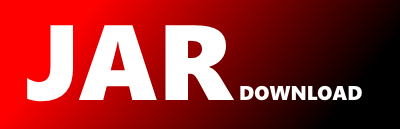
com.jeesuite.common2.excel.ExcelWriter Maven / Gradle / Ivy
The newest version!
package com.jeesuite.common2.excel;
import java.io.Closeable;
import java.io.File;
import java.io.FileOutputStream;
import java.io.IOException;
import java.io.OutputStream;
import java.math.BigDecimal;
import java.util.List;
import org.apache.commons.lang3.StringUtils;
import org.apache.poi.openxml4j.exceptions.InvalidFormatException;
import org.apache.poi.ss.usermodel.BorderStyle;
import org.apache.poi.ss.usermodel.Cell;
import org.apache.poi.ss.usermodel.CellStyle;
import org.apache.poi.ss.usermodel.FillPatternType;
import org.apache.poi.ss.usermodel.Font;
import org.apache.poi.ss.usermodel.HorizontalAlignment;
import org.apache.poi.ss.usermodel.IndexedColors;
import org.apache.poi.ss.usermodel.Row;
import org.apache.poi.ss.usermodel.Sheet;
import org.apache.poi.ss.usermodel.VerticalAlignment;
import org.apache.poi.ss.util.CellRangeAddress;
import org.apache.poi.xssf.streaming.SXSSFWorkbook;
import org.apache.poi.xssf.usermodel.XSSFWorkbook;
import org.slf4j.Logger;
import org.slf4j.LoggerFactory;
import com.jeesuite.common2.excel.helper.ExcelBeanHelper;
import com.jeesuite.common2.excel.model.ExcelMeta;
import com.jeesuite.common2.excel.model.TitleMeta;
public final class ExcelWriter implements Closeable {
private static final Logger LOG = LoggerFactory.getLogger(ExcelWriter.class);
private String sheetName;
private OutputStream outputStream;
private final SXSSFWorkbook workbook;
/**
* 构造方法,传入需要操作的excel文件路径
*
* @param excelFilePath 需要操作的excel文件的路径
* @throws IOException IO流异常
* @throws InvalidFormatException 非法的格式异常
*/
public ExcelWriter(String excelFilePath,String sheetName) throws IOException, InvalidFormatException {
this.sheetName = sheetName;
File file = new File(excelFilePath);
boolean exists = file.exists();
if(!exists)file.createNewFile();
outputStream = new FileOutputStream(file);
this.workbook = createWorkbook(exists ? file : null);
}
public ExcelWriter(String excelFilePath) throws IOException, InvalidFormatException {
this(excelFilePath,"Sheet1");
}
public ExcelWriter(OutputStream outputStream) throws IOException, InvalidFormatException {
this(outputStream,"Sheet1");
}
public ExcelWriter(OutputStream outputStream, String sheetName) throws InvalidFormatException, IOException {
super();
this.outputStream = outputStream;
this.sheetName = sheetName;
this.workbook = createWorkbook(null);
}
/**
* 设置需要读取的sheet名字,不设置默认的名字是Sheet1,也就是excel默认给的名字,所以如果文件没有自已修改,这个方法也就不用调了
*
* @param sheetName 需要读取的Sheet名字
*/
public void setSheetName(String sheetName) {
this.sheetName = sheetName;
}
private SXSSFWorkbook createWorkbook(File existFile) throws IOException, InvalidFormatException {
SXSSFWorkbook workbook;
if (existFile == null) {
workbook = new SXSSFWorkbook(1000);//内存中保留 1000条数据,以免内存溢出
} else {
workbook = new SXSSFWorkbook(new XSSFWorkbook(existFile), 1000);
}
return workbook;
}
/**
* 将数据写入excel文件
*
* @param list 数据列表
* @param 泛型
* @return 写入结果
*/
public boolean write(List list, Class clazz) {
ExcelMeta excelMeta = ExcelBeanHelper.getExcelMeta(clazz);
try {
Sheet sheet = workbook.createSheet(this.sheetName);
sheet.setDefaultColumnWidth(15);
CellStyle titleStyle = workbook.createCellStyle();
titleStyle.setAlignment(HorizontalAlignment.CENTER);
titleStyle.setVerticalAlignment(VerticalAlignment.CENTER);
titleStyle.setFillForegroundColor(IndexedColors.GREY_40_PERCENT.getIndex());
titleStyle.setFillPattern(FillPatternType.SOLID_FOREGROUND);
titleStyle.setBorderBottom(BorderStyle.THIN); //下边框
titleStyle.setBorderLeft(BorderStyle.THIN);
Font font = workbook.createFont();
font.setFontName("宋体");
font.setFontHeightInPoints((short) 13);
titleStyle.setFont(font);
//列值类型
Class>[] cellValueTypes = new Class>[excelMeta.getTitleColumnNum()];
//写标题
for (int i = 1; i <= excelMeta.getTitleRowNum(); i++) {
Row excelRow = sheet.createRow(i - 1);
for (int j = 1; j <= excelMeta.getTitleColumnNum(); j++) {
TitleMeta titleMeta = excelMeta.getTitleMeta(i, j);
Cell cell = excelRow.createCell(j - 1);
cell.setCellValue(titleMeta == null ? "" : titleMeta.getTitle());
cell.setCellStyle(titleStyle);
cellValueTypes[j-1] = titleMeta.getValueType();
}
}
//合并表头
//sheet.addMergedRegion(new CellRangeAddress(0, 0, 3, 8));
//sheet.addMergedRegion(new CellRangeAddress(0, 1, 0, 0));
mergeColumns(sheet,titleStyle);
mergeRows(sheet,titleStyle,excelMeta);
// 行数
int rowsCount = sheet.getPhysicalNumberOfRows();
//冻结表头
//sheet.createFreezePane(0, rowsCount - 1);
// 写入内容
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy