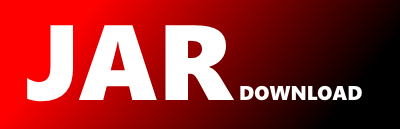
com.javanut.pronghorn.pipe.LittleEndianDataOutputBlobWriter Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pronghorn-pipes Show documentation
Show all versions of pronghorn-pipes Show documentation
Ring buffer based queuing utility for applications that require high performance and/or a small
footprint. Well suited for embedded and stream based processing.
package com.javanut.pronghorn.pipe;
import java.io.DataOutput;
import java.io.IOException;
import java.io.ObjectOutputStream;
import java.io.OutputStream;
public class LittleEndianDataOutputBlobWriter> extends OutputStream implements DataOutput {
private final Pipe p;
private final byte[] byteBuffer;
private final int byteMask;
private ObjectOutputStream oos;
private int startPosition;
private int activePosition;
public LittleEndianDataOutputBlobWriter(Pipe p) {
this.p = p;
this.byteBuffer = Pipe.blob(p);
this.byteMask = Pipe.blobMask(p);
assert(byteMask!=0);
}
public void openField() {
p.openBlobFieldWrite();
//NOTE: this method works with both high and low APIs.
startPosition = activePosition = Pipe.getWorkingBlobHeadPosition(p);
}
public int closeHighLevelField(int targetFieldLoc) {
//this method will also validate the length was in bound and throw unsupported operation if the pipe was not large enough
//instead of fail fast as soon as one field goes over we wait to the end and only check once.
int len = length();
PipeWriter.writeSpecialBytesPosAndLen(p, targetFieldLoc, len, startPosition);
p.closeBlobFieldWrite();
return len;
}
public int closeLowLevelField() {
int len = length();
Pipe.addAndGetBlobWorkingHeadPosition(p, len);
Pipe.addBytePosAndLenSpecial(p,startPosition,len);
p.closeBlobFieldWrite();
return len;
}
public int length() {
if (activePosition>=startPosition) {
return activePosition-startPosition;
} else {
return (activePosition-Integer.MIN_VALUE)+(1+Integer.MAX_VALUE-startPosition);
}
}
public byte[] toByteArray() {
byte[] result = new byte[length()];
Pipe.copyBytesFromToRing(byteBuffer, startPosition, byteMask, result, 0, Integer.MAX_VALUE, result.length);
return result;
}
public void writeObject(Object object) throws IOException {
if (null==oos) {
oos = new ObjectOutputStream(this);
}
oos.writeObject(object); //TODO:: this needs testing
oos.flush();
}
@Override
public void write(int b) throws IOException {
byteBuffer[byteMask & activePosition++] = (byte)b;
}
@Override
public void write(byte[] b) throws IOException {
write(this,b,0,b.length,Integer.MAX_VALUE);
}
@Override
public void write(byte[] b, int off, int len) throws IOException {
write(this,b,off,len,Integer.MAX_VALUE);
}
@Override
public void writeBoolean(boolean v) throws IOException {
byteBuffer[byteMask & activePosition++] = (byte) (v ? 1 : 0);
}
@Override
public void writeByte(int v) throws IOException {
byteBuffer[byteMask & activePosition++] = (byte)v;
}
@Override
public void writeShort(int v) throws IOException {
activePosition = write16(byteBuffer, byteMask, activePosition, v);
}
@Override
public void writeChar(int v) throws IOException {
activePosition = write16(byteBuffer, byteMask, activePosition, v);
}
@Override
public void writeInt(int v) throws IOException {
activePosition = write32(byteBuffer, byteMask, activePosition, v);
}
@Override
public void writeLong(long v) throws IOException {
activePosition = write64(byteBuffer, byteMask, activePosition, v);
}
@Override
public void writeFloat(float v) throws IOException {
activePosition = write32(byteBuffer, byteMask, activePosition, Float.floatToIntBits(v));
}
@Override
public void writeDouble(double v) throws IOException {
activePosition = write64(byteBuffer, byteMask, activePosition, Double.doubleToLongBits(v));
}
@Override
public void writeBytes(String s) throws IOException {
byte[] localBuf = byteBuffer;
int mask = byteMask;
int pos = activePosition;
int len = s.length();
for (int i = 0; i < len; i ++) {
localBuf[mask & pos++] = (byte) s.charAt(i);
}
activePosition = pos;
}
@Override
public void writeChars(String s) throws IOException {
byte[] localBuf = byteBuffer;
int mask = byteMask;
int pos = activePosition;
int len = s.length();
for (int i = 0; i < len; i ++) {
pos = write16(localBuf, mask, pos, (int) s.charAt(i));
}
activePosition = pos;
}
@Override
public void writeUTF(String s) throws IOException {
activePosition = encodeAsUTF8(s, s.length(), byteMask, byteBuffer, activePosition);
}
private int encodeAsUTF8(CharSequence s, int len, int mask, byte[] localBuf, int pos) {
int origPos = pos;
pos+=2;
int c = 0;
while (c < len) {
pos = Pipe.encodeSingleChar((int) s.charAt(c++), localBuf, mask, pos);
}
write16(localBuf,mask,origPos, (pos-origPos)-2); //writes bytes count up front
return pos;
}
///////////
//end of DataOutput methods
//////////
private static int write16(byte[] buf, int mask, int pos, int v) {
buf[mask & pos++] = (byte) v;
buf[mask & pos++] = (byte)(v >>> 8);
return pos;
}
private static int write32(byte[] buf, int mask, int pos, int v) {
buf[mask & pos++] = (byte) v;
buf[mask & pos++] = (byte)(v >>> 8);
buf[mask & pos++] = (byte)(v >>> 16);
buf[mask & pos++] = (byte)(v >>> 24);
return pos;
}
private static int write64(byte[] buf, int mask, int pos, long v) {
buf[mask & pos++] = (byte) v;
buf[mask & pos++] = (byte)(v >>> 8);
buf[mask & pos++] = (byte)(v >>> 16);
buf[mask & pos++] = (byte)(v >>> 24);
buf[mask & pos++] = (byte)(v >>> 32);
buf[mask & pos++] = (byte)(v >>> 40);
buf[mask & pos++] = (byte)(v >>> 48);
buf[mask & pos++] = (byte)(v >>> 56);
return pos;
}
public void writeUTF(CharSequence s) throws IOException {
activePosition = encodeAsUTF8(s, s.length(), byteMask, byteBuffer, activePosition);
}
public void writeASCII(CharSequence s) {
byte[] localBuf = byteBuffer;
int mask = byteMask;
int pos = activePosition;
int len = s.length();
for (int i = 0; i < len; i ++) {
localBuf[mask & pos++] = (byte)s.charAt(i);
}
activePosition = pos;
}
public void writeByteArray(byte[] bytes) throws IOException {
activePosition = writeByteArray(bytes, bytes.length, byteBuffer, byteMask, activePosition);
}
private int writeByteArray(byte[] bytes, int len, byte[] bufLocal, int mask, int pos) {
pos = write32(bufLocal, mask, pos, len);
for(int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy