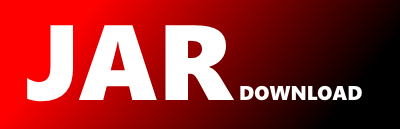
com.jayway.restassured.config.HttpClientConfig Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rest-assured Show documentation
Show all versions of rest-assured Show documentation
Java DSL for easy testing of REST services
/*
* Copyright 2013 the original author or authors.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package com.jayway.restassured.config;
import org.apache.http.client.params.ClientPNames;
import org.apache.http.client.params.CookiePolicy;
import org.apache.http.entity.mime.HttpMultipartMode;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import static com.jayway.restassured.internal.assertion.AssertParameter.notNull;
/**
* Configure the Apache HTTP Client parameters.
* Note that you can't configure the redirect settings from this config. Please use {@link RedirectConfig} for this purpose.
*
* The following parameters are applied per default:
*
*
* Parameter name Parameter value Description
*
*
* {@link ClientPNames#COOKIE_POLICY} {@link CookiePolicy#IGNORE_COOKIES} Don't automatically set response cookies in subsequent requests
*
*
*
* @see org.apache.http.client.params.ClientPNames
* @see org.apache.http.client.params.CookiePolicy
* @see org.apache.http.params.CoreProtocolPNames
*/
public class HttpClientConfig {
private final Map httpClientParams;
private final HttpMultipartMode httpMultipartMode;
/**
* Creates a new HttpClientConfig instance with the {@value ClientPNames#COOKIE_POLICY}
parameter set to {@value CookiePolicy#IGNORE_COOKIES}
.
*/
public HttpClientConfig() {
this.httpClientParams = new HashMap() {
{
put(ClientPNames.COOKIE_POLICY, CookiePolicy.IGNORE_COOKIES);
}
};
this.httpMultipartMode = HttpMultipartMode.STRICT;
}
private HttpClientConfig(Map httpClientParams, HttpMultipartMode httpMultipartMode) {
notNull(httpClientParams, "httpClientParams");
notNull(httpMultipartMode, "httpMultipartMode");
this.httpClientParams = new HashMap(httpClientParams);
this.httpMultipartMode = httpMultipartMode;
}
/**
* Creates a new HttpClientConfig instance with the parameters defined by the httpClientParams
.
*/
public HttpClientConfig(Map httpClientParams) {
this(httpClientParams, HttpMultipartMode.STRICT);
}
/**
* @return The configured parameters
*/
public Map params() {
return Collections.unmodifiableMap(httpClientParams);
}
/**
* @return The same HttpClientConfig instance. Only here for syntactic sugar.
*/
public HttpClientConfig and() {
return this;
}
/**
* Set a http client parameter.
*
* @param parameterName The name of the parameter
* @param parameterValue The value of the parameter (may be null)
* @param The parameter type
* @return An updated HttpClientConfig
*/
public HttpClientConfig setParam(String parameterName, T parameterValue) {
notNull(parameterName, "Parameter name");
final Map newParams = new HashMap(httpClientParams);
newParams.put(parameterName, parameterValue);
return new HttpClientConfig(newParams);
}
/**
* Replaces the currently configured parameters with the ones supplied by httpClientParams
. This method is the same as {@link #setParams(java.util.Map)}.
*
* @param httpClientParams The parameters to set.
* @return An updated HttpClientConfig
*/
public HttpClientConfig withParams(Map httpClientParams) {
return new HttpClientConfig(httpClientParams);
}
/**
* Replaces the currently configured parameters with the ones supplied by httpClientParams
. This method is the same as {@link #withParams(java.util.Map)}.
*
* @param httpClientParams The parameters to set.
* @return An updated HttpClientConfig
*/
public HttpClientConfig setParams(Map httpClientParams) {
return withParams(httpClientParams);
}
/**
* Add the given parameters to an already configured number of parameters.
*
* @param httpClientParams The parameters.
* @return An updated HttpClientConfig
*/
public HttpClientConfig addParams(Map httpClientParams) {
notNull(httpClientParams, "httpClientParams");
final Map newParams = new HashMap(httpClientParams);
newParams.putAll(httpClientParams);
return new HttpClientConfig(newParams);
}
/**
* Specify the HTTP Multipart mode when sending multi-part data.
*
* @param httpMultipartMode The multi-part mode to set.
* @return An updated HttpClientConfig
*/
public HttpClientConfig httpMultipartMode(HttpMultipartMode httpMultipartMode) {
return new HttpClientConfig(httpClientParams, httpMultipartMode);
}
/**
* @return A static way to create a new HttpClientConfig instance without calling "new" explicitly. Mainly for syntactic sugar.
*/
public static HttpClientConfig httpClientConfig() {
return new HttpClientConfig();
}
/**
* @return The http multi-part mode.
*/
public HttpMultipartMode httpMultipartMode() {
return httpMultipartMode;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy