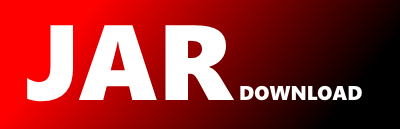
com.javanut.pronghorn.util.Blocker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pronghorn-pipes Show documentation
Show all versions of pronghorn-pipes Show documentation
Ring buffer based queuing utility for applications that require high performance and/or a small
footprint. Well suited for embedded and stream based processing.
package com.javanut.pronghorn.util;
public class Blocker {
private final long[] untilTimes;
private int itemCount;
private int blockedCount;
public enum BlockStatus {
None,
Blocked,
Released
}
public Blocker(int maxUniqueValues) {
untilTimes = new long[maxUniqueValues];
}
/**
* set release until time for a particular id.
* will return false if this id is already waiting on a particular time.
*
* @param id
* @param untilTime
*/
public boolean until(int id, long untilTime) {
assert(untilTime=0) {
long time = local[j];
if (0!=time && time=untilTimes.length)) ? false : 0!=untilTimes[item-1];
}
public long isBlockedUntil(int id) {
int item = id+1;
return (item<1) ? 0 : (0==untilTimes[item-1]? 0 : untilTimes[item-1]);
}
//TODO: urgent needs a unit test to cover
/**
* Returns true if any block will be released in the defined window.
*
*/
public boolean willReleaseInWindow(long limit) {
if (0==blockedCount) {
return false;
}
return willReleaseInWindow(limit, untilTimes);
}
private boolean willReleaseInWindow(long limit, long[] local) {
int i = itemCount; //no need to scan above this point;
while (--i>=0) {
long t = local[i];
if (0==t || t>=limit) {
} else {
return true;
}
}
return false;
}
public long durationToNextRelease(long currentTimeMillis, long defaultValue) {
if (0==blockedCount) {
return defaultValue;
}
int i = itemCount; //no need to scan above this point
long[] local = untilTimes;
long minValue = defaultValue;
while (--i>=0) {
long t = local[i];
if (t>=currentTimeMillis) {
long duration = t-currentTimeMillis;
minValue = Math.min(duration, minValue);
}
}
return minValue;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy