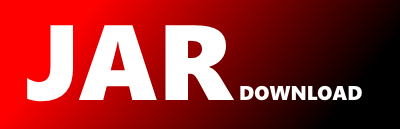
com.javanut.pronghorn.pipe.MessageSchema Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pronghorn-pipes Show documentation
Show all versions of pronghorn-pipes Show documentation
Ring buffer based queuing utility for applications that require high performance and/or a small
footprint. Well suited for embedded and stream based processing.
package com.javanut.pronghorn.pipe;
import java.lang.reflect.Field;
import java.lang.reflect.Modifier;
public abstract class MessageSchema> {
protected final FieldReferenceOffsetManager from; //will be null for schema-less
protected MessageSchema(FieldReferenceOffsetManager from) {
this.from = from;
}
public static final FieldReferenceOffsetManager from(MessageSchema schema) {
return schema.from;
}
public int getLocator(String messageName, String fieldName) {
return from.getLoc(messageName, fieldName);
}
public int getLocator(long messageId, long fieldId) {
return from.getLoc(messageId, fieldId);
}
public PipeConfig newPipeConfig(int minimumFragmentsOnRing, int maximumLenghOfVariableLengthFields) {
return new PipeConfig((T)this, minimumFragmentsOnRing, maximumLenghOfVariableLengthFields);
};
public PipeConfig newPipeConfig(int minimumFragmentsOnRing) {
return new PipeConfig((T)this, minimumFragmentsOnRing, 0);
};
public Pipe newPipe(int minimumFragmentsOnRing, int maximumLenghOfVariableLengthFields) {
return new Pipe((PipeConfig) newPipeConfig(minimumFragmentsOnRing, maximumLenghOfVariableLengthFields));
}
public Pipe newPipe(int minimumFragmentsOnRing) {
return new Pipe((PipeConfig) newPipeConfig(minimumFragmentsOnRing, 0));
}
//TODO: write a second one for assert only which confirms only 1 static instance field.
public static > S findInstance(Class clazz) {
Field[] declaredFields = clazz.getDeclaredFields();
for(int i=0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy