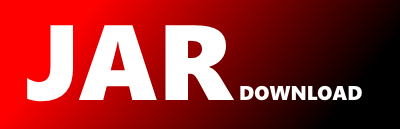
com.jelastic.api.billing.response.ArrayItemResponse Maven / Gradle / Ivy
The newest version!
/*Server class MD5: d7d1c0e4a66b16952927cb3e0d25a2b8*/
package com.jelastic.api.billing.response;
import com.jelastic.api.Response;
import com.jelastic.api.billing.response.interfaces.ArrayItem;
import com.jelastic.api.common.Constants;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.Arrays;
import java.util.Collections;
import java.util.HashSet;
import java.util.Set;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class ArrayItemResponse extends Response {
public static final String PRIVATE_LIB_PACKAGE_PREFIX = "com." + "hivext";
public static final String PUBLIC_LIB_PACKAGE_PREFIX = "com.jelastic";
public static final String SERVER_PACKAGE = ".server";
public static final String CLASS_NAME = "className";
private ArrayItem arrayItem;
private Class extends ArrayItem> className;
public static final String ARRAY_ITEM = "item";
private Set expandablePath;
public ArrayItemResponse() {
super(OK);
}
public ArrayItemResponse(int result, String error) {
super(result, error);
}
public ArrayItemResponse(Response response) {
super(response);
}
public ArrayItemResponse(Class extends ArrayItem> generic, ArrayItem arrayItem, Set expandablePath) {
super(OK);
this.className = generic;
this.arrayItem = arrayItem;
this.expandablePath = expandablePath;
}
public ArrayItemResponse(Class extends ArrayItem> generic, ArrayItem arrayItem, String expandablePath) {
super(OK);
this.className = generic;
this.arrayItem = arrayItem;
if (expandablePath == null || expandablePath.isEmpty()) {
this.expandablePath = Collections.emptySet();
} else if (expandablePath.contains("*")) {
this.expandablePath = null;
} else {
String[] stringArray = expandablePath.split(Constants.SEMICOLON_SEPARATOR);
this.expandablePath = new HashSet<>(Arrays.asList(stringArray));
}
}
public ArrayItemResponse(Class generic, ArrayItem arrayItem) {
this(generic, arrayItem, Collections.emptySet());
}
public ArrayItem getArrayItem() {
return arrayItem;
}
public void setArrayItem(ArrayItem arrayItem) {
this.arrayItem = arrayItem;
}
@Override
protected ArrayItemResponse _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
if (json.has(CLASS_NAME)) {
String clazzName = getClazzName(json);
try {
className = (Class extends ArrayItem>) Class.forName(clazzName);
} catch (ClassNotFoundException ex) {
ex.printStackTrace();
}
}
if (json.has(ARRAY_ITEM)) {
JSONObject arrayJson = json.getJSONObject(ARRAY_ITEM);
try {
Object item = className.newInstance();
arrayItem = ((ArrayItem) item)._fromJSON(arrayJson);
} catch (Exception ex) {
ex.printStackTrace();
}
}
return this;
}
protected JSONObject _toJSON() throws JSONException {
JSONObject json = super._toJSON();
if (arrayItem != null) {
if (expandablePath != null) {
json.put(ARRAY_ITEM, arrayItem._toJsonExpandable(expandablePath));
} else {
json.put(ARRAY_ITEM, arrayItem._toJSON());
}
if (className != null) {
json.put(CLASS_NAME, className.getCanonicalName());
}
}
return json;
}
private String getClazzName(JSONObject json) throws JSONException {
boolean isServerClass = this.getClass().getPackage().toString().contains(SERVER_PACKAGE);
String classNameFromJSON = json.getString(CLASS_NAME);
if (this.getClass().getPackage().getName().startsWith(PUBLIC_LIB_PACKAGE_PREFIX)) {
return classNameFromJSON.replace(PRIVATE_LIB_PACKAGE_PREFIX, PUBLIC_LIB_PACKAGE_PREFIX).replace(SERVER_PACKAGE, "");
}
return isServerClass ? classNameFromJSON : classNameFromJSON.replace(SERVER_PACKAGE, "");
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy