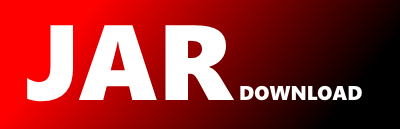
com.jayway.android.robotium.solo.ViewFetcher Maven / Gradle / Ivy
package com.jayway.android.robotium.solo;
import java.util.ArrayList;
import java.util.Iterator;
import android.app.Activity;
import android.app.Instrumentation;
import android.view.View;
import android.view.ViewGroup;
import android.widget.Button;
import android.widget.EditText;
import android.widget.GridView;
import android.widget.ImageView;
import android.widget.ListView;
import android.widget.Spinner;
import android.widget.TextView;
import android.widget.ToggleButton;
/**
* This class contains view methods. Examples are getViews(),
* getCurrentTextViews(), getCurrentImageViews().
*
* @author Renas Reda, [email protected]
*
*/
class ViewFetcher {
private final ArrayList viewList = new ArrayList();
private final ActivityUtils soloActivity;
private final Instrumentation inst;
/**
* Constructs this object.
*
* @param soloActivity the {@link Activity} instance.
* @param inst the {@link Instrumentation} instance.
*/
public ViewFetcher(ActivityUtils soloActivity, Instrumentation inst) {
this.soloActivity = soloActivity;
this.inst = inst;
}
/**
* Method used to get the absolute top view in an activity.
*
* @param view the view whose top parent is requested
* @return the top parent view
*
*/
public View getTopParent(View view) {
if (view.getParent() != null
&& !view.getParent().getClass().getName().equals(
"android.view.ViewRoot")) {
return getTopParent((View) view.getParent());
} else {
return view;
}
}
/**
* This method returns an ArrayList of all the views located in the current activity.
*
* @return ArrayList with the views found in the current activity
*
*/
public ArrayList getViews() {
Activity activity = soloActivity.getCurrentActivity();
inst.waitForIdleSync();
try {
View decorView = activity.getWindow().getDecorView();
viewList.clear();
getViews(getTopParent(decorView));
return viewList;
} catch (Throwable e) {
e.printStackTrace();
}
return null;
}
/**
* Private method which adds all the views located in the currently active
* activity to an ArrayList viewList.
*
* @param view the view who's children should be added to viewList
*
*/
private void getViews(View view) {
viewList.add(view);
if (view instanceof ViewGroup) {
ViewGroup vg = (ViewGroup) view;
for (int i = 0; i < vg.getChildCount(); i++) {
getViews(vg.getChildAt(i));
}
}
}
/**
* This method returns an ArrayList of the images contained in the current
* activity.
*
* @return ArrayList of the images contained in the current activity
*
*/
public ArrayList getCurrentImageViews() {
ArrayList viewList = getViews();
ArrayList imageViewList = new ArrayList();
Iterator iterator = viewList.iterator();
while (iterator.hasNext()) {
View view = iterator.next();
if (view instanceof android.widget.ImageView) {
imageViewList.add((ImageView) view);
}
}
return imageViewList;
}
/**
* This method returns an EditText with a certain index.
*
* @return the EditText with a specified index
*
*/
public EditText getEditText(int index) {
ArrayList editTextList = getCurrentEditTexts();
return editTextList.get(index);
}
/**
* This method returns a button with a certain index.
*
* @param index the index of the button
* @return the button with the specific index
*
*/
public Button getButton(int index) {
ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy