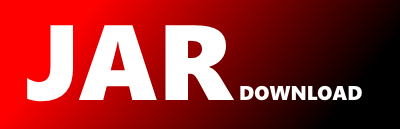
com.javanut.pronghorn.util.parse.JSONStreamVisitorCapture Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pronghorn-pipes Show documentation
Show all versions of pronghorn-pipes Show documentation
Ring buffer based queuing utility for applications that require high performance and/or a small
footprint. Well suited for embedded and stream based processing.
package com.javanut.pronghorn.util.parse;
import java.io.IOException;
import com.javanut.pronghorn.util.Appendables;
import com.javanut.pronghorn.util.ByteConsumer;
public class JSONStreamVisitorCapture implements JSONStreamVisitor {
final A target;
int depth = 0;
boolean objectStart = true;
boolean simpleArray = false;
final StringBuilder builder = new StringBuilder();
final ByteConsumer con = new ByteConsumer() {
@Override
public void consume(byte[] backing, int pos, int len, int mask) {
Appendables.appendUTF8(builder, backing, pos, len, mask);
}
@Override
public void consume(byte value) {
builder.append((char)value);
}
};
public JSONStreamVisitorCapture(A target) {
this.target = target;
}
@Override
public void nameSeparator() {
try {
objectStart = false;
target.append(':');
simpleArray = false;
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Override
public void endObject() {
try {
depth--;
target.append('}').append('\n');
objectStart = true;
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Override
public void beginObject() {
try {
depth++;
target.append('{').append('\n');
objectStart=true;
simpleArray = false;
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Override
public void beginArray() {
try {
target.append('[');
simpleArray = true;
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Override
public void endArray() {
try {
target.append(']');
simpleArray = false;
objectStart = true;
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Override
public void valueSeparator() {
try {
target.append(',');
if (!simpleArray) {
target.append('\n');
}
objectStart = true;
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Override
public void whiteSpace(byte b) {
}
@Override
public void literalTrue() {
try {
target.append("true");
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Override
public void literalNull() {
try {
target.append("null");
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Override
public void literalFalse() {
try {
target.append("false");
} catch (IOException e) {
throw new RuntimeException(e);
}
}
@Override
public void numberValue(long m, byte e) {
Appendables.appendDecimalValue(target, m, e);
}
@Override
public void stringBegin() {
try {
if (objectStart) {
for(int i = 0;i
© 2015 - 2025 Weber Informatics LLC | Privacy Policy