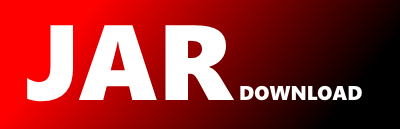
com.jdroid.java.http.urlconnection.UrlConnectionHttpService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of jdroid-java-http-urlconnection Show documentation
Show all versions of jdroid-java-http-urlconnection Show documentation
Url Connection Http Layer Implementation for Jdroid
package com.jdroid.java.http.urlconnection;
import com.jdroid.java.exception.ConnectionException;
import com.jdroid.java.exception.UnexpectedException;
import com.jdroid.java.http.AbstractHttpService;
import com.jdroid.java.http.HttpResponseWrapper;
import com.jdroid.java.http.HttpServiceProcessor;
import com.jdroid.java.http.Server;
import java.io.FileNotFoundException;
import java.io.IOException;
import java.net.ConnectException;
import java.net.HttpURLConnection;
import java.net.MalformedURLException;
import java.net.SocketTimeoutException;
import java.net.URL;
import java.net.URLConnection;
import java.util.List;
import java.util.Map;
public abstract class UrlConnectionHttpService extends AbstractHttpService {
private HttpURLConnection urlConnection;
public UrlConnectionHttpService(Server server, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy