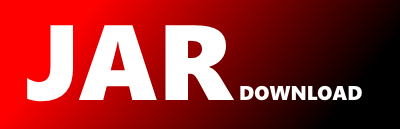
javaslang.option.None Maven / Gradle / Ivy
package javaslang.option;
import java.util.NoSuchElementException;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Predicate;
import java.util.function.Supplier;
/**
* None is a singleton representation of the undefined {@link javaslang.option.Option}. The instance
* is obtained by calling {@link #instance()}.
*
* @param The type of the optional value.
*/
public final class None implements Option {
private static final None> NONE = new None<>();
private None() {
}
/**
* Returns the singleton instance of None as {@code None} in the
* context of a type {@code }, e.g.
*
*
* final Option<Integer> o = None.instance(); // o is of type None<Integer>
*
*
* @param The type of the optional value.
* @return None
*/
public static None instance() {
@SuppressWarnings("unchecked")
final None none = (None) NONE;
return none;
}
@Override
public T get() {
throw new NoSuchElementException("No value present");
}
@Override
public T orElse(T other) {
return other;
}
@Override
public T orElseGet(Supplier extends T> other) {
return other.get();
}
@Override
public T orElseThrow(Supplier extends X> exceptionSupplier) throws X {
throw exceptionSupplier.get();
}
@Override
public boolean isPresent() {
return false;
}
@Override
public void ifPresent(Consumer super T> consumer) {
// nothing to do
}
@Override
public Option filter(Predicate super T> predicate) {
// semantically correct but structurally the same that return this;
return None.instance();
}
@Override
public Option map(Function super T, ? extends U> mapper) {
return None.instance();
}
@Override
public Option flatMap(Function super T, Option> mapper) {
return None.instance();
}
@Override
public void forEach(Consumer super T> action) {
// nothing to do
}
// super.equals and super.hashCode are fine because this is a singleton
@Override
public String toString() {
return "None";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy