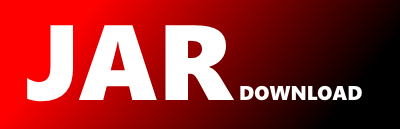
com.jayway.restassured.specification.RequestSpecification Maven / Gradle / Ivy
Show all versions of rest-assured Show documentation
/*
* Copyright 2010 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jayway.restassured.specification;
import groovyx.net.http.ContentType;
import java.util.Map;
/**
* Allows you to specify how the request will look like.
*/
public interface RequestSpecification extends RequestSender {
/**
* Specify a String request body (such as e.g. JSON or XML) that'll be sent with the request. This works for the
* POST and PUT methods only. Trying to do this for the other http methods will cause an exception to be thrown.
*
* Example of use:
*
* given().body("{ \"message\" : \"hello world\"}").then().expect().body(equalTo("hello world")).when().post("/json");
*
* This will POST a request containing JSON to "/json" and expect that the response body equals to "hello world".
*
*
*
* Note that {@link #body(String)} and {@link #content(String)} are the same except for the syntactic difference.
*
*
* @param body The body to send.
* @return The request com.jayway.restassured.specification
*/
RequestSpecification body(String body);
/**
* Specify a byte array request body that'll be sent with the request. This only works for the
* POST http method. Trying to do this for the other http methods will cause an exception to be thrown.
*
* Example of use:
*
* byte[] someBytes = ..
* given().body(someBytes).then().expect().body(equalTo("hello world")).when().post("/json");
*
* This will POST a request containing someBytes
to "/json" and expect that the response body equals to "hello world".
*
*
*
* Note that {@link #body(byte[])} and {@link #content(byte[])} are the same except for the syntactic difference.
*
*
* @param body The body to send.
* @return The request com.jayway.restassured.specification
*/
RequestSpecification body(byte[] body);
/**
* Specify a String request content (such as e.g. JSON or XML) that'll be sent with the request. This works for the
* POST and PUT methods only. Trying to do this for the other http methods will cause an exception to be thrown.
*
* Example of use:
*
* given().content("{ \"message\" : \"hello world\"}").then().expect().content(equalTo("hello world")).when().post("/json");
*
* This will POST a request containing JSON to "/json" and expect that the response content equals to "hello world".
*
*
*
* Note that {@link #body(String)} and {@link #content(String)} are the same except for the syntactic difference.
*
*
* @param content The content to send.
* @return The request com.jayway.restassured.specification
*/
RequestSpecification content(String content);
/**
* Specify a byte array request content that'll be sent with the request. This only works for the
* POST http method. Trying to do this for the other http methods will cause an exception to be thrown.
*
* Example of use:
*
* byte[] someBytes = ..
* given().content(someBytes).then().expect().content(equalTo("hello world")).when().post("/json");
*
* This will POST a request containing someBytes
to "/json" and expect that the response content equals to "hello world".
*
*
*
* Note that {@link #body(byte[])} and {@link #content(byte[])} are the same except for the syntactic difference.
*
*
* @param content The content to send.
* @return The request com.jayway.restassured.specification
*/
RequestSpecification content(byte[] content);
/**
* Specify the cookies that'll be sent with the request. This is done by specifying the cookies in name-value pairs, e.g:
*
* given().cookies("username", "John", "token", "1234").then().expect().body(equalTo("username, token")).when().get("/cookie");
*
*
* This will send a GET request to "/cookie" with two cookies:
*
* - username=John
* - token=1234
*
* and expect that the response body is equal to "username, token".
*
* @param cookieName The name of the first cookie
* @param cookieNameValuePairs The value of the first cookie followed by additional cookies in name-value pairs.
* @return The request com.jayway.restassured.specification
*/
RequestSpecification cookies(String cookieName, String...cookieNameValuePairs);
/**
* Specify the cookies that'll be sent with the request as Map e.g:
*
* Map<String, String> cookies = new HashMap<String, String>();
* cookies.put("username", "John");
* cookies.put("token", "1234");
* given().cookies(cookies).then().expect().body(equalTo("username, token")).when().get("/cookie");
*
*
* This will send a GET request to "/cookie" with two cookies:
*
* - username=John
* - token=1234
*
* and expect that the response body is equal to "username, token".
*
* @param cookies The Map containing the cookie names and their values to set in the request.
* @return The request com.jayway.restassured.specification
*/
RequestSpecification cookies(Map cookies);
/**
* Specify a cookie that'll be sent with the request e.g:
*
*
* given().cookie("username", "John").and().expect().body(equalTo("username")).when().get("/cookie");
*
* This will set the cookie username=John
in the GET request to "/cookie".
*
*
*
* You can also specify several cookies like this:
*
* given().cookie("username", "John").and().cookie("password", "1234").and().expect().body(equalTo("username")).when().get("/cookie");
*
*
*
* @see #cookies(String, String...)
* @param key The cookie key
* @param value The cookie value
* @return The request com.jayway.restassured.specification
*/
RequestSpecification cookie(String key, String value);
/**
* Specify the parameters that'll be sent with the request. This is done by specifying the parameters in name-value pairs, e.g:
*
* given().parameters("username", "John", "token", "1234").then().expect().body(equalTo("username, token")).when().get("/parameters");
*
*
* This will send a GET request to "/parameters" with two parameters:
*
* - username=John
* - token=1234
*
* and expect that the response body is equal to "username, token".
*
* @param parameterName The name of the first parameter
* @param parameterNameValuePairs The value of the first parameter followed by additional parameters in name-value pairs.
* @return The request com.jayway.restassured.specification
*/
RequestSpecification parameters(String parameterName, String...parameterNameValuePairs);
/**
* Specify the parameters that'll be sent with the request as Map e.g:
*
* Map<String, String> parameters = new HashMap<String, String>();
* parameters.put("username", "John");
* parameters.put("token", "1234");
* given().parameters(parameters).then().expect().body(equalTo("username, token")).when().get("/cookie");
*
*
* This will send a GET request to "/cookie" with two parameters:
*
* - username=John
* - token=1234
*
* and expect that the response body is equal to "username, token".
*
* @param parametersMap The Map containing the parameter names and their values to send with the request.
* @return The request com.jayway.restassured.specification
*/
RequestSpecification parameters(Map parametersMap);
/**
* Specify a parameter that'll be sent with the request e.g:
*
*
* given().parameter("username", "John").and().expect().body(equalTo("username")).when().get("/cookie");
*
* This will set the parameter username=John
in the GET request to "/cookie".
*
*
*
* You can also specify several parameters like this:
*
* given().parameter("username", "John").and().parameter("password", "1234").and().expect().body(equalTo("username")).when().get("/cookie");
*
*
*
* @see #parameters(String, String...)
* @param parameterName The parameter key
* @param parameterValue The parameter value
* @return The request com.jayway.restassured.specification
*/
RequestSpecification parameter(String parameterName, String parameterValue);
/**
* A slightly shorter version of {@link #parameters(String, String...)}.
*
* @see #parameters(String, String...)
* @param parameterName The name of the first parameter
* @param parameterNameValuePairs The value of the first parameter followed by additional parameters in name-value pairs.
* @return The request com.jayway.restassured.specification
*/
RequestSpecification params(String parameterName, String...parameterNameValuePairs);
/**
* A slightly shorter version of {@link #parameters(Map)}.
*
* @see #parameters(Map)
* @param parametersMap The Map containing the parameter names and their values to send with the request.
* @return The request com.jayway.restassured.specification
*/
RequestSpecification params(Map parametersMap);
/**
* A slightly shorter version of {@link #parameter(String, String) }.
*
* @see #parameter(String, String)
* @param parameterName The parameter key
* @param parameterValue The parameter value
* @return The request com.jayway.restassured.specification
*/
RequestSpecification param(String parameterName, String parameterValue);
/**
* Specify the headers that'll be sent with the request. This is done by specifying the headers in name-value pairs, e.g:
*
* given().headers("headerName1", "headerValue1", "headerName2", "headerValue2").then().expect().body(equalTo("something")).when().get("/headers");
*
*
* This will send a GET request to "/headers" with two headers:
*
* - headerName1=headerValue1
* - headerName2=headerValue2
*
* and expect that the response body is equal to "something".
*
* @param headerName The name of the first header
* @param headerNameValuePairs The value of the first header followed by additional headers in name-value pairs.
* @return The request com.jayway.restassured.specification
*/
RequestSpecification headers(String headerName, String ... headerNameValuePairs);
/**
* Specify the headers that'll be sent with the request as Map e.g:
*
* Map<String, String> headers = new HashMap<String, String>();
* parameters.put("headerName1", "headerValue1");
* parameters.put("headerName2", "headerValue2");
* given().headers(headers).then().expect().body(equalTo("something")).when().get("/headers");
*
*
* This will send a GET request to "/headers" with two headers:
*
* - headerName1=headerValue1
* - headerName2=headerValue2
*
* and expect that the response body is equal to "something".
*
* @param headers The Map containing the header names and their values to send with the request.
* @return The request com.jayway.restassured.specification
*/
RequestSpecification headers(Map headers);
/**
* Specify a header that'll be sent with the request e.g:
*
*
* given().header("username", "John").and().expect().body(equalTo("something")).when().get("/header");
*
* This will set the header username=John
in the GET request to "/header".
*
*
*
* You can also specify several headers like this:
*
* given().header("username", "John").and().header("zipCode", "12345").and().expect().body(equalTo("something")).when().get("/header");
*
*
*
* @see #headers(String, String...)
* @param headerName The header name
* @param headerValue The header value
* @return The request com.jayway.restassured.specification
*/
RequestSpecification header(String headerName, String headerValue);
/**
* Specify the content type of the request.
*
* @see ContentType
* @param contentType The content type of the request
* @return The request com.jayway.restassured.specification
*/
RequestSpecification contentType(ContentType contentType);
/**
* If you need to specify some credentials when performing a request.
*
* @see com.jayway.restassured.specification.AuthenticationSpecification
* @return The authentication com.jayway.restassured.specification
*/
AuthenticationSpecification authentication();
/**
* A slightly short version of {@link #authentication()}.
*
* @see #authentication()
* @see com.jayway.restassured.specification.AuthenticationSpecification
* @return The authentication com.jayway.restassured.specification
*/
AuthenticationSpecification auth();
/**
* Specify the port of the URI. E.g.
*
*
* given().port(8081).and().expect().statusCode(200).when().get("/something");
*
* will perform a GET request to http;//localhost:8081/something. It will override the default port of
* REST assured for this request only.
*
*
* Note that it's also possible to specify the port like this:
*
* expect().statusCode(200).when().get("http://localhost:8081/something");
*
*
*
* @param port The port of URI
* @return The request com.jayway.restassured.specification
*/
RequestSpecification port(int port);
/**
* Returns the response com.jayway.restassured.specification so that you can setup the expectations on the response. E.g.
*
* given().param("name", "value").then().response().body(equalTo("something")).when().get("/something");
*
*
* @return the response com.jayway.restassured.specification
*/
ResponseSpecification response();
/**
* Syntactic sugar, e.g.
*
* expect().body(containsString("OK")).and().body(containsString("something else")).when().get("/something");
*
*
* is that same as:
*
* expect().body(containsString("OK")).body(containsString("something else")).when().get("/something");
*
*
* @return the request com.jayway.restassured.specification
*/
RequestSpecification and();
/**
* Syntactic sugar, e.g.
*
* expect().body(containsString("OK")).and().with().request().parameters("param1", "value1").get("/something");
*
*
* is that same as:
*
* expect().body(containsString("OK")).and().request().parameters("param1", "value1").get("/something");
*
*
* @return the request com.jayway.restassured.specification
*/
RequestSpecification with();
/**
* Returns the response com.jayway.restassured.specification so that you can setup the expectations on the response. E.g.
*
* given().param("name", "value").then().body(equalTo("something")).when().get("/something");
*
*
* @return the response com.jayway.restassured.specification
*/
ResponseSpecification then();
/**
* Returns the response com.jayway.restassured.specification so that you can setup the expectations on the response. E.g.
*
* given().param("name", "value").and().expect().body(equalTo("something")).when().get("/something");
*
*
* @return the response com.jayway.restassured.specification
*/
ResponseSpecification expect();
/**
* Syntactic sugar, e.g.
*
* expect().body(containsString("OK")).when().get("/something");
*
*
* is that same as:
*
* expect().body(containsString("OK")).get("/something");
*
*
* @return the request com.jayway.restassured.specification
*/
RequestSpecification when();
/**
* Syntactic sugar, e.g.
*
* given().param("name1", "value1").and().given().param("name2", "value2").when().get("/something");
*
*
* is that same as:
*
* given().param("name1", "value1").and().param("name2", "value2").when().get("/something");
*
*
* @return the request com.jayway.restassured.specification
*/
RequestSpecification given();
/**
* Syntactic sugar, e.g.
*
* expect().that().body(containsString("OK")).when().get("/something");
*
*
* is that same as:
*
* expect().body(containsString("OK")).get("/something");
*
*
* @return the request com.jayway.restassured.specification
*/
RequestSpecification that();
/**
* Syntactic sugar, e.g.
*
* given().request().param("name", "John").then().expect().body(containsString("OK")).when().get("/something");
*
*
* is that same as:
*
* given().param("name", "John").then().expect().body(containsString("OK")).when().get("/something");
*
*
* @return the request com.jayway.restassured.specification
*/
RequestSpecification request();
}