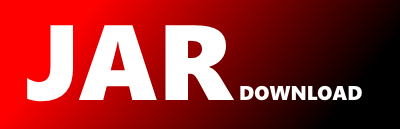
com.javanut.pronghorn.pipe.PipeMonitor Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pronghorn-pipes Show documentation
Show all versions of pronghorn-pipes Show documentation
Ring buffer based queuing utility for applications that require high performance and/or a small
footprint. Well suited for embedded and stream based processing.
package com.javanut.pronghorn.pipe;
public class PipeMonitor {
private static Pipe[] targetPipes = new Pipe[0];
public static synchronized > Pipe addMonitor(Pipe sourcePipe) {
//can only have 1 monitoring pipe, that pipe however could feed a replicator stage
if (isMonitored(sourcePipe.id)) {
throw new UnsupportedOperationException("This pipe is already configured to be monitored "+sourcePipe);
}
/////////////
if (targetPipes.length <= sourcePipe.id) {
Pipe[] newTargetPipes = new Pipe[sourcePipe.id+1];
System.arraycopy(targetPipes, 0,
newTargetPipes, 0, targetPipes.length);
targetPipes = newTargetPipes;
}
//this monitor pipe is the same size as the original
//NOTE we could make this bigger or smaller in the future as needed ...
Pipe pipe = new Pipe(sourcePipe.config());
targetPipes[sourcePipe.id] = pipe;
pipe.initBuffers();
return pipe;
}
public static > boolean monitor(Pipe sourcePipe,
long sourceSlabPos, int sourceBlobPos) {
if (isMonitored(sourcePipe.id)) {
Pipe localTarget = targetPipes[sourcePipe.id];
//will report errors if the logger does not keep this pipe clear
//but it also blocks to ensure nothing is ever lost
Pipe.presumeRoomForWrite(localTarget);
Pipe.copyFragment(sourcePipe, sourceSlabPos, sourceBlobPos, localTarget);
}
return true;
}
public static > boolean isMonitored(Pipe p) {
return isMonitored(p.id);
}
private static boolean isMonitored(int id) {
return id>=0 && id
© 2015 - 2025 Weber Informatics LLC | Privacy Policy