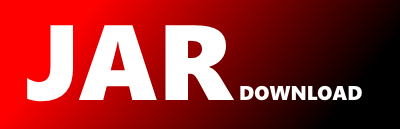
com.javanut.pronghorn.pipe.stream.StreamingReadVisitorMatcher Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pronghorn-pipes Show documentation
Show all versions of pronghorn-pipes Show documentation
Ring buffer based queuing utility for applications that require high performance and/or a small
footprint. Well suited for embedded and stream based processing.
package com.javanut.pronghorn.pipe.stream;
import static com.javanut.pronghorn.pipe.Pipe.blobMask;
import static com.javanut.pronghorn.pipe.Pipe.byteBackingArray;
import static com.javanut.pronghorn.pipe.Pipe.bytePosition;
import static com.javanut.pronghorn.pipe.Pipe.takeRingByteLen;
import static com.javanut.pronghorn.pipe.Pipe.takeRingByteMetaData;
import java.nio.Buffer;
import java.nio.ByteBuffer;
import com.javanut.pronghorn.pipe.FieldReferenceOffsetManager;
import com.javanut.pronghorn.pipe.Pipe;
public class StreamingReadVisitorMatcher extends StreamingReadVisitorAdapter {
private final Pipe expectedInput;
private final FieldReferenceOffsetManager expectedFrom;
private boolean needsClose = false;
private int activeCursor;
public StreamingReadVisitorMatcher(Pipe expectedInput) {
this.expectedInput = expectedInput;
this.expectedFrom = Pipe.from(expectedInput);
}
@Override
public boolean paused() {
return !Pipe.hasContentToRead(expectedInput, 1);
}
@Override
public void visitTemplateOpen(String name, long id) {
needsClose = true;
int msgIdx = Pipe.takeMsgIdx(expectedInput);
activeCursor = msgIdx;
if (id != expectedFrom.fieldIdScript[msgIdx]) {
throw new AssertionError("expected message id: "+expectedFrom.fieldIdScript[msgIdx]+" was given "+id);
}
}
@Override
public void visitTemplateClose(String name, long id) {
if (needsClose) {
needsClose = false;
Pipe.confirmLowLevelRead(expectedInput, Pipe.sizeOf(expectedInput, activeCursor));
Pipe.releaseReadLock(expectedInput);
}
}
@Override
public void visitFragmentOpen(String name, long id, int cursor) {
needsClose = true;
}
@Override
public void visitFragmentClose(String name, long id) {
if (needsClose) {
needsClose = false;
Pipe.confirmLowLevelRead(expectedInput, Pipe.sizeOf(expectedInput, activeCursor));
Pipe.releaseReadLock(expectedInput);
}
}
@Override
public void visitSequenceOpen(String name, long id, int length) {
int tempLen;
if ((tempLen=Pipe.takeInt(expectedInput))!=length) {
throw new AssertionError("expected length: "+Long.toHexString(tempLen)+" but got "+Long.toHexString(length));
}
needsClose = false;
Pipe.confirmLowLevelRead(expectedInput, Pipe.sizeOf(expectedInput, activeCursor));
Pipe.releaseReadLock(expectedInput);
}
@Override
public void visitSequenceClose(String name, long id) {
}
@Override
public void visitSignedInteger(String name, long id, int value) {
needsClose = true;
if (Pipe.takeInt(expectedInput) != value) {
throw new AssertionError();
}
}
@Override
public void visitUnsignedInteger(String name, long id, long value) {
needsClose = true;
int temp;
if ((temp = Pipe.takeInt(expectedInput)) != value) {
throw new AssertionError("expected integer "+temp+" but found "+value);
}
}
@Override
public void visitSignedLong(String name, long id, long value) {
needsClose = true;
if (Pipe.takeLong(expectedInput) != value) {
throw new AssertionError();
}
}
@Override
public void visitUnsignedLong(String name, long id, long value) {
needsClose = true;
long temp;
if ((temp=Pipe.takeLong(expectedInput)) != value) {
throw new AssertionError("expected long: "+Long.toHexString(temp)+" but got "+Long.toHexString(value));
}
}
@Override
public void visitDecimal(String name, long id, int exp, long mant) {
needsClose = true;
int tempExp;
if ((tempExp = Pipe.takeInt(expectedInput)) != exp) {
//TODO; AAAAAAA, decimal support is broken here must fix...
// throw new AssertionError("expected integer exponent "+tempExp+" but found "+exp);
}
long tempMant;
if ((tempMant=Pipe.takeLong(expectedInput)) != mant) {
// throw new AssertionError("expected long mantissa: "+Long.toHexString(tempMant)+" but got "+Long.toHexString(mant));
}
}
@Override
public void visitASCII(String name, long id, CharSequence value) {
needsClose = true;
int meta = Pipe.takeByteArrayMetaData((Pipe>) expectedInput);
int len = Pipe.takeByteArrayLength((Pipe>) expectedInput);
int pos = bytePosition(meta, expectedInput, len);
byte[] data = byteBackingArray(meta, expectedInput);
int mask = blobMask(expectedInput);//NOTE: the consumer must do their own ASCII conversion
//ascii so the bytes will match the chars
CharSequence seq = (CharSequence)value;
if (seq.length() != len) {
throw new AssertionError("expected ASCII length: "+Long.toHexString(len)+" but got "+Long.toHexString(seq.length()));
}
int i = 0;
while (i) expectedInput);
int len = Pipe.takeByteArrayLength((Pipe>) expectedInput);
int pos = bytePosition(meta, expectedInput, len);
byte[] data = byteBackingArray(meta, expectedInput);
int mask = blobMask(expectedInput);//NOTE: the consumer must do their own ASCII conversion
CharSequence seq = (CharSequence)value;
int seqPos = 0;
//we must check that seq equals the text encoded in data and if they do not match throw an AssertionError.
long charAndPos = ((long)pos)<<32;
long limit = ((long)pos+len)<<32;
while (charAndPos) expectedInput);
int len = Pipe.takeByteArrayLength((Pipe>) expectedInput);
int pos = bytePosition(meta, expectedInput, len);
byte[] data = byteBackingArray(meta, expectedInput);
int mask = blobMask(expectedInput);//NOTE: the consumer must do their own ASCII conversion
if (value.remaining() != len) {
throw new AssertionError("expected bytes length: "+len+" but got "+value.remaining());
}
int i = 0;
while (i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy