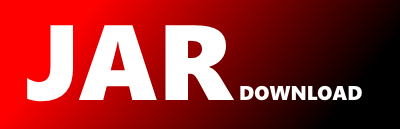
com.jayway.restassured.RestAssured Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-assured Show documentation
Show all versions of rest-assured Show documentation
Java DSL for easy testing of REST services
/*
* Copyright 2010 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jayway.restassured;
import com.jayway.restassured.authentication.*;
import com.jayway.restassured.internal.RequestSpecificationImpl;
import com.jayway.restassured.internal.ResponseParserRegistrar;
import com.jayway.restassured.internal.ResponseSpecificationImpl;
import com.jayway.restassured.internal.TestSpecificationImpl;
import com.jayway.restassured.parsing.Parser;
import com.jayway.restassured.response.Response;
import com.jayway.restassured.specification.RequestSender;
import com.jayway.restassured.specification.RequestSpecification;
import com.jayway.restassured.specification.ResponseSpecification;
/**
* REST Assured is a Java DSL for simplifying testing of REST based services built on top of
* HTTP Builder.
* It supports POST, GET, PUT, DELETE and HEAD
* requests and to verify the response of these requests. Usage examples:
*
* -
* Assume that the GET request (to http://localhost:8080/lotto) returns JSON as:
*
* {
* "lotto":{
* "lottoId":5,
* "winning-numbers":[2,45,34,23,7,5,3],
* "winners":[{
* "winnerId":23,
* "numbers":[2,45,34,23,3,5]
* },{
* "winnerId":54,
* "numbers":[52,3,12,11,18,22]
* }]
* }
* }
*
*
* REST assured can then help you to easily make the GET request and verify the response. E.g. if you want to verify
* that lottoId is equal to 5 you can do like this:
*
*
* expect().body("lotto.lottoId", equalTo(5)).when().get("/lotto");
*
*
* or perhaps you want to check that the winnerId's are 23 and 54:
*
* expect().body("lotto.winners.winnerId", hasItems(23, 54)).when().get("/lotto");
*
*
* -
* XML can be verified in a similar way. Image that a POST request to http://localhost:8080/greetXML returns:
*
* <greeting>
* <firstName>{params("firstName")}</firstName>
* <lastName>{params("lastName")}</lastName>
* </greeting>
*
*
* i.e. it sends back a greeting based on the firstName and lastName parameter sent in the request.
* You can easily perform and verify e.g. the firstName with REST assured:
*
* with().parameters("firstName", "John", "lastName", "Doe").expect().body("greeting.firstName", equalTo("John")).when().post("/greetXML");
*
*
* If you want to verify both firstName and lastName you may do like this:
*
* with().parameters("firstName", "John", "lastName", "Doe").expect().body("greeting.firstName", equalTo("John")).and().body("greeting.lastName", equalTo("Doe")).when().post("/greetXML");
*
*
* or a little shorter:
*
* with().parameters("firstName", "John", "lastName", "Doe").expect().body("greeting.firstName", equalTo("John"), "greeting.lastName", equalTo("Doe")).when().post("/greetXML");
*
*
* -
* You can also verify XML responses using x-path. For example:
*
* expect().body(hasXPath("/greeting/firstName", containsString("Jo"))).given().parameters("firstName", "John", "lastName", "Doe").when().post("/greetXML");
*
* or
*
* expect().body(hasXPath("/greeting/firstName[text()='John']")).with().parameters("firstName", "John", "lastName", "Doe").post("/greetXML");
*
*
* -
* XML response bodies can also be verified against an XML Schema (XSD) or DTD.
XSD example:
*
* expect().body(matchesXsd(xsd)).when().get("/carRecords");
*
* DTD example:
*
* expect().body(matchesDtd(dtd)).when().get("/videos");
*
* matchesXsd
and matchesDtd
are Hamcrest matchers which you can import from {@link com.jayway.restassured.matcher.RestAssuredMatchers}.
*
* -
* Besides specifying request parameters you can also specify headers, cookies, body and content type.
*
* -
* Cookie:
*
* given().cookie("username", "John").then().expect().body(equalTo("username")).when().get("/cookie");
*
*
* -
* Headers:
*
* given().header("MyHeader", "Something").and(). ..
* given().headers("MyHeader", "Something", "MyOtherHeader", "SomethingElse").and(). ..
*
*
* -
* Content Type:
*
* given().contentType(ContentType.TEXT). ..
*
*
* -
* Body:
*
* given().request().body("some body"). .. // Works for POST and PUT requests
* given().request().body(new byte[]{42}). .. // Works for POST
*
*
*
*
* -
* You can also verify status code, status line, cookies, headers, content type and body.
*
* -
* Cookie:
*
* expect().cookie("cookieName", "cookieValue"). ..
* expect().cookies("cookieName1", "cookieValue1", "cookieName2", "cookieValue2"). ..
* expect().cookies("cookieName1", "cookieValue1", "cookieName2", containsString("Value2")). ..
*
*
* -
* Status:
*
* expect().statusCode(200). ..
* expect().statusLine("something"). ..
* expect().statusLine(containsString("some")). ..
*
*
* -
* Headers:
*
* expect().header("headerName", "headerValue"). ..
* expect().headers("headerName1", "headerValue1", "headerName2", "headerValue2"). ..
* expect().headers("headerName1", "headerValue1", "headerName2", containsString("Value2")). ..
*
*
* -
* Content-Type:
*
* expect().contentType(ContentType.JSON). ..
*
*
* -
* Full body/content matching:
*
* expect().body(equalsTo("something")). ..
* expect().content(equalsTo("something")). .. // Same as above
*
*
*
*
* -
* REST assured also supports some authentication schemes, for example basic authentication:
*
* given().auth().basic("username", "password").expect().statusCode(200).when().get("/secured/hello");
*
* Other supported schemes are OAuth and certificate authentication.
*
* -
* By default REST assured assumes host localhost and port 8080 when doing a request. If you want a different port you can do:
*
* given().port(80). ..
*
* or simply:
*
* .. when().get("http://myhost.org:80/doSomething");
*
*
* -
* Parameters can also be set directly on the url:
*
* ..when().get("/name?firstName=John&lastName=Doe");
*
*
* -
* You can use the {@link com.jayway.restassured.path.xml.XmlPath} or {@link com.jayway.restassured.path.json.JsonPath} to
* easily parse XML or JSON data from a response.
*
* - XML example:
*
* String xml = post("/greetXML?firstName=John&lastName=Doe").andReturn().asString();
* // Now use XmlPath to get the first and last name
* String firstName = with(xml).get("greeting.firstName");
* String lastName = with(xml).get("greeting.firstName");
*
* // or a bit more efficiently:
* XmlPath xmlPath = new XmlPath(xml).setRoot("greeting");
* String firstName = xmlPath.get("firstName");
* String lastName = xmlPath.get("lastName");
*
*
* - JSON example:
*
* String json = get("/lotto").asString();
* // Now use JsonPath to get data out of the JSON body
* int lottoId = with(json).getInt("lotto.lottoId);
* List winnerIds = with(json).get("lotto.winners.winnerId");
*
* // or a bit more efficiently:
* JsonPath jsonPath = new JsonPath(json).setRoot("lotto");
* int lottoId = jsonPath.getInt("lottoId");
* List winnderIds = jsonPath.get("winnders.winnderId");
*
*
*
*
* -
* REST Assured providers predefined parsers for e.g. HTML, XML and JSON. But you can parse other kinds of content by registering a predefined parser for unsupported mime-types by using:
*
* RestAssured.registerParser(<mime-type>, <parser>);
*
* E.g. to register that mime-type 'application/vnd.uoml+xml'
should be parsed using the XML parser do:
*
* RestAssured.registerParser("application/vnd.uoml+xml", Parser.XML);
*
* You can also unregister a parser using:
*
* RestAssured.unregisterParser("application/vnd.uoml+xml");
*
*
* -
* You can also change the default base URI, base path, port and authentication scheme for all subsequent requests:
*
* RestAssured.baseURI = "http://myhost.org";
* RestAssured.port = 80;
* RestAssured.basePath = "/resource";
* RestAssured.authentication = basic("username", "password");
*
* This means that a request like e.g. get("/hello")
goes to: http://myhost.org:8080/resource/hello
* which basic authentication credentials "username" and "password".
* You can reset to the standard baseURI (localhost), basePath (empty), standard port (8080) and default authentication scheme (none) using:
*
* RestAssured.reset();
*
*
*
*
* In order to use REST assured effectively it's recommended to statically import
* methods from the following classes:
*
* - com.jayway.restassured.RestAssured.*
* - com.jayway.restassured.matcher.RestAssuredMatchers.*
* - org.hamcrest.Matchers.*
*
*
*/
public class RestAssured {
public static final String DEFAULT_URI = "http://localhost";
public static final int DEFAULT_PORT = 8080;
public static final String DEFAULT_PATH = "";
public static final AuthenticationScheme DEFAULT_AUTH = new NoAuthScheme();
/**
* The base URI that's used by REST assured when making requests if a non-fully qualified URI is used in the request.
* Default value is {@value #DEFAULT_URI}.
*/
public static String baseURI = DEFAULT_URI;
/**
* The port that's used by REST assured when is left out of the specified URI when making a request.
* Default value is {@value #DEFAULT_PORT}.
*/
public static int port = DEFAULT_PORT;
/**
* A base path that's added to the {@link #baseURI} by REST assured when making requests. E.g. let's say that
* the {@link #baseURI} is http://localhost
and basePath
is /resource
* then
*
*
* ..when().get("/something");
*
*
* will make a request to http://localhost/resource
.
* Default basePath
value is empty.
*/
public static String basePath = DEFAULT_PATH;
/**
* Set an authentication scheme that should be used for each request. By default no authentication is used.
* If you have specified an authentication scheme and wish to override it for a single request then
* you can do this using:
*
*
* given().auth().none()..
*
*
*/
public static AuthenticationScheme authentication = DEFAULT_AUTH;
/**
* Start building the response part of the test com.jayway.restassured.specification. E.g.
*
*
* expect().body("lotto.lottoId", equalTo(5)).when().get("/lotto");
*
*
* will expect that the response body for the GET request to "/lotto" should
* contain JSON or XML which has a lottoId equal to 5.
*
* @return A response com.jayway.restassured.specification.
*/
public static ResponseSpecification expect() {
return createTestSpecification().getResponseSpecification();
}
/**
* Start building the request part of the test com.jayway.restassured.specification. E.g.
*
*
* with().parameters("firstName", "John", "lastName", "Doe").expect().body("greeting.firstName", equalTo("John")).when().post("/greetXML");
*
*
* will send a POST request to "/greetXML" with request parameters firstName=John and lastName=Doe and
* expect that the response body containing JSON or XML firstName equal to John.
*
* The only difference between {@link #with()} and {@link #given()} is syntactical.
*
* @return A request com.jayway.restassured.specification.
*/
public static RequestSpecification with() {
return given();
}
/**
* Start building the request part of the test com.jayway.restassured.specification. E.g.
*
*
* given().parameters("firstName", "John", "lastName", "Doe").expect().body("greeting.firstName", equalTo("John")).when().post("/greetXML");
*
*
* will send a POST request to "/greetXML" with request parameters firstName=John and lastName=Doe and
* expect that the response body containing JSON or XML firstName equal to John.
*
* The only difference between {@link #with()} and {@link #given()} is syntactical.
*
* @return A request com.jayway.restassured.specification.
*/
public static RequestSpecification given() {
return createTestSpecification().getRequestSpecification();
}
/**
* When you have long specifications it can be better to split up the definition of response and request specifications in multiple lines.
* You can then pass the response and request specifications to this method. E.g.
*
*
* RequestSpecification requestSpecification = with().parameters("firstName", "John", "lastName", "Doe");
* ResponseSpecification responseSpecification = expect().body("greeting", equalTo("Greetings John Doe"));
* given(requestSpecification, responseSpecification).get("/greet");
*
*
* This will perform a GET request to "/greet" and verify it according to the responseSpecification
.
*
* @return A test com.jayway.restassured.specification.
*/
public static RequestSender given(RequestSpecification requestSpecification, ResponseSpecification responseSpecification) {
return new TestSpecificationImpl(requestSpecification, responseSpecification);
}
/**
* Perform a GET request to a path
. Normally the path doesn't have to be fully-qualified e.g. you don't need to
* specify the path as http://localhost:8080/path. In this case it's enough to use /path.
*
* @param path The path to send the request to.
* @return The response of the GET request. The response can only be returned if you don't use any REST Assured response expectations.
*/
public static Response get(String path) {
return given().get(path);
}
/**
* Perform a POST request to a path
. Normally the path doesn't have to be fully-qualified e.g. you don't need to
* specify the path as http://localhost:8080/path. In this case it's enough to use /path.
*
* @param path The path to send the request to.
* @return The response of the request. The response can only be returned if you don't use any REST Assured response expectations.
*/
public static Response post(String path) {
return given().post(path);
}
/**
* Perform a PUT request to a path
. Normally the path doesn't have to be fully-qualified e.g. you don't need to
* specify the path as http://localhost:8080/path. In this case it's enough to use /path.
*
* @param path The path to send the request to.
* @return The response of the request. The response can only be returned if you don't use any REST Assured response expectations.
*/
public static Response put(String path) {
return given().put(path);
}
/**
* Perform a DELETE request to a path
. Normally the path doesn't have to be fully-qualified e.g. you don't need to
* specify the path as http://localhost:8080/path. In this case it's enough to use /path.
*
* @param path The path to send the request to.
* @return The response of the request. The response can only be returned if you don't use any REST Assured response expectations.
*/
public static Response delete(String path) {
return given().delete(path);
}
/**
* Perform a HEAD request to a path
. Normally the path doesn't have to be fully-qualified e.g. you don't need to
* specify the path as http://localhost:8080/path. In this case it's enough to use /path.
*
* @param path The path to send the request to.
* @return The response of the request. The response can only be returned if you don't use any REST Assured response expectations.
*/
public static Response head(String path) {
return given().head(path);
}
/**
* Create a http basic authentication scheme.
*
* @param userName The user name.
* @param password The password.
* @return The authentication scheme
*/
public static AuthenticationScheme basic(String userName, String password) {
final BasicAuthScheme scheme = new BasicAuthScheme();
scheme.setUserName(userName);
scheme.setPassword(password);
return scheme;
}
/**
* Sets a certificate to be used for SSL authentication. See {@link Class#getResource(String)}
* for how to get a URL from a resource on the classpath.
*
* @param certURL URL to a JKS keystore where the certificate is stored.
* @param password password to decrypt the keystore
* @return The authentication scheme
*/
public static AuthenticationScheme certificate(String certURL, String password) {
final CertAuthScheme scheme = new CertAuthScheme();
scheme.setCertURL(certURL);
scheme.setPassword(password);
return scheme;
}
/**
* Use http digest authentication.
*
* @param userName The user name.
* @param password The password.
* @return The authentication scheme
*/
public static AuthenticationScheme digest(String userName, String password) {
return basic(userName, password);
}
/**
* Excerpt from the HttpBuilder docs:
* OAuth sign the request. Note that this currently does not wait for a WWW-Authenticate challenge before sending the the OAuth header.
* All requests to all domains will be signed for this instance.
* This assumes you've already generated an accessToken and secretToken for the site you're targeting.
* For More information on how to achieve this, see the Signpost documentation.
*
* @param consumerKey
* @param consumerSecret
* @param accessToken
* @param secretToken
* @return The authentication scheme
*/
public static AuthenticationScheme oauth(String consumerKey, String consumerSecret, String accessToken, String secretToken) {
OAuthScheme scheme = new OAuthScheme();
scheme.setConsumerKey(consumerKey);
scheme.setConsumerSecret(consumerSecret);
scheme.setAccessToken(accessToken);
scheme.setSecretToken(secretToken);
return scheme;
}
/**
* Register a custom mime-type to be parsed using a predefined parser. E.g. let's say you want parse
* mime-type application/vnd.uoml+xml with the XML parser to be able to verify the response using the XML dot notations:
*
* expect().body("document.child", equalsTo("something"))..
*
* Since application/vnd.uoml+xml is not registered to be processed by the XML parser by default you need to explicitly
* tell REST Assured to use this parser before making the request:
*
* RestAssured.registerParser("application/vnd.uoml+xml, Parser.XML");
*
*
* @param mimeType The mime-type to register
* @param parser The parser to use when verifying the response.
*/
public static void registerParser(String mimeType, Parser parser) {
ResponseParserRegistrar.registerParser(mimeType, parser);
}
/**
* Unregister the parser associated with the provided mime-type
*
* @param mimeType The mime-type associated with the parser to unregister.
*/
public static void unregisterParser(String mimeType) {
ResponseParserRegistrar.unregisterParser(mimeType);
}
/**
* Resets the {@link #baseURI}, {@link #basePath}, {@link #port} and {@link #authentication} to their default values of
* {@value #DEFAULT_URI}, {@value #DEFAULT_PATH}, {@value #DEFAULT_PORT} and no authentication
.
*/
public static void reset() {
baseURI = DEFAULT_URI;
port = DEFAULT_PORT;
basePath = DEFAULT_PATH;
authentication = DEFAULT_AUTH;
}
private static TestSpecificationImpl createTestSpecification() {
return new TestSpecificationImpl(new RequestSpecificationImpl(baseURI, port, basePath, authentication), new ResponseSpecificationImpl());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy