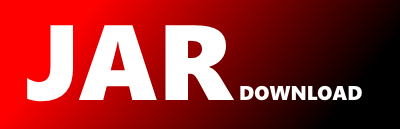
com.jeesuite.cache.local.GuavaLevel1CacheProvider Maven / Gradle / Ivy
/**
*
*/
package com.jeesuite.cache.local;
import java.io.IOException;
import java.util.Map;
import java.util.concurrent.Callable;
import java.util.concurrent.ConcurrentHashMap;
import java.util.concurrent.TimeUnit;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.google.common.cache.Cache;
import com.google.common.cache.CacheBuilder;
/**
* 本地缓存服务
* @description
* @author vakin
* @date 2016年6月1日
*/
public class GuavaLevel1CacheProvider implements Level1CacheProvider{
private static final String _NULL = "NULL";
private static final Logger logger = LoggerFactory.getLogger(GuavaLevel1CacheProvider.class);
private Map> caches = new ConcurrentHashMap>();
private int maxSize = 10000;
private int timeToLiveSeconds = 600;
public void setMaxSize(int maxSize) {
this.maxSize = maxSize;
}
public void setTimeToLiveSeconds(int timeToLiveSeconds) {
this.timeToLiveSeconds = timeToLiveSeconds;
}
@Override
public void start() {
}
public boolean set(String cacheName,String key,Object value){
if(value == null)return true;
Cache cache = getCacheHolder(cacheName);
if(cache != null){
cache.put(key, value);
}
return true;
}
@SuppressWarnings("unchecked")
public T get(String cacheName,String key){
try {
Cache cache = getCacheHolder(cacheName);
if(cache != null){
Object result = cache.get(key, new Callable
© 2015 - 2025 Weber Informatics LLC | Privacy Policy