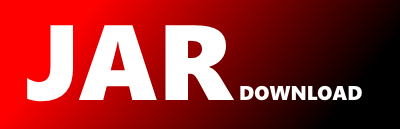
com.jayway.android.robotium.solo.Searcher Maven / Gradle / Ivy
package com.jayway.android.robotium.solo;
import java.util.ArrayList;
import java.util.Iterator;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import android.app.Instrumentation;
import android.widget.Button;
import android.widget.EditText;
import android.widget.TextView;
import android.widget.ToggleButton;
/**
* This class contains various search methods. Examples are: searchEditText(),
* searchText(), searchButton().
*
* @author Renas Reda, [email protected]
*
*/
class Searcher {
private final ViewFetcher soloView;
private final Scroller soloScroll;
private final Instrumentation inst;
private final int PAUS = 500;
/**
* Constructs this object.
*
* @param soloView the {@link ViewFetcher} instance.
* @param soloActivity the {@link ActivityUtils} instance.
* @param soloScroll the {@link Scroller} instance.
* @param inst the {@link Instrumentation} instance.
*/
public Searcher(ViewFetcher soloView, Scroller soloScroll, Instrumentation inst) {
this.soloView = soloView;
this.soloScroll = soloScroll;
this.inst = inst;
}
/**
* Searches for a text string in the edit texts located in the current
* activity.
*
* @param search the search string to be searched. Regular expressions are supported
* @return true if an edit text with the given text is found or false if it is not found
*
*/
public boolean searchEditText(String search) {
inst.waitForIdleSync();
RobotiumUtils.sleep(PAUS);
Pattern p = Pattern.compile(search);
Matcher matcher;
ArrayList editTextList = soloView.getCurrentEditTexts();
Iterator iterator = editTextList.iterator();
while (iterator.hasNext()) {
EditText editText = (EditText) iterator.next();
matcher = p.matcher(editText.getText().toString());
if (matcher.find()) {
return true;
}
}
if (soloScroll.scrollDownList())
return searchEditText(search);
else
return false;
}
/**
* Searches for a button with the given search string and returns true if at least one button
* is found with the expected text
*
* @param search the string to be searched. Regular expressions are supported
* @return true if a button with the given text is found and false if it is not found
*
*/
public boolean searchButton(String search) {
return searchButton(search, 0);
}
/**
* Searches for a toggle button with the given search string and returns true if at least one button
* is found with the expected text
*
* @param search the string to be searched. Regular expressions are supported
* @return true if a toggle button with the given text is found and false if it is not found
*
*/
public boolean searchToggleButton(String search) {
return searchToggleButton(search, 0);
}
/**
* Searches for a button with the given search string and returns true if the
* searched button is found a given number of times
*
* @param search the string to be searched. Regular expressions are supported
* @param matches the number of matches expected to be found. 0 matches means that one or more
* matches are expected to be found
* @return true if a button with the given text is found a given number of times and false
* if it is not found
*
*/
public boolean searchButton(String search, int matches) {
inst.waitForIdleSync();
RobotiumUtils.sleep(PAUS);
Pattern p = Pattern.compile(search);
Matcher matcher;
int countMatches=0;
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy