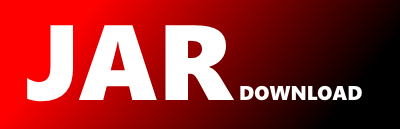
commonMain.extensions.EitherCombine.kt Maven / Gradle / Ivy
package com.javiersc.either.extensions
import com.javiersc.either.Either
import kotlin.jvm.JvmName
/** Combine an Either with another Either where both and the resulting Either have the same type */
public fun Either.combine(
either: Either,
left: (L, L) -> L,
right: (R, R) -> R,
): Either =
when (this) {
is Either.Left ->
when (either) {
is Either.Left -> Either.Left(left(this.left, either.left))
is Either.Right -> this
}
is Either.Right ->
when (either) {
is Either.Left -> either
is Either.Right -> Either.Right(right(this.right, either.right))
}
}
/** Combine an Either with another Either where all Either have different types */
@JvmName("combine2")
public fun Either.combine(
another: Either,
left: (L1, L2) -> L,
thisLeft: (L1) -> L,
anotherLeft: (L2) -> L,
right: (R1, R2) -> R,
): Either =
when (this) {
is Either.Left ->
when (another) {
is Either.Left -> Either.Left(left(this.left, another.left))
is Either.Right -> Either.Left(thisLeft(this.left))
}
is Either.Right ->
when (another) {
is Either.Left -> Either.Left(anotherLeft(another.left))
is Either.Right -> Either.Right(right(this.right, another.right))
}
}
/**
* Combine an Either with another Either where all Either have different right type and same left
* type
*/
@JvmName("combine3")
public fun Either.combine(
either: Either,
left: (L, L) -> L,
right: (R1, R2) -> R,
): Either =
when (this) {
is Either.Left ->
when (either) {
is Either.Left -> Either.Left(left(this.left, either.left))
is Either.Right -> this
}
is Either.Right ->
when (either) {
is Either.Left -> either
is Either.Right -> Either.Right(right(this.right, either.right))
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy