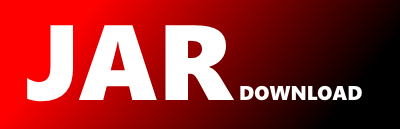
com.jelastic.api.core.utils.CollectionUtils Maven / Gradle / Ivy
The newest version!
/*Server class MD5: b012e11a957f142bf2aa261cd1571a1c*/
package com.jelastic.api.core.utils;
import java.util.Collection;
import java.util.HashMap;
import java.util.Map;
import java.util.Set;
import java.util.concurrent.ConcurrentHashMap;
import java.util.function.Function;
import java.util.function.Predicate;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class CollectionUtils {
public static boolean isEmpty(Collection coll) {
return (coll == null || coll.isEmpty());
}
public static Predicate distinctByKey(Function super T, ?> keyExtractor) {
Set
© 2015 - 2025 Weber Informatics LLC | Privacy Policy