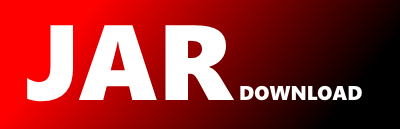
com.jdroid.github.service.StargazerService Maven / Gradle / Ivy
/******************************************************************************
* Copyright (c) 2015 Jon Ander Peñalba
* All rights reserved. This program and the accompanying materials
* are made available under the terms of the Eclipse Public License v1.0
* which accompanies this distribution, and is available at
* http://www.eclipse.org/legal/epl-v10.html
*
* Contributors:
* Jon Ander Peñalba - initial API and implementation
*****************************************************************************/
package com.jdroid.github.service;
import com.google.gson.reflect.TypeToken;
import com.jdroid.github.IRepositoryIdProvider;
import com.jdroid.github.Repository;
import com.jdroid.github.User;
import com.jdroid.github.client.GitHubClient;
import com.jdroid.github.client.PageIterator;
import com.jdroid.github.client.PagedRequest;
import java.io.IOException;
import java.util.List;
import static com.jdroid.github.client.IGitHubConstants.SEGMENT_REPOS;
import static com.jdroid.github.client.IGitHubConstants.SEGMENT_STARGAZERS;
import static com.jdroid.github.client.IGitHubConstants.SEGMENT_STARRED;
import static com.jdroid.github.client.IGitHubConstants.SEGMENT_USER;
import static com.jdroid.github.client.IGitHubConstants.SEGMENT_USERS;
import static com.jdroid.github.client.PagedRequest.PAGE_FIRST;
import static com.jdroid.github.client.PagedRequest.PAGE_SIZE;
/**
* Service class for dealing with users starring GitHub repositories.
*
* @see GitHub stargazer
* API documentation
* @since 4.2
*/
public class StargazerService extends GitHubService {
/**
* Create stargazer service
*/
public StargazerService() {
super();
}
/**
* Create stargazer service
*
* @param client
*/
public StargazerService(GitHubClient client) {
super(client);
}
/**
* Create page stargazer request
*
* @param repository
* @param start
* @param size
* @return request
*/
protected PagedRequest createStargazerRequest(
IRepositoryIdProvider repository, int start, int size) {
String id = getId(repository);
PagedRequest request = createPagedRequest(start, size);
StringBuilder uri = new StringBuilder(SEGMENT_REPOS);
uri.append('/').append(id);
uri.append(SEGMENT_STARGAZERS);
request.setUri(uri);
request.setType(new TypeToken>() {
}.getType());
return request;
}
/**
* Get users starring the given repository
*
* @param repository
* @return non-null but possibly empty list of users
* @throws IOException
*/
public List getStargazers(IRepositoryIdProvider repository)
throws IOException {
PagedRequest request = createStargazerRequest(repository,
PAGE_FIRST, PAGE_SIZE);
return getAll(request);
}
/**
* Page stargazers of given repository
*
* @param repository
* @return page iterator
*/
public PageIterator pageStargazers(IRepositoryIdProvider repository) {
return pageStargazers(repository, PAGE_SIZE);
}
/**
* Page stargazers of given repository
*
* @param repository
* @param size
* @return page iterator
*/
public PageIterator pageStargazers(IRepositoryIdProvider repository,
int size) {
return pageStargazers(repository, PAGE_FIRST, size);
}
/**
* Page stargazers of given repository
*
* @param repository
* @param start
* @param size
* @return page iterator
*/
public PageIterator pageStargazers(IRepositoryIdProvider repository,
int start, int size) {
PagedRequest request = createStargazerRequest(repository, start,
size);
return createPageIterator(request);
}
/**
* Create page starred request
*
* @param user
* @param start
* @param size
* @return request
*/
protected PagedRequest createStarredRequest(String user,
int start, int size) {
if (user == null)
throw new IllegalArgumentException("User cannot be null"); //$NON-NLS-1$
if (user.length() == 0)
throw new IllegalArgumentException("User cannot be empty"); //$NON-NLS-1$
PagedRequest request = createPagedRequest(start, size);
StringBuilder uri = new StringBuilder(SEGMENT_USERS);
uri.append('/').append(user);
uri.append(SEGMENT_STARRED);
request.setUri(uri);
request.setType(new TypeToken>() {
}.getType());
return request;
}
/**
* Create page starred request
*
* @param start
* @param size
* @return request
*/
protected PagedRequest createStarredRequest(int start, int size) {
PagedRequest request = createPagedRequest(start, size);
request.setUri(SEGMENT_USER + SEGMENT_STARRED);
request.setType(new TypeToken>() {
}.getType());
return request;
}
/**
* Get repositories starred by the given user
*
* @param user
* @return non-null but possibly empty list of repositories
* @throws IOException
*/
public List getStarred(String user) throws IOException {
PagedRequest request = createStarredRequest(user,
PAGE_FIRST, PAGE_SIZE);
return getAll(request);
}
/**
* Page repositories starred by given user
*
* @param user
* @return page iterator
* @throws IOException
*/
public PageIterator pageStarred(String user) throws IOException {
return pageStarred(user, PAGE_SIZE);
}
/**
* Page repositories starred by given user
*
* @param user
* @param size
* @return page iterator
* @throws IOException
*/
public PageIterator pageStarred(String user, int size)
throws IOException {
return pageStarred(user, PAGE_FIRST, size);
}
/**
* Page repositories starred by given user
*
* @param user
* @param start
* @param size
* @return page iterator
* @throws IOException
*/
public PageIterator pageStarred(String user, int start, int size)
throws IOException {
PagedRequest request = createStarredRequest(user, start,
size);
return createPageIterator(request);
}
/**
* Get repositories starred by the currently authenticated user
*
* @return non-null but possibly empty list of repositories
* @throws IOException
*/
public List getStarred() throws IOException {
PagedRequest request = createStarredRequest(PAGE_FIRST,
PAGE_SIZE);
return getAll(request);
}
/**
* Page repositories starred by the currently authenticated user
*
* @return page iterator
* @throws IOException
*/
public PageIterator pageStarred() throws IOException {
return pageStarred(PAGE_SIZE);
}
/**
* Page repositories starred by the currently authenticated user
*
* @param size
* @return page iterator
* @throws IOException
*/
public PageIterator pageStarred(int size) throws IOException {
return pageStarred(PAGE_FIRST, size);
}
/**
* Page repositories starred by the currently authenticated user
*
* @param start
* @param size
* @return page iterator
* @throws IOException
*/
public PageIterator pageStarred(int start, int size)
throws IOException {
PagedRequest request = createStarredRequest(start, size);
return createPageIterator(request);
}
/**
* Is currently authenticated user starring given repository?
*
* @param repository
* @return {@code true} if starred, {@code false} otherwise
* @throws IOException
*/
public boolean isStarring(IRepositoryIdProvider repository)
throws IOException {
String id = getId(repository);
StringBuilder uri = new StringBuilder(SEGMENT_USER);
uri.append(SEGMENT_STARRED);
uri.append('/').append(id);
return check(uri.toString());
}
/**
* Add currently authenticated user as a stargazer of the given repository
*
* @param repository
* @throws IOException
*/
public void star(IRepositoryIdProvider repository) throws IOException {
String id = getId(repository);
StringBuilder uri = new StringBuilder(SEGMENT_USER);
uri.append(SEGMENT_STARRED);
uri.append('/').append(id);
client.put(uri.toString());
}
/**
* Remove currently authenticated user as a stargazer of the given repository
*
* @param repository
* @throws IOException
*/
public void unstar(IRepositoryIdProvider repository) throws IOException {
String id = getId(repository);
StringBuilder uri = new StringBuilder(SEGMENT_USER);
uri.append(SEGMENT_STARRED);
uri.append('/').append(id);
client.delete(uri.toString());
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy