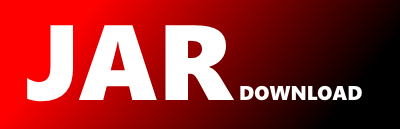
com.jelastic.api.Response Maven / Gradle / Ivy
The newest version!
package com.jelastic.api;
import org.json.JSONException;
import org.json.JSONObject;
import java.lang.reflect.Constructor;
import java.lang.reflect.Field;
import java.lang.reflect.Method;
import java.net.URL;
import java.security.*;
import java.security.cert.Certificate;
import java.util.Iterator;
/**
* @author Ruslan Sinitskiy
*/
public class Response implements ResponseInterface {
public static final int OK = 0;
public static final int SERVICE_NOT_AVAILABLE = 1;
public static final int ACCESS_DENIED = 2;
public static final int INVALID_PARAM = 3;
public static final int ABORT = 4;
public static final int SERVICE_NOT_FOUND = 5;
public static final int METHOD_NOT_FOUND = 6;
public static final int NOT_AUTHENTICATED = 7;
public static final int PERMISSION_DENIED = 8;
public static final int OPERATION_NOT_ALLOWED = 9;
public static final int APPLICATION_IS_BLOCKED = 10;
public static final int APPLICATION_NOT_EXIST = 11;
public static final int INVALID_CHECKSUM = 12;
public static final int SYSTEM_ERROR = 13;
public static final int APPLICATION_NOT_PRESENT_IN_CLUSTER_NODE = 14;
public static final int SYSTEM_APPLICATION_IS_NOT_PERMITTED = 15;
public static final int APPLICATION_NOT_LOADED = 16;
public static final int INVALID_PARAM_NOTICE = 22;
public static final int IO_ERROR = 17;
public static final int DEPRECATED = 18;
public static final int EXEC_TIMEOUT = 19;
public static final int ERROR_UNKNOWN = 99;
public static final int ERROR_STORAGE_RESPONSE = 301;
public static final int ERROR_STORAGE_CONNECTION = 302;
public static final int ERROR_STORAGE_IO = 303;
public static final int ERROR_STORAGE_UNAVAILABLE = 304;
public static final int ERROR_STORAGE_REQUEST = 305;
public static final int ERROR_FILE_MAX_SIZE = 401;
public static final int ERROR_FILE_NOT_ALLOWED = 402;
public static final int ERROR_FILE_NOT_SPECIFIED = 403;
public static final int EMAIL_INVALID_FORMAT = 501;
public static final int EMAIL_IS_REGISTERED = 502;
public static final int EMAIL_NOT_EXIST = 503;
public static final int PASSWORD_INVALID_FORMAT = 504;
public static final int INVALID_TOKEN = 505;
public static final int EMAIL_NOT_REGISTERED = 506;
public static final int VALUE_IS_TOO_LONG = 507;
public static final int ERROR_EMAIL_TRANSPORT = 601;
public static final int AUTHENTICATION_FAILED = 701;
public static final int USER_NOT_AUTHENTICATED = 702;
public static final int USER_NOT_EXIST = 703;
public static final int USER_IS_BLOCKED = 704;
public static final int USER_ACCESS_DENIED = 705;
public static final int USER_ALREADY_ACTIVATED = 706;
public static final int USER_ID_NOT_EXIT = 707;
public static final int USER_NOT_ACTIVATED = 708;
public static final int USER_PASSWORD_DOES_NOT_MATCH = 709;
public static final int USER_ALREADY_RELATED = 710;
public static final int USER_NOT_RELATED = 711;
public static final int PASSWORD_INVALID = 801;
public static final int TARGET_APPLICATION_NOT_EXIST = 901;
public static final int APPLICATION_FIELD_NOT_EXIST = 902;
public static final int APPLICATION_RIGHTS_NOT_EXIST = 903;
public static final int QUOTA_APPLICATIONS_COUNT_MAX = 904;
public static final int APPLICATIONS_POOL_IS_EXIST = 905;
public static final int APPLICATIONS_POOL_NOT_EXIST = 906;
public static final int APPLICATION_CONFIG_FORMAT_ERROR = 907;
public static final int APPLICATION_EXPORT_DB_SIZE_LIMIT = 908;
public static final int APPLICATION_EXPORT_DB_UPLOAD_ERROR = 909;
public static final int APPLICATION_EXPORT_RESOURCES_UPLOAD_ERROR = 910;
public static final int APPLICATION_IMPORT_DB_ARCHIVE_ERROR = 911;
public static final int APPLICATION_EXPORT_RESOURCES_SIZE_LIMIT = 912;
public static final int SOLUTION_ALREADY_EXIST = 913;
public static final int SOLUTION_NOT_EXIST = 914;
public static final int SOLUTION_FIELD_NOT_EXIST = 915;
public static final int SOLUTION_NOT_CLONABLE = 916;
public static final int APPLICATION_ALREADY_EXIST = 917;
public static final int APPLICATION_WRONG_TRANSFER_STATE = 918;
public static final int TYPE_NAME_INVALID = 1001;
public static final int TYPE_IS_EXIST = 1002;
public static final int TYPE_NOT_EXIST = 1003;
public static final int TYPE_NOT_DEFINED = 1004;
public static final int FIELD_IS_EXIST = 1005;
public static final int FIELD_NOT_EXIST = 1006;
public static final int FIELD_NAME_INVALID = 1007;
public static final int FIELD_NAME_IS_RESERVED = 1008;
public static final int UNIQUE_FORMAT_INVALID = 1009;
public static final int TYPE_IS_RESERVED = 1010;
public static final int QUOTA_TYPES_COUNT_MAX = 1011;
public static final int QUOTA_FIELDS_COUNT_MAX = 1012;
public static final int TYPE_LENGTH_MAX = 1013;
//public static final int TYPE_CANNOT_CONTAIN_HIMSELF = 1014;
public static final int OBJECT_NOT_EXIST = 1101;
public static final int OBJECT_FORMAT_ERROR = 1102;
public static final int OBJECT_ASSOCIATION_NOT_EXIST = 1103;
public static final int OBJECT_DUPLICATE_ENTRY = 1104;
public static final int PROPERTY_NOT_EXIST = 1201;
public static final int PROPERTY_NAME_INVALID = 1202;
public static final int PROPERTY_READ_ONLY = 1203;
public static final int CRITERIA_FORMAT_INVALID = 1301;
public static final int ACCESS_SUBJECT_NOT_EXIST = 1401;
public static final int ACCESS_OBJECT_NOT_EXIST = 1402;
public static final int ACCESS_RIGHTS_NOT_EXIST = 1403;
public static final int ACCESS_PRIORITY_NOT_EXIST = 1404;
public static final int ACCESS_RIGHTS_NOT_DEFINED = 1405;
public static final int ACCESS_ROLE_NOT_EXIST = 1406;
public static final int ACCESS_ROLE_IS_EXIST = 1407;
public static final int ACCESS_ROLE_IS_NOT_APPLIED = 1408;
public static final int ACCESS_RIGHTS_IS_NOT_APPLIED = 1409;
public static final int ACCESS_ROLE_TYPE_IS_NOT_DEFINED = 1410;
public static final int REQUEST_FORMAT_INVALID = 1501;
public static final int METHOD_FORMAT_INVALID = 1502;
public static final int QUOTA_METHODS_COUNT_MAX = 1601;
public static final int SCRIPT_TYPE_NOT_SUPPORTED = 1701;
public static final int SCRIPT_NOT_FOUND = 1702;
public static final int SCRIPT_FIELD_NOT_DEFINED = 1703;
public static final int SCRIPT_EVAL_EXCEPTION = 1704;
public static final int SCRIPT_EVAL_TIMEOUT = 1705;
public static final int SCRIPT_EVAL_DISABLED_IN_NODE = 1706;
public static final int SCRIPT_IO_EXCEPTION = 1707;
public static final int SCRIPT_IMPORT_EXCEPTION = 1708;
public static final int SCRIPT_ONLY_LOCAL_EVAL_ALLOWED = 1709;
public static final int SCRIPT_ALREADY_EXIST = 1710;
public static final int SCRIPT_ANNOTATION_NOT_SUPPORTED = 1711;
public static final int SCRIPT_ANNOTATION_SYNTAX_ERROR = 1712;
public static final int SCRIPT_ANNOTATION_VALUE_NOT_SUPPORTED = 1713;
public static final int SCRIPT_COMPILE_TIMEOUT = 1714;
public static final int SCRIPT_ANNOTATION_USAGE_NOT_ALLOWED = 1715;
public static final int SCHEDULER_TRIGGER_UNKNOWN_FORMAT = 1801;
public static final int SCHEDULER_TASK_NOT_FOUND = 1802;
public static final int SCHEDULER_PARAMS_INVALID_FORMAT = 1803;
public static final int SYSTEM_USER_GROUP_NOT_EXIST = 1901;
public static final int FILE_PATH_ACCESS_DENIED = 2001;
public static final int FILE_PATH_NOT_EXIST = 2002;
public static final int FILE_PATH_IS_EXIST = 2003;
public static final int FILE_PATH_NOT_DIRECTORY = 2004;
public static final int FILE_PATH_IS_DIRECTORY = 2005;
public static final int FILE_MAX_LENGTH_LIMIT = 2006;
public static final int FILE_NAME_SYNTAX_ERROR = 2007;
public static final int SYSTEM_EVENT_UNKNOWN = 2101;
public static final int SYSTEM_EVENT_ERROR_EXECUTE = 2102;
public static final int REDIRECT_CLUSTER_NODE_EXCEPTION = 2201;
public static final int NODE_MANAGER_ALL_HARDWARE_NODES_ARE_BUSY = 2202;
public static final int NODE_MANAGER_ALL_IPS_ARE_BUSY = 2203;
public static final int POOL_MANAGER_FAILED_TO_GET_IP_ADDRESS = 2204;
public static final int POOL_MANAGER_FAILED_TO_GET_NODE = 2205;
public static final int CONTEXT_NAME_INVALID = 2301;
// BILLING
public static final int SERVICE_PLAN_NOT_FOUND = 2206;
public static final int EXTERN_BILLING_SYSTEM = 2207;
public static final int NEGATIVE_PRICE = 2208;
public static final int SERVICE_PLAN_ALREADY_EXIST = 2209;
public static final int HIBERNATE_EXCEPTION = 2210; // DB
public static final int SERVICE_PLAN_IS_USED = 2211; // cannot be deleted from db foreign references
public static final int ILLEGAL_STATE = 2212;
public static final int NOT_SUPPORTED = 2213;
public static final int EXTERN_BILLING_FRAUD_CHECK_REQUIRED = 2222;
// error message contains text for the end user.
// display it.
public static final int INVALID_USER_INPUT = 2223;
public static final int REQUEST_ABORTED = 2224;
// SSL
public static final int SSL_CERTIFICATE_NOT_VALID = 3001;
public static final int VCS_PROJECT_NOT_EXIST = 2500;
public static final int VCS_PROJECT_ALREADY_EXIST = 2501;
public static final int VCS_TYPE_NOT_SUPPORT = 2502;
public static final int POOL_MANAGER_POOL_IP_NOT_FOUND = 2700;
public static final int POOL_MANAGER_NO_FREE_IP = 2701;
public static final int POOL_MANAGER_POOL_IP_NOW_BUSY = 2702;
//JEM
public static final int JEM_AUTHENTICATION_FAILED = 4000;
public static final int JEM_ERROR_READ_CONFIG = 4001;
public static final int JEM_NO_FREE_DISK_SPACE = 4075;
public static final int JEM_ERROR_UNKNOWN = 4099;
//Virtualization Layer
public static final int VL_DISK_QUOTA_EXCEEDED = 5000;
public static final int VL_EMPTY_OS_NODE_POOL = 5001;
public static final int VL_MANAGER = 5002;
public static final int ENVIRONMENT_MIGRATION_IS_NOT_POSSIBLE = 5003;
//SSH access to container
public static final int SSH_KEY_INVALID = 6000;
public static final int SSH_KEY_NOT_UNIQUE_FINGERPRINT = 6001;
public static final int SSH_KEY_NOT_UNIQUE_TITLE = 6002;
public static final int SSH_KEY_TOO_LONG = 6003;
public static final int SSH_KEY_TOO_LONG_TITLE = 6004;
public static final int SSH_ACCESS_DISABLED = 6010;
public static final int SSH_KEY_GENERATION_FAIL = 6020;
public static final int NODE_CREDENTIALS_ABSENT = 6030;
public static final int LABEL_VALUE_NOT_VALID = 8301;
public static final int LABEL_NAME_NOT_VALID = 8300;
public static String SOURCE_OF_RESPONSE = "hx-core";
protected int result = -1;
protected int reason = -1;
protected String error = null;
protected String source = SOURCE_OF_RESPONSE;
private String raw = null;
private static final Response OK_RESPONSE = new Response(Response.OK);
public int getResult() {
return result;
}
public void setResult(int result) {
this.result = result;
}
public int getReason() {
return reason;
}
public void setReason(int reason) {
this.reason = reason;
}
public String getError() {
return error;
}
public void setError(String error) {
this.error = error;
}
public String getSource() {
return source;
}
public void setSource(String source) {
this.source = source;
}
public String getRaw() {
return raw;
}
public void setRaw(String raw) {
this.raw = raw;
}
public Response() {
}
public Response(int result) {
this.result = result;
}
public Response(int result, String error) {
this.result = result;
this.error = error;
}
public Response(int result, String error, int reason) {
this.result = result;
this.error = error;
this.reason = reason;
}
public Response(int result, String error, String source) {
this(result, error);
this.source = source;
}
public Response(Response response) {
this(response.getResult(), response.getError());
}
public boolean isOK() {
return getResult() == OK;
}
public Object fromString(String str) {
try {
return fromJSON(new JSONObject(str));
} catch (JSONException ex) {
// System.out.println("WARNING: JSONException ---------------");
// System.out.println(str);
// System.out.println("--------------------------------------");
// ex.printStackTrace();
setError(ex.toString() + " --> " + str.substring(0, Math.min(str.length(), 500)) + "...");
setResult(ERROR_UNKNOWN);
return this;
}
}
protected Response _fromJSON(JSONObject json) throws JSONException {
Class clazz = this.getClass();
if (json.has("result")) {
result = json.getInt("result");
}
if (json.has("reason")) {
reason = json.getInt("reason");
}
if (json.has("error")) {
error = json.getString("error");
}
if (json.has("source")) {
source = json.getString("source");
}
if (json.has("raw")) {
raw = json.getString("raw");
}
for (Method m : clazz.getDeclaredMethods()) {
String name = m.getName();
if (name.equalsIgnoreCase("fromJSON") || name.equalsIgnoreCase("_fromJSON")) {
return this;
}
}
/**
* ниже следующий код выполнится только если у класа потомка не определн свой метод fromJSON или _fromJSON
*/
Iterator keys = json.keys();
while (keys.hasNext()) {
String key = keys.next();
try {
Field field = clazz.getDeclaredField(key);
Object value = json.get(key);
field.setAccessible(true);
field.set(this, value);
} catch (IllegalAccessException ex2) {
ex2.printStackTrace();
} catch (NoSuchFieldException ex2) {
continue;
}
}
return this;
}
public Response fromJSON(JSONObject json) {
try {
return _fromJSON(json);
} catch (JSONException ex) {
ex.printStackTrace();
error = ex.toString();
result = ERROR_UNKNOWN;
}
return this;
}
protected JSONObject _toJSON() throws JSONException {
JSONObject json = new JSONObject();
if (getResult() > -1) {
json.put("result", getResult());
}
if (getReason() > -1) {
json.put("reason", getReason());
}
if (getError() != null) {
json.put("error", getError());
}
if (!isOK() && getSource() != null) {
json.put("source", getSource());
}
if (getRaw() != null) {
json.put("raw", getRaw());
}
return json;
}
public JSONObject toJSON() {
JSONObject json = null;
try {
json = _toJSON();
} catch (Exception ex) {
ex.printStackTrace();
try {
json = new JSONObject().put("result", ERROR_UNKNOWN).put("error", ex.toString());
} catch (JSONException exc) {
}
}
return json;
}
@Override
public String toString() {
return toJSON().toString();
}
public Object get(final String name) {
Permissions perms = new Permissions();
perms.add(new AllPermission());
ProtectionDomain domain = new ProtectionDomain(new CodeSource((URL) null, (Certificate[]) null), perms);
AccessControlContext relaxedAcc = new AccessControlContext(new ProtectionDomain[]{domain});
final Class clazz = getClass();
final Object _this = this;
return AccessController.doPrivileged(new PrivilegedAction
© 2015 - 2025 Weber Informatics LLC | Privacy Policy