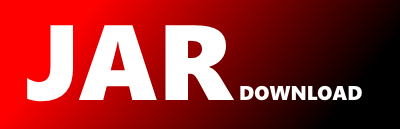
com.jd.blockchain.consensus.AsyncInvoker Maven / Gradle / Ivy
package com.jd.blockchain.consensus;
import java.lang.reflect.InvocationHandler;
import java.lang.reflect.Method;
import java.lang.reflect.Proxy;
import utils.concurrent.AsyncFuture;
public class AsyncInvoker {
private static ThreadLocal> resultHolder;
static {
resultHolder = new ThreadLocal<>();
}
@SuppressWarnings("unchecked")
public static T asynchorize(Class serviceClazz, T serviceInstance) {
if (serviceInstance instanceof AsyncService) {
return (T) Proxy.newProxyInstance(serviceClazz.getClassLoader(), new Class>[] { serviceClazz },
new AsyncInvocationHandle(serviceClazz, (AsyncService) serviceInstance));
}
throw new IllegalArgumentException("The specified service instance is not supported by this asynchronize util!");
}
@SuppressWarnings("unchecked")
public static AsyncFuture call(T methodCall){
AsyncFuture result = (AsyncFuture) resultHolder.get();
resultHolder.set(null);
return result;
}
private static class AsyncInvocationHandle implements InvocationHandler {
private Class serviceClazz;
private AsyncService serviceInstance;
public AsyncInvocationHandle(Class serviceClazz, AsyncService serviceInstance) {
this.serviceInstance = serviceInstance;
this.serviceClazz = serviceClazz;
}
@Override
public Object invoke(Object proxy, Method method, Object[] args) throws Throwable {
resultHolder.remove();
if (method.getDeclaringClass() == serviceClazz) {
//async invoke;
AsyncFuture
© 2015 - 2025 Weber Informatics LLC | Privacy Policy