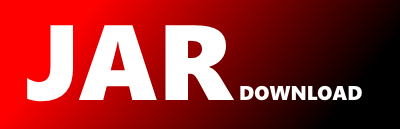
scriptella.jdbc.StatementWrapper Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2006-2012 The Scriptella Project Team.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package scriptella.jdbc;
import scriptella.spi.ParametersCallback;
import scriptella.spi.QueryCallback;
import scriptella.util.ExceptionUtils;
import scriptella.util.IOUtils;
import java.io.Closeable;
import java.sql.PreparedStatement;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.sql.Statement;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
* Abstraction for {@link java.sql.Statement} and {@link java.sql.PreparedStatement}.
*
* @author Fyodor Kupolov
* @version 1.0
*/
abstract class StatementWrapper implements Closeable {
private static final Logger LOG = Logger.getLogger(StatementWrapper.class.getName());
protected final JdbcTypesConverter converter;
protected final T statement;
/**
* For testing only.
*/
protected StatementWrapper() {
converter = null;
statement = null;
}
protected StatementWrapper(T statement, JdbcTypesConverter converter) {
if (statement == null) {
throw new IllegalArgumentException("statement cannot be null");
}
if (converter == null) {
throw new IllegalArgumentException("converter cannot be null");
}
this.statement = statement;
this.converter = converter;
}
/**
* Release any resources opened by this statement.
*/
public void close() {
JdbcUtils.closeSilent(statement);
}
/**
* Executes the given SQL statement, which may be an INSERT, UPDATE, or DELETE statement
* or an SQL statement that returns nothing, such as an SQL DDL statement.
*
* @return either the row count for INSERT, UPDATE, or DELETE statements or 0 for SQL statements that return nothing.
* @throws SQLException if JDBC driver fails to execute the operation.
*/
public abstract int update() throws SQLException;
/**
* Executes the query and returns the result set.
*
* @return result set with query result.
* @throws SQLException if JDBC driver fails to execute the operation.
*/
protected abstract ResultSet query() throws SQLException;
public void query(final QueryCallback queryCallback, final ParametersCallback parametersCallback) throws SQLException {
ResultSetAdapter r = null;
try {
r = new ResultSetAdapter(query(), parametersCallback, converter);
while (r.next()) {
queryCallback.processRow(r);
}
} finally {
IOUtils.closeSilently(r);
}
}
public void setParameters(final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy