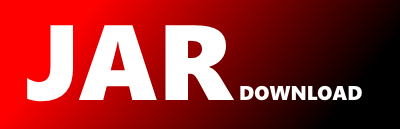
com.jelastic.api.billing.response.AccountResponse Maven / Gradle / Ivy
/*Server class MD5: 127675d8064f0d685b1d35bd6b54420c*/
package com.jelastic.api.billing.response;
import com.jelastic.api.Response;
import com.jelastic.api.core.utils.DateUtils;
import com.jelastic.api.system.persistence.Account;
import com.jelastic.api.system.persistence.AccountStatus;
import com.jelastic.api.system.persistence.GroupPricingModel;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.math.BigDecimal;
import java.text.ParseException;
import java.util.Date;
import java.util.HashSet;
import java.util.Set;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class AccountResponse extends Response {
public static final String UID = "uid";
public static final String BALANCE = "balance";
public static final String CREDIT = "credit";
public static final String DEBIT = "debit";
public static final String STATUS = "status";
public static final String GROUP = "group";
public static final String BONUS = "bonus";
public static final String EMAIL = "email";
public static final String CREATED_ON = "createdOn";
public static final String UPDATED_GROUP_ON = "updatedGroupOn";
public static final String UPDATED_STATUS_ON = "updatedStatusOn";
public static final String GROUP_WIN_DOMAIN = "groupWinDomain";
public static final String IS_COMMERIAL = "isCommerial";
public static final String IS_ENABLED = "isEnabled";
public static final String GROUP_TYPE = "groupType";
public static final String IS_REGISTERED = "isRegistered";
public static final String HARD_NODE_GROUPS = "hardNodeGroups";
public static final String DEFAULT_HN_GROUP = "defaultHardNodeGroup";
private static final String PREVIOUS_UPDATE_GROUP_ON = "previousUpdatedGroupOn";
private static final String LANGUAGE = "language";
private static final String EBS_USER_ID = "ebsUserId";
private static final String DEFAULT_PAYMENT_METHOD_ID = "defaultPayMethodId";
private Integer uid;
private BigDecimal balance;
private BigDecimal credit;
private BigDecimal debit;
private AccountStatus status;
private Date createdOn;
private Date updatedGroupOn;
private Date updatedStatusOn;
private String group;
private Integer groupWinDomain;
private BigDecimal bonus;
private String email;
private Boolean isEnabled;
private Boolean isCommerial;
private String groupType;
private Boolean isRegistered;
private Set hardNodeGroups = new HashSet();
private String defaultHardNodeGroup;
private Date previousUpdatedGroupOn;
private String language;
private String ebsUserId;
private String defaultPayMethodId;
public AccountResponse() {
super(OK);
}
public AccountResponse(Integer uid) {
this();
this.uid = uid;
}
public AccountResponse(int result, String error) {
super(result, error);
}
public AccountResponse(int result, String error, int uid) {
super(result, error);
this.uid = uid;
}
public AccountResponse(Account account) {
this(account, false);
}
public AccountResponse(Account account, boolean isFullInfo) {
this();
this.uid = account.getUid();
this.balance = account.getBalance();
this.status = account.getStatus();
this.createdOn = account.getCreatedOn();
this.updatedGroupOn = account.getUpdatedGroupOn();
this.updatedStatusOn = account.getUpdatedStatusOn();
this.group = account.getGroup().getName();
this.groupWinDomain = account.getGroup().getWinDomainId();
this.bonus = account.getBonus();
this.email = account.getEmail();
this.isEnabled = account.getEnabled();
this.isCommerial = account.getGroup().getType().isCommercial();
this.groupType = account.getGroup().getType().toString();
this.isRegistered = account.isRegistered();
for (GroupPricingModel groupPricingModel : account.getGroup().getGroupPricingModels()) {
if (groupPricingModel.isEnabled()) {
this.hardNodeGroups.add(groupPricingModel.getHardNodeGroup());
}
if (groupPricingModel.isDefault()) {
this.defaultHardNodeGroup = groupPricingModel.getHardNodeGroup();
}
}
this.ebsUserId = account.getEbsUserIdAsStringNullable();
if (isFullInfo) {
this.previousUpdatedGroupOn = account.getPreviousUpdatedGroupOn();
this.language = account.getLanguage();
this.defaultPayMethodId = account.getDefaultPayMethodIdStr();
}
}
public AccountResponse(Integer uid, BigDecimal credit, BigDecimal debit) {
this();
this.uid = uid;
this.credit = credit;
this.debit = debit;
}
public Integer getGroupWinDomain() {
return groupWinDomain;
}
public void setGroupWinDomain(Integer groupWinDomain) {
this.groupWinDomain = groupWinDomain;
}
public Boolean isCommerial() {
return isCommerial;
}
public void setCommerial(Boolean commerial) {
isCommerial = commerial;
}
public BigDecimal getBalance() {
return balance;
}
public void setBalance(BigDecimal balance) {
this.balance = balance;
}
public Date getCreatedOn() {
return createdOn;
}
public void setCreatedOn(Date createdOn) {
this.createdOn = createdOn;
}
public Date getUpdatedGroupOn() {
return updatedGroupOn;
}
public void setUpdatedGroupOn(Date updatedGroupOn) {
this.updatedGroupOn = updatedGroupOn;
}
public BigDecimal getCredit() {
return credit;
}
public void setCredit(BigDecimal credit) {
this.credit = credit;
}
public AccountStatus getStatus() {
return status;
}
public void setStatus(AccountStatus status) {
this.status = status;
}
public Integer getUid() {
return uid;
}
public void setUid(Integer uid) {
this.uid = uid;
}
public BigDecimal getDebit() {
return debit;
}
public void setDebit(BigDecimal debit) {
this.debit = debit;
}
public String getGroup() {
return group;
}
public void setGroup(String group) {
this.group = group;
}
public BigDecimal getBonus() {
return bonus;
}
public void setBonus(BigDecimal bonus) {
this.bonus = bonus;
}
public String getEmail() {
return email;
}
public void setEmail(String email) {
this.email = email;
}
public Boolean getEnabled() {
return isEnabled;
}
public void setEnabled(Boolean enabled) {
isEnabled = enabled;
}
public String getGroupType() {
return groupType;
}
public void setGroupType(String groupType) {
this.groupType = groupType;
}
public Boolean isRegistered() {
return isRegistered;
}
public void setRegistered(Boolean isRegistered) {
this.isRegistered = isRegistered;
}
public Set getHardNodeGroups() {
return hardNodeGroups;
}
public void setHardNodeGroups(Set hardNodeGroups) {
this.hardNodeGroups = hardNodeGroups;
}
public String getDefaultHardNodeGroup() {
return defaultHardNodeGroup;
}
public void setDefaultHardNodeGroup(String defaultHardNodeGroup) {
this.defaultHardNodeGroup = defaultHardNodeGroup;
}
public Date getUpdatedStatusOn() {
return updatedStatusOn;
}
public void setUpdatedStatusOn(Date updatedStatusOn) {
this.updatedStatusOn = updatedStatusOn;
}
public Boolean getIsEnabled() {
return isEnabled;
}
public void setIsEnabled(Boolean isEnabled) {
this.isEnabled = isEnabled;
}
public Date getPreviousUpdatedGroupOn() {
return previousUpdatedGroupOn;
}
public void setPreviousUpdatedGroupOn(Date previousUpdatedGroupOn) {
this.previousUpdatedGroupOn = previousUpdatedGroupOn;
}
public String getLanguage() {
return language;
}
public void setLanguage(String language) {
this.language = language;
}
public String getEbsUserId() {
return ebsUserId;
}
public void setEbsUserId(String ebsUserId) {
this.ebsUserId = ebsUserId;
}
public Integer getDefaultPayMethodId() {
return Integer.parseInt(defaultPayMethodId);
}
public String getDefaultPayMethodIdStr() {
return defaultPayMethodId;
}
public void setDefaultPayMethodId(Integer defaultPayMethodId) {
this.defaultPayMethodId = Integer.toString(defaultPayMethodId);
}
public void setDefaultPayMethodId(String defaultPayMethodId) {
this.defaultPayMethodId = defaultPayMethodId;
}
@Override
public JSONObject _toJSON() throws JSONException {
JSONObject json = super._toJSON();
json.put(UID, this.uid);
json.put(BALANCE, this.balance);
json.put(CREDIT, this.credit);
json.put(DEBIT, this.debit);
json.put(IS_COMMERIAL, this.isCommerial);
json.put(IS_REGISTERED, this.isRegistered);
if (this.status != null) {
json.put(STATUS, this.status.getValue());
}
if (this.createdOn != null) {
json.put(CREATED_ON, DateUtils.formatSqlDateTime(this.createdOn));
}
if (this.updatedGroupOn != null) {
json.put(UPDATED_GROUP_ON, DateUtils.formatSqlDateTime(this.updatedGroupOn));
}
if (this.updatedStatusOn != null) {
json.put(UPDATED_STATUS_ON, DateUtils.formatSqlDateTime(this.updatedStatusOn));
}
json.put(GROUP, group);
if (groupWinDomain != null) {
json.put(GROUP_WIN_DOMAIN, groupWinDomain);
}
json.put(BONUS, bonus);
json.put(EMAIL, email);
if (isEnabled != null) {
json.put(IS_ENABLED, isEnabled ? 1 : 0);
}
json.put(GROUP_TYPE, groupType);
json.put(HARD_NODE_GROUPS, new JSONArray(hardNodeGroups));
json.put(DEFAULT_HN_GROUP, defaultHardNodeGroup);
json.putOpt(PREVIOUS_UPDATE_GROUP_ON, previousUpdatedGroupOn);
json.putOpt(LANGUAGE, language);
json.putOpt(EBS_USER_ID, ebsUserId);
json.putOpt(DEFAULT_PAYMENT_METHOD_ID, defaultPayMethodId);
return json;
}
@Override
public AccountResponse _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
if (json.has(UID)) {
this.uid = json.getInt(UID);
}
if (json.has(IS_COMMERIAL)) {
this.isCommerial = json.getBoolean(IS_COMMERIAL);
}
if (json.has(IS_REGISTERED)) {
this.isRegistered = json.getBoolean(IS_REGISTERED);
}
if (json.has(BALANCE)) {
this.balance = BigDecimal.valueOf(json.getDouble(BALANCE));
}
if (json.has(CREDIT)) {
this.credit = BigDecimal.valueOf(json.getDouble(CREDIT));
}
if (json.has(DEBIT)) {
this.debit = BigDecimal.valueOf(json.getDouble(DEBIT));
}
if (json.has(STATUS)) {
this.status = AccountStatus.parse(json.getInt(STATUS));
}
if (json.has(CREATED_ON)) {
try {
this.createdOn = DateUtils.parseSqlDateTime(json.getString(CREATED_ON));
} catch (ParseException ex) {
throw new JSONException(ex);
}
}
if (json.has(UPDATED_GROUP_ON)) {
try {
this.updatedGroupOn = DateUtils.parseSqlDateTime(json.getString(UPDATED_GROUP_ON));
} catch (ParseException ex) {
throw new JSONException(ex);
}
}
if (json.has(UPDATED_STATUS_ON)) {
try {
this.updatedStatusOn = DateUtils.parseSqlDateTime(json.getString(UPDATED_STATUS_ON));
} catch (ParseException ex) {
throw new JSONException(ex);
}
}
if (json.has(GROUP)) {
this.group = json.getString(GROUP);
}
if (json.has(GROUP_WIN_DOMAIN)) {
this.groupWinDomain = json.getInt(GROUP_WIN_DOMAIN);
}
if (json.has(BONUS)) {
this.bonus = BigDecimal.valueOf(json.getDouble(BONUS));
}
if (json.has(EMAIL)) {
this.email = json.getString(EMAIL);
}
if (json.has(IS_ENABLED)) {
this.isEnabled = json.getInt(IS_ENABLED) == 1;
}
if (json.has(GROUP_TYPE)) {
this.groupType = json.getString(GROUP_TYPE);
}
if (json.has(HARD_NODE_GROUPS)) {
this.hardNodeGroups = new HashSet();
JSONArray jsonArray = json.getJSONArray(HARD_NODE_GROUPS);
for (int i = 0; i < jsonArray.length(); i++) {
this.hardNodeGroups.add(jsonArray.getString(i));
}
}
if (json.has(DEFAULT_HN_GROUP)) {
this.defaultHardNodeGroup = json.getString(DEFAULT_HN_GROUP);
}
if (json.has(PREVIOUS_UPDATE_GROUP_ON)) {
try {
this.previousUpdatedGroupOn = DateUtils.parseSqlDateTime(json.getString(PREVIOUS_UPDATE_GROUP_ON));
} catch (ParseException ex) {
throw new JSONException(ex);
}
}
if (json.has(LANGUAGE)) {
this.language = json.getString(LANGUAGE);
}
if (json.has(EBS_USER_ID)) {
this.ebsUserId = json.getString(EBS_USER_ID);
}
if (json.has(DEFAULT_PAYMENT_METHOD_ID)) {
this.defaultPayMethodId = json.getString(DEFAULT_PAYMENT_METHOD_ID);
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy