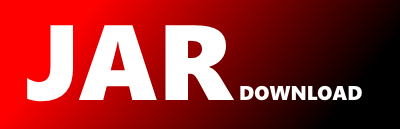
com.javanut.json.encode.NullableAppendableByteWriterWrapper Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pronghorn-pipes Show documentation
Show all versions of pronghorn-pipes Show documentation
Ring buffer based queuing utility for applications that require high performance and/or a small
footprint. Well suited for embedded and stream based processing.
package com.javanut.json.encode;
import com.javanut.pronghorn.util.AppendableByteWriter;
public class NullableAppendableByteWriterWrapper implements AppendableByteWriter {
public boolean wasNull;
public AppendableByteWriter> externalWriter;
protected boolean needsQuote;
@Override
public NullableAppendableByteWriterWrapper append(CharSequence csq) {
if (null !=csq) {
assert(!wasNull) : "Can not write text after writing a null";
if (needsQuote) {
externalWriter.writeByte('"');
needsQuote = false;
}
externalWriter.append(csq);
} else {
wasNull = true;
needsQuote = false;
}
return this;
}
@Override
public NullableAppendableByteWriterWrapper append(CharSequence csq, int start, int end) {
assert(!wasNull) : "Can not write text after writing a null";
if (needsQuote) {
externalWriter.writeByte('"');
needsQuote = false;
}
externalWriter.append(csq,start,end);
return this;
}
@Override
public NullableAppendableByteWriterWrapper append(char c) {
assert(!wasNull) : "Can not write text after writing a null";
if (needsQuote) {
externalWriter.writeByte('"');
needsQuote = false;
}
externalWriter.append(c);
return this;
}
@Override
public void write(byte[] b) {
assert(!wasNull) : "Can not write text after writing a null";
if (needsQuote) {
externalWriter.writeByte('"');
needsQuote = false;
}
externalWriter.write(b);
}
@Override
public void write(byte[] b, int pos, int len) {
assert(!wasNull) : "Can not write text after writing a null";
if (needsQuote) {
externalWriter.writeByte('"');
needsQuote = false;
}
externalWriter.write(b,pos,len);
}
@Override
public void writeByte(int asciiChar) {
assert(!wasNull) : "Can not write text after writing a null";
if (needsQuote) {
externalWriter.writeByte('"');
needsQuote = false;
}
externalWriter.writeByte(asciiChar);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy