);
map.put("where", query.conditions.compileWhereOperationMap());
this.addWhereItem(key, "$notInQuery", map);
return this;
}
JBQuery setWhere(Map> value) {
this.conditions.setWhere(value);
return this;
}
public void deleteAllInBackground(DeleteCallback deleteCallback){
assembleParameters();
manager.deleteByQuery(getParameters() , false , deleteCallback);
}
public void deleteAll() throws JBException{
assembleParameters();
final Object[] objects = new Object[1];
manager.deleteByQuery(getParameters(), true, new DeleteCallback() {
@Override
public void done() {
}
@Override
public void error(JBException e) {
objects[0] = e;
}
});
if (objects[0] != null)
throw (JBException) objects[0];
}
public List find() throws JBException{
assembleParameters();
final Object[] objects = new Object[2];
manager.objectQuery(getParameters(), true, new FindCallback() {
@Override
public void done(List r) {
objects[0] = r;
}
@Override
public void error(JBException e) {
objects[1] = e;
}
}, cachePolicy);
if (objects[1] != null)
throw ((JBException) objects[1]);
return (List) objects[0];
}
public void findInBackground(FindCallback callback) {
assembleParameters();
manager.objectQuery(getParameters(), false ,callback, cachePolicy);
}
public int count() throws JBException {
assembleParameters();
final Object[] objects = new Object[2];
manager.countQuery(getParameters(), true , new CountCallback() {
@Override
public void done(int count) {
objects[0] = count;
}
@Override
public void error(JBException e) {
objects[1] = e;
}
});
if (objects[1] != null)
throw ((JBException) objects[1]);
return (int) objects[0];
}
public void countInBackground(CountCallback callback) {
assembleParameters();
manager.countQuery(getParameters(), false , callback);
}
protected Map assembleParameters() {
return this.conditions.assembleParameters();
}
public enum CachePolicy {
CACHE_ELSE_NETWORK,
CACHE_ONLY,
CACHE_THEN_NETWORK,
IGNORE_CACHE,
NETWORK_ELSE_CACHE,
NETWORK_ONLY;
private CachePolicy() {
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy