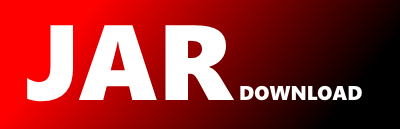
com.jayway.android.robotium.solo.Solo Maven / Gradle / Ivy
package com.jayway.android.robotium.solo;
import java.util.ArrayList;
import junit.framework.Assert;
import android.app.Activity;
import android.app.Instrumentation;
import android.content.pm.ActivityInfo;
import android.view.KeyEvent;
import android.view.View;
import android.widget.Button;
import android.widget.CheckBox;
import android.widget.CheckedTextView;
import android.widget.CompoundButton;
import android.widget.DatePicker;
import android.widget.EditText;
import android.widget.GridView;
import android.widget.ImageButton;
import android.widget.ImageView;
import android.widget.ProgressBar;
import android.widget.RadioButton;
import android.widget.ScrollView;
import android.widget.Spinner;
import android.widget.TextView;
import android.widget.ListView;
import android.widget.TimePicker;
import android.widget.ToggleButton;
/**
* This class contains all the methods that the sub-classes have. It supports test
* cases that span over multiple activities.
*
* Robotium has full support for Activities, Dialogs, Toasts, Menus and Context Menus.
*
* When writing tests there is no need to plan for or expect new activities in the test case.
* All is handled automatically by Robotium-Solo. Robotium-Solo can be used in conjunction with
* ActivityInstrumentationTestCase2. The test cases are written from a user
* perspective were technical details are not needed.
*
*
* Example of usage (test case spanning over multiple activities):
*
*
*
* public void setUp() throws Exception {
* solo = new Solo(getInstrumentation(), getActivity());
* }
*
* public void testTextShows() throws Exception {
*
* solo.clickOnText("Categories");
* solo.clickOnText("Other");
* solo.clickOnButton("Edit");
* solo.searchText("Edit Window");
* solo.clickOnButton("Commit");
* assertTrue(solo.searchText("Changes have been made successfully"));
* }
*
*
*
*
* @author Renas Reda, [email protected]
*
*/
public class Solo {
private final Asserter asserter;
private final ViewFetcher viewFetcher;
private final Checker checker;
private final Clicker clicker;
private final Presser presser;
private final Searcher searcher;
private final ActivityUtils activitiyUtils;
private final DialogUtils dialogUtils;
private final TextEnterer textEnterer;
private final Scroller scroller;
private final RobotiumUtils robotiumUtils;
private final Sleeper sleeper;
private final Waiter waiter;
private final Setter setter;
private final Instrumentation inst;
public final static int LANDSCAPE = ActivityInfo.SCREEN_ORIENTATION_LANDSCAPE; // 0
public final static int PORTRAIT = ActivityInfo.SCREEN_ORIENTATION_PORTRAIT; // 1
public final static int RIGHT = KeyEvent.KEYCODE_DPAD_RIGHT;
public final static int LEFT = KeyEvent.KEYCODE_DPAD_LEFT;
public final static int UP = KeyEvent.KEYCODE_DPAD_UP;
public final static int DOWN = KeyEvent.KEYCODE_DPAD_DOWN;
public final static int ENTER = KeyEvent.KEYCODE_ENTER;
public final static int MENU = KeyEvent.KEYCODE_MENU;
public final static int DELETE = KeyEvent.KEYCODE_DEL;
/**
* Constructor that takes in the instrumentation and the start activity.
*
* @param inst the {@link Instrumentation} instance.
* @param activity {@link Activity} the start activity
*
*/
public Solo(Instrumentation inst, Activity activity) {
this.inst = inst;
this.sleeper = new Sleeper();
this.activitiyUtils = new ActivityUtils(inst, activity, sleeper);
this.setter = new Setter(activitiyUtils);
this.viewFetcher = new ViewFetcher(inst, activitiyUtils, sleeper);
this.asserter = new Asserter(activitiyUtils, sleeper);
this.dialogUtils = new DialogUtils(viewFetcher, sleeper);
this.scroller = new Scroller(inst, activitiyUtils, viewFetcher, sleeper);
this.searcher = new Searcher(viewFetcher, scroller, inst, sleeper);
this.waiter = new Waiter(viewFetcher, searcher,scroller, sleeper);
this.checker = new Checker(viewFetcher, waiter);
this.robotiumUtils = new RobotiumUtils(inst, sleeper);
this.clicker = new Clicker(viewFetcher, scroller,robotiumUtils, inst, sleeper, waiter);
this.presser = new Presser(viewFetcher, clicker, inst, sleeper);
this.textEnterer = new TextEnterer(activitiyUtils, waiter);
}
/**
* Returns an {@code ArrayList} of the {@code View} objects located in the current
* {@code Activity}.
*
* @return an {@code ArrayList} of the {@code View} objects located in the current {@code Activity}
*
*/
public ArrayList getViews() {
try {
return viewFetcher.getViews(null);
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
/**
* Returns an {@code ArrayList} of the {@code View} objects contained in the parent view
*
* @param parent the parent view from which to return the views
* @return an {@code ArrayList} of the {@code View} objects contained in the given {@code View}
*
*/
public ArrayList getViews(View parent) {
try {
return viewFetcher.getViews(parent);
} catch (Exception e) {
e.printStackTrace();
return null;
}
}
/**
* Returns the absolute top parent {@code View} in for a given {@code View}.
*
* @param view the {@code View} whose top parent is requested
* @return the top parent {@code View}
*
*/
public View getTopParent(View view) {
View topParent = viewFetcher.getTopParent(view);
return topParent;
}
/**
* Clears the value of an {@link EditText}.
*
* @param index the index of the {@code EditText} that should be cleared. 0 if only one is available
*
*/
public void clearEditText(int index) {
waiter.waitForView(EditText.class, index);
ArrayList visibleEditTexts = RobotiumUtils.removeInvisibleViews(getCurrentEditTexts());
if(index > visibleEditTexts.size()-1)
Assert.assertTrue("EditText with index " + index + " is not available!", false);
textEnterer.setEditText(visibleEditTexts.get(index), "");
}
/**
* Clears the value of an {@link EditText}.
*
* @param editText the {@code EditText} that should be cleared
*
*/
public void clearEditText(EditText editText) {
waiter.waitForView(EditText.class, 0);
textEnterer.setEditText(editText, "");
}
/**
* Waits for a text to be shown. Default timeout is 20 seconds.
*
* @param text the text that is expected to be shown
* @return {@code true} if text is shown and {@code false} if it is not shown before the timeout
*
*/
public boolean waitForText(String text) {
return waiter.waitForText(text);
}
/**
* Waits for a text to be shown.
*
* @param text the text that needs to be shown
* @param minimumNumberOfMatches the minimum number of matches that are expected to be shown. {@code 0} means any number of matches
* @param timeout the the amount of time in milliseconds to wait
* @return {@code true} if text is shown and {@code false} if it is not shown before the timeout
*
*/
public boolean waitForText(String text, int minimumNumberOfMatches, long timeout) {
return waiter.waitForText(text, minimumNumberOfMatches, timeout);
}
/**
* Waits for a text to be shown.
*
* @param text the text that needs to be shown
* @param minimumNumberOfMatches the minimum number of matches that are expected to be shown. {@code 0} means any number of matches
* @param timeout the the amount of time in milliseconds to wait
* @param scroll {@code true} if scrolling should be performed
* @return {@code true} if text is shown and {@code false} if it is not shown before the timeout
*
*/
public boolean waitForText(String text, int minimumNumberOfMatches, long timeout, boolean scroll) {
return waiter.waitForText(text, minimumNumberOfMatches, timeout, scroll);
}
/**
* Waits for a view to be shown.
*
* @param viewClass the {@code View} class to wait for
* @param minimumNumberOfMatches the minimum number of matches that are expected to be shown. {@code 0} means any number of matches
* @param timeout the amount of time in milliseconds to wait
* @return {@code true} if view is shown and {@code false} if it is not shown before the timeout
*/
public boolean waitForView(final Class viewClass, final int minimumNumberOfMatches, final int timeout){
int index = minimumNumberOfMatches-1;
if(index < 1)
index = 0;
return waiter.waitForView(viewClass, index, timeout, true);
}
/**
* Waits for a view to be shown.
*
* @param viewClass the {@code View} class to wait for
* @param minimumNumberOfMatches the minimum number of matches that are expected to be shown. {@code 0} means any number of matches
* @param timeout the amount of time in milliseconds to wait
* @param scroll {@code true} if scrolling should be performed
* @return {@code true} if view is shown and {@code false} if it is not shown before the timeout
*/
public boolean waitForView(final Class viewClass, final int minimumNumberOfMatches, final int timeout,final boolean scroll){
int index = minimumNumberOfMatches-1;
if(index < 1)
index = 0;
return waiter.waitForView(viewClass, index, timeout, scroll);
}
/**
* Searches for a text string in the {@link EditText} objects located in the current
* {@code Activity}. Will automatically scroll when needed.
*
* @param text the text to search for
* @return {@code true} if an {@code EditText} with the given text is found or {@code false} if it is not found
*
*/
public boolean searchEditText(String text) {
boolean found = searcher.searchWithTimeoutFor(EditText.class, text, 1, true, false);
return found;
}
/**
* Searches for a {@link Button} with the given text string and returns true if at least one {@code Button}
* is found. Will automatically scroll when needed.
*
* @param text the text to search for. The parameter will be interpreted as a regular expression
* @return {@code true} if a {@code Button} with the given text is found and {@code false} if it is not found
*
*/
public boolean searchButton(String text) {
boolean found = searcher.searchWithTimeoutFor(Button.class, text, 0, true, false);
return found;
}
/**
* Searches for a {@link Button} with the given text string and returns true if at least one {@code Button}
* is found. Will automatically scroll when needed.
*
* @param text the text to search for. The parameter will be interpreted as a regular expression
* @param onlyVisible {@code true} if only {@code Button} visible on the screen should be searched
* @return {@code true} if a {@code Button} with the given text is found and {@code false} if it is not found
*
*/
public boolean searchButton(String text, boolean onlyVisible) {
boolean found = searcher.searchWithTimeoutFor(Button.class, text, 0, true, onlyVisible);
return found;
}
/**
* Searches for a {@link ToggleButton} with the given text string and returns {@code true} if at least one {@code ToggleButton}
* is found. Will automatically scroll when needed.
*
* @param text the text to search for. The parameter will be interpreted as a regular expression
* @return {@code true} if a {@code ToggleButton} with the given text is found and {@code false} if it is not found
*
*/
public boolean searchToggleButton(String text) {
boolean found = searcher.searchWithTimeoutFor(ToggleButton.class, text, 0, true, false);
return found;
}
/**
* Searches for a {@link Button} with the given text string and returns {@code true} if the
* searched {@code Button} is found a given number of times. Will automatically scroll when needed.
*
* @param text the text to search for. The parameter will be interpreted as a regular expression
* @param minimumNumberOfMatches the minimum number of matches expected to be found. {@code 0} matches means that one or more
* matches are expected to be found
* @return {@code true} if a {@code Button} with the given text is found a given number of times and {@code false}
* if it is not found
*
*/
public boolean searchButton(String text, int minimumNumberOfMatches) {
boolean found = searcher.searchWithTimeoutFor(Button.class, text, minimumNumberOfMatches, true, false);
return found;
}
/**
* Searches for a {@link Button} with the given text string and returns {@code true} if the
* searched {@code Button} is found a given number of times. Will automatically scroll when needed.
*
* @param text the text to search for. The parameter will be interpreted as a regular expression
* @param minimumNumberOfMatches the minimum number of matches expected to be found. {@code 0} matches means that one or more
* matches are expected to be found
* @param onlyVisible {@code true} if only {@code Button} visible on the screen should be searched
* @return {@code true} if a {@code Button} with the given text is found a given number of times and {@code false}
* if it is not found
*
*/
public boolean searchButton(String text, int minimumNumberOfMatches, boolean onlyVisible) {
boolean found = searcher.searchWithTimeoutFor(Button.class, text, minimumNumberOfMatches, true, onlyVisible);
return found;
}
/**
* Searches for a {@link ToggleButton} with the given text string and returns {@code true} if the
* searched {@code ToggleButton} is found a given number of times. Will automatically scroll when needed.
*
* @param text the text to search for. The parameter will be interpreted as a regular expression
* @param minimumNumberOfMatches the minimum number of matches expected to be found. {@code 0} matches means that one or more
* matches are expected to be found
* @return {@code true} if a {@code ToggleButton} with the given text is found a given number of times and {@code false}
* if it is not found
*
*/
public boolean searchToggleButton(String text, int minimumNumberOfMatches) {
boolean found = searcher.searchWithTimeoutFor(ToggleButton.class, text, minimumNumberOfMatches, true, false);
return found;
}
/**
* Searches for a text string and returns {@code true} if at least one item
* is found with the expected text. Will automatically scroll when needed.
*
* @param text the text to search for. The parameter will be interpreted as a regular expression
* @return {@code true} if the search string is found and {@code false} if it is not found
*
*/
public boolean searchText(String text) {
boolean found = searcher.searchWithTimeoutFor(TextView.class, text, 0, true, false);
return found;
}
/**
* Searches for a text string and returns {@code true} if at least one item
* is found with the expected text. Will automatically scroll when needed.
*
* @param text the text to search for. The parameter will be interpreted as a regular expression
* @param onlyVisible {@code true} if only texts visible on the screen should be searched
* @return {@code true} if the search string is found and {@code false} if it is not found
*
*/
public boolean searchText(String text, boolean onlyVisible) {
boolean found = searcher.searchWithTimeoutFor(TextView.class, text, 0, true, onlyVisible);
return found;
}
/**
* Searches for a text string and returns {@code true} if the searched text is found a given
* number of times. Will automatically scroll when needed.
*
* @param text the text to search for. The parameter will be interpreted as a regular expression
* @param minimumNumberOfMatches the minimum number of matches expected to be found. {@code 0} matches means that one or more
* matches are expected to be found
* @return {@code true} if text string is found a given number of times and {@code false} if the text string
* is not found
*
*/
public boolean searchText(String text, int minimumNumberOfMatches) {
boolean found = searcher.searchWithTimeoutFor(TextView.class, text, minimumNumberOfMatches, true, false);
return found;
}
/**
* Searches for a text string and returns {@code true} if the searched text is found a given
* number of times.
*
* @param text the text to search for. The parameter will be interpreted as a regular expression.
* @param minimumNumberOfMatches the minimum number of matches expected to be found. {@code 0} matches means that one or more
* matches are expected to be found
* @param scroll {@code true} if scrolling should be performed
* @return {@code true} if text string is found a given number of times and {@code false} if the text string
* is not found
*
*/
public boolean searchText(String text, int minimumNumberOfMatches, boolean scroll) {
return searcher.searchWithTimeoutFor(TextView.class, text, minimumNumberOfMatches, scroll, false);
}
/**
* Searches for a text string and returns {@code true} if the searched text is found a given
* number of times.
*
* @param text the text to search for. The parameter will be interpreted as a regular expression.
* @param minimumNumberOfMatches the minimum number of matches expected to be found. {@code 0} matches means that one or more
* matches are expected to be found
* @param scroll {@code true} if scrolling should be performed
* @param onlyVisible {@code true} if only texts visible on the screen should be searched
* @return {@code true} if text string is found a given number of times and {@code false} if the text string
* is not found
*
*/
public boolean searchText(String text, int minimumNumberOfMatches, boolean scroll, boolean onlyVisible) {
return searcher.searchWithTimeoutFor(TextView.class, text, minimumNumberOfMatches, scroll, onlyVisible);
}
/**
* Sets the Orientation (Landscape/Portrait) for the current activity.
*
* @param orientation the orientation to be set. Solo.
{@link #LANDSCAPE} for landscape or
* Solo.
{@link #PORTRAIT} for portrait.
*
*/
public void setActivityOrientation(int orientation)
{
activitiyUtils.setActivityOrientation(orientation);
}
/**
* Returns an {@code ArrayList} of all the opened/active activities.
*
* @return an {@code ArrayList} of all the opened/active activities
*
*/
public ArrayList getAllOpenedActivities()
{
return activitiyUtils.getAllOpenedActivities();
}
/**
* Returns the current {@code Activity}.
*
* @return the current {@code Activity}
*
*/
public Activity getCurrentActivity() {
Activity activity = activitiyUtils.getCurrentActivity();
return activity;
}
/**
* Asserts that the expected {@link Activity} is the currently active one.
*
* @param message the message that should be displayed if the assert fails
* @param name the name of the {@code Activity} that is expected to be active e.g. {@code "MyActivity"}
*
*/
public void assertCurrentActivity(String message, String name)
{
asserter.assertCurrentActivity(message, name);
}
/**
* Asserts that the expected {@link Activity} is the currently active one.
*
* @param message the message that should be displayed if the assert fails
* @param expectedClass the {@code Class} object that is expected to be active e.g. {@code MyActivity.class}
*
*/
@SuppressWarnings("unchecked")
public void assertCurrentActivity(String message, Class expectedClass)
{
asserter.assertCurrentActivity(message, expectedClass);
}
/**
* Asserts that the expected {@link Activity} is the currently active one, with the possibility to
* verify that the expected {@code Activity} is a new instance of the {@code Activity}.
*
* @param message the message that should be displayed if the assert fails
* @param name the name of the activity that is expected to be active e.g. {@code "MyActivity"}
* @param isNewInstance {@code true} if the expected {@code Activity} is a new instance of the {@code Activity}
*
*/
public void assertCurrentActivity(String message, String name, boolean isNewInstance)
{
asserter.assertCurrentActivity(message, name, isNewInstance);
}
/**
* Asserts that the expected {@link Activity} is the currently active one, with the possibility to
* verify that the expected {@code Activity} is a new instance of the {@code Activity}.
*
* @param message the message that should be displayed if the assert fails
* @param expectedClass the {@code Class} object that is expected to be active e.g. {@code MyActivity.class}
* @param isNewInstance {@code true} if the expected {@code Activity} is a new instance of the {@code Activity}
*
*/
@SuppressWarnings("unchecked")
public void assertCurrentActivity(String message, Class expectedClass,
boolean isNewInstance) {
asserter.assertCurrentActivity(message, expectedClass, isNewInstance);
}
/**
* Asserts that the available memory in the system is not low.
*
*/
public void assertMemoryNotLow()
{
asserter.assertMemoryNotLow();
}
/**
* Waits for a {@link android.app.Dialog} to close.
*
* @param timeout the amount of time in milliseconds to wait
* @return {@code true} if the {@code Dialog} is closed before the timeout and {@code false} if it is not closed
*
*/
public boolean waitForDialogToClose(long timeout) {
return dialogUtils.waitForDialogToClose(timeout);
}
/**
* Simulates pressing the hardware back key.
*
*/
public void goBack()
{
robotiumUtils.goBack();
}
/**
* Clicks on a given coordinate on the screen.
*
* @param x the x coordinate
* @param y the y coordinate
*
*/
public void clickOnScreen(float x, float y) {
sleeper.sleep();
inst.waitForIdleSync();
clicker.clickOnScreen(x, y);
}
/**
* Long clicks a given coordinate on the screen.
*
* @param x the x coordinate
* @param y the y coordinate
*
*/
public void clickLongOnScreen(float x, float y) {
clicker.clickLongOnScreen(x, y, 0);
}
/**
* Long clicks a given coordinate on the screen for a given amount of time.
*
* @param x the x coordinate
* @param y the y coordinate
* @param time the amount of time to long click
*
*/
public void clickLongOnScreen(float x, float y, int time) {
clicker.clickLongOnScreen(x, y, time);
}
/**
* Clicks on a {@link Button} with a given text. Will automatically scroll when needed.
*
* @param name the name of the {@code Button} presented to the user. The parameter will be interpreted as a regular expression
*
*/
public void clickOnButton(String name) {
clicker.clickOn(Button.class, name);
}
/**
* Clicks on an {@link ImageButton} with a given index.
*
* @param index the index of the {@code ImageButton} to be clicked. 0 if only one is available
*
*/
public void clickOnImageButton(int index) {
clicker.clickOn(ImageButton.class, index);
}
/**
* Clicks on a {@link ToggleButton} with a given text.
*
* @param name the name of the {@code ToggleButton} presented to the user. The parameter will be interpreted as a regular expression
*
*/
public void clickOnToggleButton(String name) {
clicker.clickOn(ToggleButton.class, name);
}
/**
* Clicks on a menu item with a given text.
* @param text the menu text that should be clicked on. The parameter will be interpreted as a regular expression
*
*/
public void clickOnMenuItem(String text)
{
clicker.clickOnMenuItem(text);
}
/**
* Clicks on a menu item with a given text.
*
* @param text the menu text that should be clicked on. The parameter will be interpreted as a regular expression
* @param subMenu true if the menu item could be located in a sub menu
*
*/
public void clickOnMenuItem(String text, boolean subMenu)
{
clicker.clickOnMenuItem(text, subMenu);
}
/**
* Presses a {@link android.view.MenuItem} with a given index. Index {@code 0} is the first item in the
* first row, Index {@code 3} is the first item in the second row and
* index {@code 5} is the first item in the third row.
*
* @param index the index of the menu item to be pressed
*
*/
public void pressMenuItem(int index) {
presser.pressMenuItem(index);
}
/**
* Presses on a {@link Spinner} (drop-down menu) item.
*
* @param spinnerIndex the index of the {@code Spinner} menu to be used
* @param itemIndex the index of the {@code Spinner} item to be pressed relative to the currently selected item
* A Negative number moves up on the {@code Spinner}, positive moves down
*
*/
public void pressSpinnerItem(int spinnerIndex, int itemIndex)
{
presser.pressSpinnerItem(spinnerIndex, itemIndex);
}
/**
* Clicks on a given {@link View}.
*
* @param view the {@code View} that should be clicked
*
*/
public void clickOnView(View view) {
waiter.waitForClickableItems();
clicker.clickOnScreen(view);
}
/**
* Long clicks on a given {@link View}.
*
* @param view the view that should be long clicked
*
*/
public void clickLongOnView(View view) {
waiter.waitForClickableItems();
clicker.clickOnScreen(view, true, 0);
}
/**
* Long clicks on a given {@link View} for a given amount of time.
*
* @param view the view that should be long clicked
* @param time the amount of time to long click
*
*/
public void clickLongOnView(View view, int time) {
waiter.waitForClickableItems();
clicker.clickOnScreen(view, true, time);
}
/**
* Clicks on a {@link View} displaying a given
* text. Will automatically scroll when needed.
*
* @param text the text that should be clicked on. The parameter will be interpreted as a regular expression
*
*/
public void clickOnText(String text) {
clicker.clickOnText(text, false, 1, true, 0);
}
/**
* Clicks on a {@link View} displaying a given text. Will automatically scroll when needed.
*
* @param text the text that should be clicked on. The parameter will be interpreted as a regular expression
* @param match the match that should be clicked on
*
*/
public void clickOnText(String text, int match) {
clicker.clickOnText(text, false, match, true, 0);
}
/**
* Clicks on a {@link View} displaying a given text.
*
* @param text the text that should be clicked on. The parameter will be interpreted as a regular expression
* @param match the match that should be clicked on
* @param scroll true if scrolling should be performed
*
*/
public void clickOnText(String text, int match, boolean scroll) {
clicker.clickOnText(text, false, match, scroll, 0);
}
/**
* Long clicks on a given {@link View}. Will automatically scroll when needed. {@link #clickOnText(String)} can then be
* used to click on the context menu items that appear after the long click.
*
* @param text the text that should be clicked on. The parameter will be interpreted as a regular expression
*
*/
public void clickLongOnText(String text)
{
clicker.clickOnText(text, true, 1, true, 0);
}
/**
* Long clicks on a given {@link View}. Will automatically scroll when needed. {@link #clickOnText(String)} can then be
* used to click on the context menu items that appear after the long click.
*
* @param text the text that should be clicked on. The parameter will be interpreted as a regular expression
* @param match the match that should be clicked on
*
*/
public void clickLongOnText(String text, int match)
{
clicker.clickOnText(text, true, match, true, 0);
}
/**
* Long clicks on a given {@link View}. {@link #clickOnText(String)} can then be
* used to click on the context menu items that appear after the long click.
*
* @param text the text that should be clicked on. The parameter will be interpreted as a regular expression
* @param match the match that should be clicked on
* @param scroll true if scrolling should be performed
*
*/
public void clickLongOnText(String text, int match, boolean scroll)
{
clicker.clickOnText(text, true, match, scroll, 0);
}
/**
* Long clicks on a given {@link View}. {@link #clickOnText(String)} can then be
* used to click on the context menu items that appear after the long click.
*
* @param text the text that should be clicked on. The parameter will be interpreted as a regular expression
* @param match the match that should be clicked on
* @param time the amount of time to long click
*/
public void clickLongOnText(String text, int match, int time)
{
clicker.clickOnText(text, true, match, true, time);
}
/**
* Long clicks on a given {@link View} and then selects
* an item from the context menu that appears. Will automatically scroll when needed.
*
* @param text the text to be clicked on. The parameter will be interpreted as a regular expression
* @param index the index of the menu item to be pressed. {@code 0} if only one is available
*
*/
public void clickLongOnTextAndPress(String text, int index) {
clicker.clickLongOnTextAndPress(text, index);
}
/**
* Clicks on a {@link Button} with a given index.
*
* @param index the index number of the {@code Button}. {@code 0} if only one is available
*
*/
public void clickOnButton(int index) {
clicker.clickOn(Button.class, index);
}
/**
* Clicks on a {@link RadioButton} with a given index.
*
* @param index the index of the {@code RadioButton} to be clicked. {@code 0} if only one is available
*
*/
public void clickOnRadioButton(int index) {
clicker.clickOn(RadioButton.class, index);
}
/**
* Clicks on a {@link CheckBox} with a given index.
*
* @param index the index of the {@code CheckBox} to be clicked. {@code 0} if only one is available
*
*/
public void clickOnCheckBox(int index) {
clicker.clickOn(CheckBox.class, index);
}
/**
* Clicks on an {@link EditText} with a given index.
*
* @param index the index of the {@code EditText} to be clicked. {@code 0} if only one is available
*
*/
public void clickOnEditText(int index) {
clicker.clickOn(EditText.class, index);
}
/**
* Clicks on a given list line and returns an {@code ArrayList} of the {@link TextView} objects that
* the list line is showing. Will use the first list it finds.
*
* @param line the line that should be clicked
* @return an {@code ArrayList} of the {@code TextView} objects located in the list line
*
*/
public ArrayList clickInList(int line) {
return clicker.clickInList(line);
}
/**
* Clicks on a given list line on a specified list and
* returns an {@code ArrayList} of the {@link TextView}s that the list line is showing.
*
* @param line the line that should be clicked
* @param listIndex the index of the list. 1 if two lists are available
* @return an {@code ArrayList} of the {@code TextView} objects located in the list line
*
*/
public ArrayList clickInList(int line, int listIndex) {
return clicker.clickInList(line, listIndex);
}
/**
* Simulate touching a given location and dragging it to a new location.
*
* This method was copied from {@code TouchUtils.java} in the Android Open Source Project, and modified here.
*
* @param fromX X coordinate of the initial touch, in screen coordinates
* @param toX X coordinate of the drag destination, in screen coordinates
* @param fromY X coordinate of the initial touch, in screen coordinates
* @param toY Y coordinate of the drag destination, in screen coordinates
* @param stepCount How many move steps to include in the drag
*
*/
public void drag(float fromX, float toX, float fromY, float toY,
int stepCount) {
scroller.drag(fromX, toX, fromY, toY, stepCount);
}
/**
* Scrolls down the screen.
*
* @return {@code true} if more scrolling can be done and {@code false} if it is at the end of
* the screen
*
*/
public boolean scrollDown() {
waiter.waitForViews(ListView.class, ScrollView.class);
return scroller.scroll(Scroller.Direction.DOWN);
}
/**
* Scrolls up the screen.
*
* @return {@code true} if more scrolling can be done and {@code false} if it is at the top of
* the screen
*
*/
public boolean scrollUp(){
waiter.waitForViews(ListView.class, ScrollView.class);
return scroller.scroll(Scroller.Direction.UP);
}
/**
* Scrolls down a list with a given {@code listIndex}.
*
* @param listIndex the {@link ListView} to be scrolled. {@code 0} if only one list is available
* @return {@code true} if more scrolling can be done
*
*/
public boolean scrollDownList(int listIndex) {
return scroller.scrollList(listIndex, Scroller.Direction.DOWN, null);
}
/**
* Scrolls up a list with a given {@code listIndex}.
*
* @param listIndex the {@link ListView} to be scrolled. {@code 0} if only one list is available
* @return {@code true} if more scrolling can be done
*
*/
public boolean scrollUpList(int listIndex) {
return scroller.scrollList(listIndex, Scroller.Direction.UP, null);
}
/**
* Scrolls horizontally.
*
* @param side the side to which to scroll; {@link #RIGHT} or {@link #LEFT}
*
*/
public void scrollToSide(int side) {
switch (side){
case RIGHT: scroller.scrollToSide(Scroller.Side.RIGHT); break;
case LEFT: scroller.scrollToSide(Scroller.Side.LEFT); break;
}
}
/**
* Sets the date in a {@link DatePicker} with a given index.
