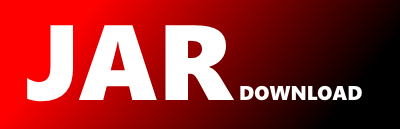
com.jdroid.java.collections.Iterables Maven / Gradle / Ivy
package com.jdroid.java.collections;
import java.util.Collection;
public class Iterables {
/**
* Returns the number of elements in {@code iterable}.
*
* @param iterable The {@link Iterable}
* @return The {@link Iterable} size
*/
public static int size(Iterable> iterable) {
return (iterable instanceof Collection) ? ((Collection>)iterable).size()
: Iterators.size(iterable.iterator());
}
/**
* Copies an iterable's elements into an array.
*
* @param iterable the iterable to copy
* @param type the type of the elements
* @return a newly-allocated array into which all the elements of the iterable have been copied
*/
public static T[] toArray(Iterable extends T> iterable, Class type) {
Collection extends T> collection = toCollection(iterable);
T[] array = ObjectArrays.newArray(type, collection.size());
return collection.toArray(array);
}
/**
* Converts an iterable into a collection. If the iterable is already a collection, it is returned. Otherwise, an
* {@link java.util.ArrayList} is created with the contents of the iterable in the same iteration order.
*/
private static Collection toCollection(Iterable iterable) {
return (iterable instanceof Collection) ? (Collection)iterable : Lists.newArrayList(iterable.iterator());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy