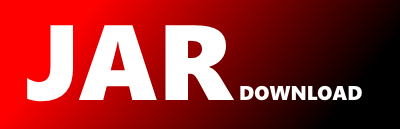
com.javanut.pronghorn.util.primitive.LoisOpBitMap Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pronghorn-pipes Show documentation
Show all versions of pronghorn-pipes Show documentation
Ring buffer based queuing utility for applications that require high performance and/or a small
footprint. Well suited for embedded and stream based processing.
package com.javanut.pronghorn.util.primitive;
import java.util.Arrays;
public class LoisOpBitMap extends LoisOperator {
private final int id;
//first int is jump to next
//second int top 3 is the id
//second int lower 29 are bits
//last int is the starting position
public LoisOpBitMap(int id) {
this.id = id;
}
private static final int firstValue(int idx, Lois lois) {
return lois.data[idx+lois.blockSize-1];
}
public static int valuesTracked(Lois lois) {
return ((lois.blockSize-2)*32)-3; //((16-2)*32)-3 -> 445
}
private final int lastValue(int idx, Lois lois) {
return firstValue(idx,lois)+valuesTracked(lois)-1;
}
@Override
public boolean isBefore(int idx, int value, Lois lois) {
return valuelastValue(idx,lois);
}
@Override
public boolean remove(int prev, int idx, int value, Lois lois) {
int bitIdx = value-firstValue(idx, lois);
int byteOffset = 0;
int bitOffset = 0;
if (bitIdx>29) {
byteOffset = idx+2+((bitIdx-29)>>5);
bitOffset = (bitIdx-29)&0x1F;
} else {
byteOffset = idx+1;
bitOffset = bitIdx;
}
int mask = 1< 4 ??
//}
int firstValue = firstValue(idx, lois);
int tracked = valuesTracked(lois);
//System.err.println("insert "+value+" first "+firstValue+" tracked "+tracked);
if (value >= firstValue+tracked) {
//System.err.println("new block");
//insert a new next block, after idx.
int newBlockId = LoisOpSimpleList.createNewBlock(idx, lois, value);
lois.data[idx] = newBlockId;//do not inline data array may be modified.
return true;
} else {
assert(!isAfter(idx, value, lois)) : "Must not be AFTER this block must be inside it";
if (isBefore(idx, value, lois)) {
//we need to copy this full block as is to a new location
//then rewrite this position as a simple pointing to new position.
int newHome = lois.newBlock();
System.arraycopy(lois.data, idx, lois.data, newHome, lois.blockSize);
assert(firstValue(idx, lois) == firstValue(newHome, lois));
LoisOpSimpleList.formatNewBlock(lois, value, idx, newHome);
return false;
} else {
int bitIdx = value - firstValue;
//System.err.println("bit idx "+bitIdx);
int byteOffset = 0;
int bitOffset = 0;
if (bitIdx >= 29) {
byteOffset = idx+2+((bitIdx-29)>>5);
bitOffset = (bitIdx-29)&0x1F;
//System.err.println("bit offset "+bitOffset);
} else {
//0-28
byteOffset = idx+1;
bitOffset = bitIdx;
}
int mask = 1<= 0) {
dat = lois.data[++z];
for(int x = 0; x < 32; x++) {
//System.err.println("#"+value);
if (0 != (dat & (1<=29) {
final int byteOffset = (bit-29)>>5;
final int bitOffset = (bit-29)&0x1F;
//System.err.println("a - set bit at "+(idx+2+byteOffset));
lois.data[idx+2+byteOffset] |= (1<=29) {
byteOffset = idx+2+((b-29)>>5);
bitOffset = (b-29)&0x1F;
} else {
byteOffset = idx+1;
bitOffset = b;
}
int mask = 1<
© 2015 - 2025 Weber Informatics LLC | Privacy Policy