*
* @param index the index of the {@code DatePicker}. {@code 0} if only one is available
* @param year the year e.g. 2011
* @param monthOfYear the month e.g. 03
* @param dayOfMonth the day e.g. 10
*
*/
public void setDatePicker(int index, int year, int monthOfYear, int dayOfMonth) {
waiter.waitForView(DatePicker.class, index);
ArrayList visibleDatePickers = RobotiumUtils.removeInvisibleViews(getCurrentDatePickers());
if(index > visibleDatePickers.size()-1)
Assert.assertTrue("DatePicker with index " + index + " is not available!", false);
setter.setDatePicker(visibleDatePickers.get(index), year, monthOfYear, dayOfMonth);
}
/**
* Sets the date in a given {@link DatePicker}.
*
* @param datePicker the {@code DatePicker} object.
* @param year the year e.g. 2011
* @param monthOfYear the month e.g. 03
* @param dayOfMonth the day e.g. 10
*
*/
public void setDatePicker(DatePicker datePicker, int year, int monthOfYear, int dayOfMonth) {
waiter.waitForView(DatePicker.class, 0);
setter.setDatePicker(datePicker, year, monthOfYear, dayOfMonth);
}
/**
* Sets the time in a {@link TimePicker} with a given index.
*
* @param index the index of the {@code TimePicker}. {@code 0} if only one is available
* @param hour the hour e.g. 15
* @param minute the minute e.g. 30
*
*/
public void setTimePicker(int index, int hour, int minute) {
waiter.waitForView(TimePicker.class, index);
ArrayList visibleTimePickers = RobotiumUtils.removeInvisibleViews(getCurrentTimePickers());
if(index > visibleTimePickers.size()-1)
Assert.assertTrue("TimePicker with index " + index + " is not available!", false);
setter.setTimePicker(visibleTimePickers.get(index), hour, minute);
}
/**
* Sets the time in a given {@link TimePicker}.
*
* @param timePicker the {@code TimePicker} object.
* @param hour the hour e.g. 15
* @param minute the minute e.g. 30
*
*/
public void setTimePicker(TimePicker timePicker, int hour, int minute) {
waiter.waitForView(TimePicker.class, 0);
setter.setTimePicker(timePicker, hour, minute);
}
/**
* Sets the progress of a {@link ProgressBar} with a given index. Examples are SeekBar and RatingBar.
*
* @param index the index of the {@code ProgressBar}
* @param progress the progress that the {@code ProgressBar} should be set to
*
*/
public void setProgressBar(int index, int progress){
waiter.waitForView(ProgressBar.class, index);
ArrayList visibleProgressBars = RobotiumUtils.removeInvisibleViews(getCurrentProgressBars());
if(index > visibleProgressBars.size()-1)
Assert.assertTrue("ProgressBar with index " + index + " is not available!", false);
setter.setProgressBar(visibleProgressBars.get(index), progress);
}
/**
* Sets the progress of a given {@link ProgressBar}. Examples are SeekBar and RatingBar.
*
* @param progressBar the {@code ProgressBar}
* @param progress the progress that the {@code ProgressBar} should be set to
*
*/
public void setProgressBar(ProgressBar progressBar, int progress){
waiter.waitForView(ProgressBar.class, 0);
setter.setProgressBar(progressBar, progress);
}
/**
* Enters text into an {@link EditText} with a given index.
*
* @param index the index of the {@code EditText}. {@code 0} if only one is available
* @param text the text string to enter into the {@code EditText} field
*
*/
public void enterText(int index, String text) {
waiter.waitForView(EditText.class, index);
ArrayList visibleEditTexts = RobotiumUtils.removeInvisibleViews(getCurrentEditTexts());
if(index > visibleEditTexts.size()-1)
Assert.assertTrue("EditText with index " + index + " is not available!", false);
textEnterer.setEditText(visibleEditTexts.get(index), text);
}
/**
* Enters text into a given {@link EditText}.
*
* @param editText the {@code EditText} to enter text into
* @param text the text string to enter into the {@code EditText} field
*
*/
public void enterText(EditText editText, String text) {
waiter.waitForView(EditText.class, 0);
textEnterer.setEditText(editText, text);
}
/**
* Clicks on an {@link ImageView} with a given index.
*
* @param index the index of the {@link ImageView} to be clicked. {@code 0} if only one is available
*
*/
public void clickOnImage(int index) {
clicker.clickOn(ImageView.class, index);
}
/**
* Returns an {@code ArrayList} of the {@code ImageView} objects contained in the current
* {@code Activity}.
*
* @return an {@code ArrayList} of the {@code ImageView} objects contained in the current
* {@code Activity}
*
*/
public ArrayList getCurrentImageViews() {
return viewFetcher.getCurrentViews(ImageView.class);
}
/**
* Returns an {@code EditText} with a given index.
*
* @param index the index of the {@code EditText}. {@code 0} if only one is available
* @return the {@code EditText} with a specified index or {@code null} if index is invalid
*
*/
public EditText getEditText(int index) {
EditText editText = viewFetcher.getView(EditText.class, index);
return editText;
}
/**
* Returns a {@code Button} with a given index.
*
* @param index the index of the {@code Button}. {@code 0} if only one is available
* @return the {@code Button} with a specified index or {@code null} if index is invalid
*
*/
public Button getButton(int index) {
Button button = viewFetcher.getView(Button.class, index);
return button;
}
/**
* Returns a {@code TextView} with a given index.
*
* @param index the index of the {@code TextView}. {@code 0} if only one is available
* @return the {@code TextView} with a specified index or {@code null} if index is invalid
*
*/
public TextView getText(int index) {
return viewFetcher.getView(TextView.class, index);
}
/**
* Returns an {@code ImageView} with a given index.
*
* @param index the index of the {@code ImageView}. {@code 0} if only one is available
* @return the {@code ImageView} with a specified index or {@code null} if index is invalid
*
*/
public ImageView getImage(int index) {
return viewFetcher.getView(ImageView.class, index);
}
/**
* Returns an {@code ImageButton} with a given index.
*
* @param index the index of the {@code ImageButton}. {@code 0} if only one is available
* @return the {@code ImageButton} with a specified index or {@code null} if index is invalid
*
*/
public ImageButton getImageButton(int index) {
return viewFetcher.getView(ImageButton.class, index);
}
/**
* Returns a {@link TextView} which shows a given text.
*
* @param text the text that is shown
* @return the {@code TextView} that shows the given text
*/
public TextView getText(String text)
{
return viewFetcher.getView(TextView.class, text);
}
/**
* Returns a {@link Button} which shows a given text.
*
* @param text the text that is shown
* @return the {@code Button} that shows the given text
*/
public Button getButton(String text)
{
return viewFetcher.getView(Button.class, text);
}
/**
* Returns an {@link EditText} which shows a given text.
*
* @param text the text that is shown
* @return the {@code EditText} which shows the given text
*/
public EditText getEditText(String text)
{
return viewFetcher.getView(EditText.class, text);
}
/**
* Returns a {@code View} with a given id.
*
* @param id the R.id of the {@code View} to be returned
* @return a {@code View} with a given id
*/
public View getView(int id){
return viewFetcher.getView(id);
}
/**
* Returns an {@code ArrayList} of the {@code EditText} objects contained in the current
* {@code Activity}.
*
* @return an {@code ArrayList} of the {@code EditText} objects contained in the current
* {@code Activity}
*
*/
public ArrayList getCurrentEditTexts() {
return viewFetcher.getCurrentViews(EditText.class);
}
/**
* Returns an {@code ArrayList} of the {@code ListView} objects contained in the current
* {@code Activity}.
*
* @return an {@code ArrayList} of the {@code ListView} objects contained in the current
* {@code Activity}
*
*/
public ArrayList getCurrentListViews() {
return viewFetcher.getCurrentViews(ListView.class);
}
/**
* Returns an {@code ArrayList} of the {@code ScrollView} objects contained in the current
* {@code Activity}.
*
* @return an {@code ArrayList} of the {@code ScrollView} objects contained in the current
* {@code Activity}
*
*/
public ArrayList getCurrentScrollViews() {
return viewFetcher.getCurrentViews(ScrollView.class);
}
/**
* Returns an {@code ArrayList} of the {@code Spinner} objects (drop-down menus) contained in the current
* {@code Activity}.
*
* @return an {@code ArrayList} of the {@code Spinner} objects (drop-down menus) contained in the current
* {@code Activity}
*
*/
public ArrayList getCurrentSpinners() {
return viewFetcher.getCurrentViews(Spinner.class);
}
/**
* Returns an {@code ArrayList} of the {@code TextView} objects contained in the current
* {@code Activity} or {@code View}.
*
* @param parent the parent {@code View} from which the {@code TextView} objects should be returned. {@code null} if
* all {@code TextView} objects from the current {@code Activity} should be returned
*
* @return an {@code ArrayList} of the {@code TextView} objects contained in the current
* {@code Activity} or {@code View}
*
*/
public ArrayList getCurrentTextViews(View parent) {
return viewFetcher.getCurrentViews(TextView.class, parent);
}
/**
* Returns an {@code ArrayList} of the {@code GridView} objects contained in the current
* {@code Activity}.
*
* @return an {@code ArrayList} of the {@code GridView} objects contained in the current
* {@code Activity}
*
*/
public ArrayList getCurrentGridViews() {
return viewFetcher.getCurrentViews(GridView.class);
}
/**
* Returns an {@code ArrayList} of the {@code Button} objects located in the current
* {@code Activity}.
*
* @return an {@code ArrayList} of the {@code Button} objects located in the current {@code Activity}
*
*/
public ArrayList
© 2015 - 2025 Weber Informatics LLC | Privacy Policy