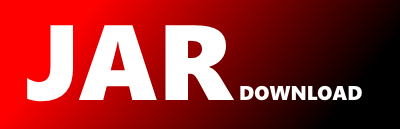
com.jelastic.api.billing.utils.TariffUtils Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 081b2fb7bac8d2dbc71394d73cd91e8b*/
package com.jelastic.api.billing.utils;
import com.jelastic.api.Response;
import com.jelastic.api.common.Constants;
import com.jelastic.api.system.persistence.CalculatingStrategyData;
import com.jelastic.api.system.persistence.TariffPlan;
import com.jelastic.api.system.persistence.TariffPlanType;
import com.jelastic.api.system.persistence.Tier;
import java.util.stream.Collectors;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class TariffUtils {
public final static List MULTI_ASSIGNED_TYPES = Arrays.asList(TariffPlanType.LICENCE, TariffPlanType.EXTERNAL);
public static ArrayList sortTiers(Set tiers) {
ArrayList sortedTiers = new ArrayList(tiers);
sortedTiers.sort(Comparator.comparingDouble(Tier::getValue));
return sortedTiers;
}
public static Response validateTiers(Set tiers) {
if (tiers.isEmpty()) {
return new Response(Response.INVALID_PARAM, "No tiers found.");
}
Tier previousTier = null;
ArrayList sortedTiers = sortTiers(tiers);
for (Tier sortedTier : sortedTiers) {
if (sortedTier.getPrice() >= 100000000000L) {
return new Response(Response.INVALID_PARAM, "'price' must be < 100000000000");
}
if (previousTier == null && sortedTier.getValue() < 1) {
return new Response(Response.INVALID_PARAM, "'value' of the first tier must be >= 1");
}
if (sortedTier.getPrice() < 0 || sortedTier.getFree() < 0) {
return new Response(Response.INVALID_PARAM, "'price' and 'free' of the tier must be >= 0");
}
if (previousTier != null && previousTier.getValue() == sortedTier.getValue()) {
return new Response(Response.INVALID_PARAM, "next tier 'value' must be greater than previous");
}
previousTier = sortedTier;
}
return Response.OK();
}
public static Set getTariffsPlansDifference(Set first, Set second) {
Set difference = new HashSet<>();
difference.addAll(subtractTariffPlans(first, second));
difference.addAll(subtractTariffPlans(second, first));
return difference;
}
private static Set subtractTariffPlans(Set first, Set second) {
Set difference = new HashSet<>();
for (TariffPlan tariffPlan : first) {
if (!tariffPlan.isInArray(second)) {
difference.add(tariffPlan);
}
}
return difference;
}
public static Response validateTariffPlans(Set tariffPlans) {
Map> validator = new HashMap<>();
for (TariffPlan tariffPlan : tariffPlans) {
Set modelTypeKeywords = validator.get(tariffPlan.getType());
if (modelTypeKeywords == null) {
Set keywords = new HashSet<>();
keywords.add(tariffPlan.getKeyword());
validator.put(tariffPlan.getType(), keywords);
continue;
}
if (modelTypeKeywords.contains(tariffPlan.getKeyword()) && !MULTI_ASSIGNED_TYPES.contains(tariffPlan.getType())) {
return new Response(Response.INVALID_PARAM, "Only one tariff plan with type [" + tariffPlan.getType() + "] and keyword [" + tariffPlan.getKeyword() + "] must be assigned.");
}
modelTypeKeywords.add(tariffPlan.getKeyword());
}
return Response.OK();
}
public static TariffPlan findTariffPlan(Set tariffPlans, TariffPlanType type, String keyword) {
List suitableTariffPlans = tariffPlans.stream().filter(plan -> plan.getType().equals(type)).collect(Collectors.toList());
TariffPlan tariffPlan = suitableTariffPlans.stream().filter(plan -> plan.getKeyword().equalsIgnoreCase(keyword)).findFirst().orElse(null);
if (tariffPlan == null) {
tariffPlan = suitableTariffPlans.stream().filter(plan -> plan.getKeyword().equalsIgnoreCase(Constants.TARIFF_PLAN_TYPE_DEFAULT)).findFirst().orElse(null);
}
return tariffPlan;
}
public static void overrideStrategyData(TariffPlan newTariffPlan, TariffPlan clonedTariffPlan) {
if (newTariffPlan.getStrategyData() != null) {
CalculatingStrategyData data = newTariffPlan.getStrategyData();
if (clonedTariffPlan.getStrategyData() != null) {
if (data.getRoundingMode() == null && !data.isRoundingModeReceived()) {
data.setRoundingMode(clonedTariffPlan.getStrategyData().getRoundingMode());
}
if (data.getScale() == -1) {
data.setScale(clonedTariffPlan.getStrategyData().getScale());
}
if (data.getStrategyMode() == null) {
data.setStrategyMode(clonedTariffPlan.getStrategyData().getStrategyMode());
}
}
clonedTariffPlan.setStrategyData(data);
}
}
}