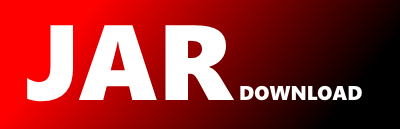
com.javaoffers.batis.modelhelper.util.HelperUtils Maven / Gradle / Ivy
The newest version!
package com.javaoffers.batis.modelhelper.util;
import com.javaoffers.batis.modelhelper.anno.BaseModel;
import com.javaoffers.batis.modelhelper.anno.BaseUnique;
import com.javaoffers.batis.modelhelper.core.ConvertRegisterSelectorDelegate;
import com.javaoffers.batis.modelhelper.exception.BaseException;
import com.javaoffers.batis.modelhelper.exception.ParseModelException;
import com.javaoffers.batis.modelhelper.utils.SoftCache;
import com.javaoffers.batis.modelhelper.utils.Utils;
import org.apache.commons.lang3.StringUtils;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import java.lang.reflect.Field;
import java.lang.reflect.ParameterizedType;
import java.lang.reflect.Type;
import java.util.*;
import java.util.stream.Collectors;
/**
* @Description: Tools
* @Auther: create by cmj on 2021/12/9 11:23
*/
public class HelperUtils {
static Logger logger = LoggerFactory.getLogger(HelperUtils.class);
static final String modelSeparation = "__";
private static final ConvertRegisterSelectorDelegate convert = ConvertRegisterSelectorDelegate.convert;
/**
* Convert underscore to camel case
*
* @param list_map
* @return
*/
@Deprecated
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy