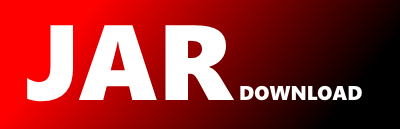
com.javanut.json.encode.JSONArray Maven / Gradle / Ivy
Show all versions of pronghorn-pipes Show documentation
package com.javanut.json.encode;
import java.util.Collection;
import java.util.Collections;
import java.util.Iterator;
import java.util.List;
import com.javanut.json.JSONType;
import com.javanut.json.encode.function.*;
/**
*
* @param Root of renderer
* @param Data source type
* @param Builder return type
* @param Iterating node
*/
public abstract class JSONArray {
final JSONBuilder builder;
private final IteratorFunction iterator;
JSONArray(JSONBuilder builder, IteratorFunction iterator) {
this.builder = builder;
this.iterator = iterator;
}
//@FunctionalInterface
public static interface ArrayCompletion {
P end();
}
static JSONArray createArray(
JSONBuilder builder,
final ToMemberFunction accessor, // Convert parent T to iteratable M
final IteratorFunction iterator, // iterate of M using N
final ArrayCompletion ending) {
return new JSONArray(
// called by builder to select null script
builder.beginArray(accessor),
// called by script to iterate over array given re-accessing M from T
iterator) {
@Override
P arrayEnded() {
return ending.end();
}
};
}
static , N> JSONArray createListArray(
JSONBuilder builder,
final ToMemberFunction accessor,
final ArrayCompletion ending) {
return new JSONArray(
builder.beginArray(accessor),
new IteratorFunction() {
@Override
public M get(M o, int i) {
return i < o.size() ? o : null;
}
}) {
@Override
P arrayEnded() {
return ending.end();
}
};
}
static JSONArray createBasicArray(
JSONBuilder builder,
final ToMemberFunction accessor,
final ArrayCompletion ending) {
return new JSONArray(
builder.beginArray(accessor),
new IteratorFunction() {
@Override
public N[] get(N[] o, int i) {
return i < o.length ? o : null;
}
}) {
@Override
P arrayEnded() {
return ending.end();
}
};
}
static , N> JSONArray, P, Iterator> createCollectionArray(
JSONBuilder builder,
final ToMemberFunction accessor,
final ArrayCompletion ending) {
return new JSONArray, P, Iterator>(
builder.beginArray(new ToMemberFunction>() {
@Override
public Iterator get(T o) {
Collection collection = accessor.get(o);
return collection != null ? collection.iterator() : null;
}
}),
new IteratorFunction, Iterator>() {
@Override
public Iterator get(Iterator o, int i) {
return o.hasNext() ? o : null;
}
}) {
@Override
P arrayEnded() {
return ending.end();
}
};
}
private P childCompleted() {
builder.endArray();
return arrayEnded();
}
abstract P arrayEnded();
// Object
@Deprecated
public JSONObject beginObject(IterMemberFunction accessor) {
return new JSONObject(builder.beginObject(iterator, accessor)) {
@Override
P objectEnded() {
return childCompleted();
}
};
}
public JSONObject startObject(IterMemberFunction accessor) {
return new JSONObject(builder.beginObject(iterator, accessor)) {
@Override
P objectEnded() {
return childCompleted();
}
};
}
// Array
public JSONArray array(IterMemberFunction accessor, IteratorFunction iterator) {
return new JSONArray(builder.beginArray(this.iterator, accessor), iterator) {
@Override
P arrayEnded() {
return childCompleted();
}
};
}
public , N2> JSONArray listArray(IterMemberFunction accessor) {
return new JSONArray(
builder.beginArray(this.iterator, accessor),
new IteratorFunction() {
@Override
public M get(M obj, int i) {
return i < obj.size() ? obj : null;
}
}) {
@Override
P arrayEnded() {
return childCompleted();
}
};
}
public JSONArray basicArray(IterMemberFunction accessor) {
return new JSONArray(
builder.beginArray(this.iterator, accessor),
new IteratorFunction() {
@Override
public N2[] get(N2[] obj, int i) {
return i < obj.length ? obj : null;
}
}) {
@Override
P arrayEnded() {
return childCompleted();
}
};
}
public , N2> JSONArray, P, Iterator> iterArray(final IterMemberFunction accessor) {
return new JSONArray, P, Iterator>(
builder.beginArray(this.iterator, new IterMemberFunction>() {
@Override
public Iterator get(T o, int i) {
Collection m = accessor.get(o, i);
return m != null ? m.iterator() : null;
}
}),
new IteratorFunction, Iterator>() {
@Override
public Iterator get(Iterator obj, int i) {
return obj.hasNext() ? obj : null;
}
}) {
@Override
P arrayEnded() {
return childCompleted();
}
};
}
// Renderer
public P renderer(JSONRenderer renderer, IterMemberFunction accessor) {
builder.addBuilder(iterator, renderer.builder, accessor);
return this.childCompleted();
}
/*
public P recurseRoot(IterMemberFunction accessor) {
builder.recurseRoot(iterator, accessor);
return this.childCompleted();
}
*/
public JSONArraySelect beginSelect() {
return new JSONArraySelect(builder.beginSelect(), iterator) {
@Override
P selectEnded() {
return childCompleted();
}
};
}
// Null
public P empty() {
return this.childCompleted();
}
public P constantNull() {
builder.addNull(iterator);
return this.childCompleted();
}
// Bool
public P bool(IterBoolFunction func) {
builder.addBool(iterator, null, func);
return this.childCompleted();
}
public P bool(IterBoolFunction func, JSONType encode) {
builder.addBool(iterator, null, func, encode);
return this.childCompleted();
}
public P nullableBool(IterBoolFunction isNull, IterBoolFunction func) {
builder.addBool(iterator, isNull, func);
return this.childCompleted();
}
public P nullableBool(IterBoolFunction isNull, IterBoolFunction func, JSONType encode) {
builder.addBool(iterator, isNull, func, encode);
return this.childCompleted();
}
// Integer
public P integer(IterLongFunction func) {
builder.addInteger(iterator, null, func);
return this.childCompleted();
}
public P integer(IterLongFunction func, JSONType encode) {
builder.addInteger(iterator, null, func, encode);
return this.childCompleted();
}
public P nullableInteger(IterBoolFunction isNull, IterLongFunction func) {
builder.addInteger(iterator, isNull, func);
return this.childCompleted();
}
public P nullableInteger(IterBoolFunction isNull, IterLongFunction func, JSONType encode) {
builder.addInteger(iterator, isNull, func, encode);
return this.childCompleted();
}
// Decimal
public P decimal(int precision, IterDoubleFunction func) {
builder.addDecimal(iterator, precision, null, func);
return this.childCompleted();
}
public P decimal(int precision, IterDoubleFunction func, JSONType encode) {
builder.addDecimal(iterator, precision, null, func, encode);
return this.childCompleted();
}
public P nullableDecimal(int precision, IterBoolFunction isNull, IterDoubleFunction func) {
builder.addDecimal(iterator, precision, isNull, func);
return this.childCompleted();
}
public P nullableDecimal(int precision, IterBoolFunction isNull, IterDoubleFunction func, JSONType encode) {
builder.addDecimal(iterator, precision, isNull, func, encode);
return this.childCompleted();
}
// String
public P string(IterStringFunction func) {
builder.addString(iterator, false, func);
return this.childCompleted();
}
public P string(IterStringFunction func, JSONType encode) {
builder.addString(iterator, false, func, encode);
return this.childCompleted();
}
public P nullableString(IterStringFunction func) {
builder.addString(iterator, true, func);
return this.childCompleted();
}
public P nullableString(IterStringFunction func, JSONType encode) {
builder.addString(iterator, true, func, encode);
return this.childCompleted();
}
// Enum
public > P enumName(IterEnumFunction func) {
builder.addEnumName(iterator, func);
return this.childCompleted();
}
public > P enumOrdinal(IterEnumFunction func) {
builder.addEnumOrdinal(iterator, func);
return this.childCompleted();
}
}