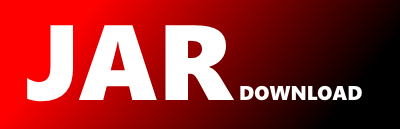
com.jelastic.api.core.utils.JSONUtils Maven / Gradle / Ivy
/*Server class MD5: 14490e8c044c7651523101eba1b0e3d2*/
package com.jelastic.api.core.utils;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class JSONUtils {
public static final Object toJSON(Object obj) {
if (obj == null) {
return null;
}
Object respone = null;
try {
if (isSimpleType(obj) || obj instanceof JSONObject || obj instanceof JSONArray) {
respone = obj;
} else if (obj instanceof Map) {
respone = new JSONObject((Map) obj);
} else if (obj instanceof String) {
String str = ((String) obj).trim();
if (str.startsWith("[") && str.endsWith("]")) {
respone = new JSONArray(str);
} else if (str.startsWith("{") && str.endsWith("}")) {
respone = new JSONObject(str);
} else {
respone = obj;
}
} else if (obj instanceof Collection) {
respone = new JSONArray((Collection) obj);
} else if (obj.getClass().isArray()) {
Object[] arr = (Object[]) obj;
JSONArray jsonArray = new JSONArray();
for (int i = 0; i < arr.length; i++) {
jsonArray.put(toJSON(arr[i]));
}
respone = jsonArray;
} else {
respone = obj;
}
} catch (Exception ex) {
ex.printStackTrace();
respone = ex.toString();
}
return respone;
}
public static final String jsonStringify(Object obj) {
if (obj == null) {
return "";
}
if (obj instanceof String) {
return (String) obj;
} else {
return toJSON(obj).toString();
}
}
public static final Map mapify(Object obj) {
return mapify(obj, false);
}
public static final Map mapify(Object obj, boolean stringify) {
if (obj != null && obj instanceof Map) {
return (Map) obj;
}
Map map = new HashMap();
if (obj != null) {
Object toJson = toJSON(obj);
if (toJson instanceof String && !(obj instanceof String)) {
toJson = toJSON(toJson);
}
if (toJson instanceof JSONObject) {
JSONObject json = (JSONObject) toJson;
Iterator keys = json.keys();
try {
while (keys.hasNext()) {
String key = (String) keys.next();
if (stringify) {
map.put(key, String.valueOf(json.get(key)));
} else {
map.put(key, json.get(key));
}
}
} catch (JSONException ex) {
throw new RuntimeException(ex.toString());
}
} else {
throw new RuntimeException("error mapify object [" + obj.getClass() + " -> " + obj + "]");
}
}
return map;
}
public final static boolean isSimpleType(Object obj) {
if (obj == null) {
return false;
}
Class clazz = obj.getClass();
return clazz == boolean.class || clazz == byte.class || clazz == char.class || clazz == short.class || clazz == int.class || clazz == long.class || clazz == float.class || clazz == double.class || clazz == Boolean.class || clazz == Byte.class || clazz == Character.class || clazz == Short.class || clazz == Integer.class || clazz == Long.class || clazz == Float.class || clazz == Double.class;
}
public static Object getFieldValueFromJson(JSONObject jsonObject, String fieldName) {
if (jsonObject == null) {
return null;
}
if (jsonObject.has(fieldName) && !jsonObject.isNull(fieldName)) {
try {
return jsonObject.get(fieldName);
} catch (JSONException e) {
return null;
}
}
return null;
}
public static JSONObject mergeJsonObjects(JSONObject... jsonObject) {
JSONObject mergedJsonObject = new JSONObject();
return mergeIntoJsonObject(mergedJsonObject, jsonObject);
}
public static JSONObject mergeIntoJsonObject(JSONObject baseObject, JSONObject... jsonObject) {
for (JSONObject item : jsonObject) {
Iterator iterator = item.keys();
while (iterator.hasNext()) {
String fieldName = iterator.next().toString();
try {
baseObject.put(fieldName, item.get(fieldName));
} catch (JSONException e) {
e.printStackTrace();
}
}
}
return baseObject;
}
public static Map toMap(String jsonString) {
Map result = new HashMap<>();
if (jsonString == null || jsonString.isEmpty()) {
return result;
}
JSONObject jsonObject;
try {
jsonObject = new JSONObject(jsonString);
} catch (JSONException e) {
throw new RuntimeException(String.format("Can't parse string '%s' to JSON", jsonString), e);
}
if (jsonObject != JSONObject.NULL) {
Iterator keysIterator = jsonObject.keys();
while (keysIterator.hasNext()) {
String key = keysIterator.next();
Object value = jsonObject.opt(key);
result.put(key, value == null ? null : String.valueOf(value));
}
}
return result;
}
public static boolean isJSONArray(Object object) {
if (object == null) {
return false;
}
if (object instanceof JSONArray) {
return true;
} else if (object instanceof JSONObject) {
return false;
}
try {
new JSONArray((String) object);
return true;
} catch (JSONException ignored) {
return false;
}
}
public static boolean isJSONObject(Object object) {
if (object == null) {
return false;
}
if (object instanceof JSONObject) {
return true;
}
try {
new JSONObject((String) object);
return true;
} catch (JSONException ignored) {
return false;
}
}
public static Set toIntegerSet(String jsonArray) throws JSONException {
JSONArray array = new JSONArray(jsonArray);
HashSet result = new HashSet<>();
for (int i = 0; i < array.length(); i++) {
result.add(array.getInt(i));
}
return result;
}
public static JSONArray toJSONArray(Collection collection) {
if (CollectionUtils.isEmpty(collection)) {
return null;
}
JSONArray jsonArray = new JSONArray();
for (T item : collection) {
jsonArray.put(item);
}
return jsonArray;
}
public static HashSet fromJSONArray(String jsonArrayString) throws JSONException {
if (StringUtils.isBlank(jsonArrayString)) {
return null;
}
return fromJSONArray(new JSONArray(jsonArrayString));
}
public static HashSet fromJSONArray(JSONArray jsonArray) throws JSONException {
if (jsonArray == null) {
return null;
}
HashSet result = new HashSet<>();
for (int i = 0; i < jsonArray.length(); i++) {
result.add((T) jsonArray.get(i));
}
return result;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy