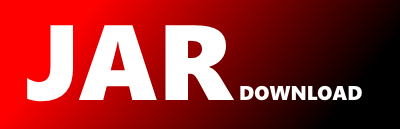
com.javanut.pronghorn.util.template.StringTemplateBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of pronghorn-pipes Show documentation
Show all versions of pronghorn-pipes Show documentation
Ring buffer based queuing utility for applications that require high performance and/or a small
footprint. Well suited for embedded and stream based processing.
package com.javanut.pronghorn.util.template;
import com.javanut.pronghorn.util.AppendableByteWriter;
import com.javanut.pronghorn.util.ByteWriter;
public class StringTemplateBuilder extends StringTemplateRenderer implements ByteWriter {
private StringTemplateScript[] script;
private int count;
public StringTemplateBuilder() {
this.script = new StringTemplateScript[8];
}
public StringTemplateBuilder add(String text) {
return addBytes(text.getBytes());
}
public StringTemplateBuilder add(final byte[] byteData) {
return add(byteData, 0, byteData.length);
}
public StringTemplateBuilder add(final byte[] byteData, int pos, int len) {
if (byteData != null && len > 0) {
final byte[] localData = new byte[len];
System.arraycopy(byteData, pos, localData, 0, len);
addBytes(localData);
}
return this;
}
public StringTemplateBuilder add(StringTemplateScript script) {
return append(script);
}
public StringTemplateBuilder add(final StringTemplateIterScript script) {
return append(
new StringTemplateScript() {
@Override
public void render(AppendableByteWriter writer, T source) {
for(int i = 0; (script.render(writer, source, i)); i++) {
}
}
});
}
public StringTemplateBuilder add(final StringTemplateScript[] branches, final StringTemplateBranching select) {
final StringTemplateScript[] localData = new StringTemplateScript[branches.length];
System.arraycopy(branches, 0, localData, 0, branches.length);
return append(
new StringTemplateScript() {
@Override
public void render(AppendableByteWriter writer, T source) {
int s = select.branch(source);
if (s != -1) {
assert (s < localData.length) : "String template builder selected invalid branch.";
localData[s].render(writer, source);
}
}
});
}
public StringTemplateRenderer finish() {
return this;
}
@Override
public void render(final AppendableByteWriter> writer, final T source) {
render(this,writer,source);
}
public static void render(StringTemplateBuilder builder, AppendableByteWriter> writer, T source) {
//assert(immutable) : "String template builder can only be rendered after lock.";
StringTemplateScript[] localScript = builder.script;
for(int i=0;i addBytes(final byte[] byteData) {
return append(
new StringTemplateScript() {
@Override
public void render(AppendableByteWriter writer, T source) {
writer.write(byteData);
}
});
}
private StringTemplateBuilder append(StringTemplateScript fetchData) {
//assert(!immutable) : "String template builder cannot be modified after lock.";
if (count==script.length) {
StringTemplateScript[] newScript = new StringTemplateScript[script.length*2];
System.arraycopy(script, 0, newScript, 0, script.length);
script = newScript;
}
script[count++] = fetchData;
return this;
}
@Override
public void write(byte[] encodedBlock) {
add(encodedBlock);
}
@Override
public void write(byte[] encodedBlock, int pos, int len) {
add(encodedBlock, pos, len);
}
@Override
public void writeByte(final int asciiChar) {
append(
new StringTemplateScript() {
@Override
public void render(AppendableByteWriter writer, T source) {
writer.writeByte(asciiChar);
}
});
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy