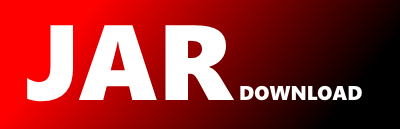
com.javanut.pronghorn.pipe.util.hash.IntHashTable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pronghorn-pipes Show documentation
Show all versions of pronghorn-pipes Show documentation
Ring buffer based queuing utility for applications that require high performance and/or a small
footprint. Well suited for embedded and stream based processing.
package com.javanut.pronghorn.pipe.util.hash;
import java.util.Arrays;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
/**
* Non-Thread safe simple fast hash for int to int mapping.
*
* No set is allowed unless no previous value is found.
* To change previous value replace must be called.
* Remove can not be supported.
* Key must not be zero.
*
* @author Nathan Tippy
*
*/
public class IntHashTable {
private static final Logger logger = LoggerFactory.getLogger(IntHashTable.class);
public static IntHashTable EMPTY = new IntHashTable(0);
private final int mask;
private final long[] data;
private int space;
private final int bits;
public static IntHashTable newTableExpectingCount(int count) {
return new IntHashTable((int) (1+Math.ceil(Math.log(count)/Math.log(2)) ));
}
public IntHashTable(int bits) {
this.bits = bits;
int size = 1<> 32);
}
private static long scanForItem(int key, int mask, int hash, long[] data2, long block) {
while ((block != 0) && ((int)block) != key) {
block = data2[++hash & mask];
}
return block;
}
public static boolean hasItem(IntHashTable ht, int key) {
int mask = ht.mask;
int hash = MurmurHash.hash32finalizer(key);
long block = ht.data[hash & mask];
while (((int)block) != key && block != 0) {
block = ht.data[++hash & mask];
}
return 0!=block;
}
public static boolean replaceItem(IntHashTable ht, int key, int newValue) {
int mask = ht.mask;
int hash = MurmurHash.hash32finalizer(key);
int temp = (int)ht.data[hash&mask];//just the lower int.
while (temp != key && temp != 0) {
temp = (int)ht.data[++hash & mask];
}
if (0 == temp) {
return false; //do not set item if it does not hold a previous value.
}
long block = newValue;
block = (block<<32) | (0xFFFFFFFF&key);
ht.data[hash&mask] = block;
return true;
}
public static void visit(IntHashTable ht, IntHashTableVisitor visitor) {
int j = ht.mask+1;
while (--j >= 0) {
long block = ht.data[j];
if (0!=block) {
int key = (int)block;
int value = (int)(block>>32);
visitor.visit(key,value);
}
}
}
public static IntHashTable doubleSize(IntHashTable ht) {
IntHashTable newHT = new IntHashTable(ht.bits+1);
int j = ht.mask+1;
while (--j >= 0) {
long block = ht.data[j];
if (0!=block) {
int key = (int)block;
int value = (int)(block>>32);
setItem(newHT, key, value);
}
}
assert(newHT.space>0);
return newHT;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy