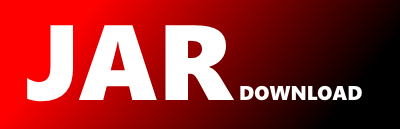
com.jelastic.api.data.po.DockerLink Maven / Gradle / Ivy
/*Server class MD5: f0c2a8fce96520e460a1573ec709a09a*/
package com.jelastic.api.data.po;
import com.jelastic.api.core.utils.CommonJSONUtils;
import org.json.JSONException;
import org.json.JSONObject;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class DockerLink {
public static final String SOURCE_NODE_ID = "sourceNodeId";
public static final String TARGET_NODE_ID = "targetNodeId";
public static final String ALIAS = "alias";
public static final String TYPE = "type";
public static final String GROUP_ALIAS = "groupAlias";
private JSONObject jsonObject = new JSONObject();
public DockerLink() {
}
public DockerLink(int sourceNodeId, int targetNodeId, String alias, DockerLinkType linkType, String groupAlias) {
try {
jsonObject.put(SOURCE_NODE_ID, sourceNodeId);
jsonObject.put(TARGET_NODE_ID, targetNodeId);
jsonObject.put(ALIAS, alias);
jsonObject.put(TYPE, linkType.toString());
jsonObject.put(GROUP_ALIAS, groupAlias);
} catch (JSONException e) {
e.printStackTrace();
}
}
public DockerLink(DockerLink link) {
try {
jsonObject.put(SOURCE_NODE_ID, link.getSourceNodeId());
jsonObject.put(TARGET_NODE_ID, link.getTargetNodeId());
jsonObject.put(ALIAS, link.getAlias());
jsonObject.put(TYPE, link.getType().toString());
jsonObject.put(ALIAS, link.getAlias());
jsonObject.put(GROUP_ALIAS, link.getGroupAlias());
} catch (JSONException e) {
e.printStackTrace();
}
}
public Integer getSourceNodeId() {
return (Integer) CommonJSONUtils.getFieldValueFromJson(this.jsonObject, SOURCE_NODE_ID);
}
public void setSourceNodeId(Integer sourceNodeId) {
try {
this.jsonObject.put(SOURCE_NODE_ID, sourceNodeId);
} catch (JSONException e) {
e.printStackTrace();
}
}
public Integer getTargetNodeId() {
return (Integer) CommonJSONUtils.getFieldValueFromJson(this.jsonObject, TARGET_NODE_ID);
}
public void setTargetNodeId(Integer targetNodeId) {
try {
this.jsonObject.put(TARGET_NODE_ID, targetNodeId);
} catch (JSONException e) {
e.printStackTrace();
}
}
public String getAlias() {
return (String) CommonJSONUtils.getFieldValueFromJson(this.jsonObject, ALIAS);
}
public void setAlias(String alias) {
try {
this.jsonObject.put(ALIAS, alias);
} catch (JSONException e) {
e.printStackTrace();
}
}
public String getGroupAlias() {
return (String) CommonJSONUtils.getFieldValueFromJson(this.jsonObject, GROUP_ALIAS);
}
public void setGroupAlias(String groupAlias) {
try {
this.jsonObject.put(GROUP_ALIAS, groupAlias);
} catch (JSONException e) {
e.printStackTrace();
}
}
public DockerLinkType getType() {
return DockerLinkType.valueOf(CommonJSONUtils.getFieldValueFromJson(this.jsonObject, TYPE).toString());
}
public void setType(DockerLinkType type) {
try {
this.jsonObject.put(TYPE, type.toString());
} catch (JSONException e) {
e.printStackTrace();
}
}
@Override
public String toString() {
return this.jsonObject.toString();
}
public JSONObject _toJSON() {
return jsonObject;
}
public DockerLink _fromJSON(JSONObject json) {
try {
if (json.has(SOURCE_NODE_ID)) {
setSourceNodeId(json.getInt(SOURCE_NODE_ID));
}
if (json.has(TARGET_NODE_ID)) {
setTargetNodeId(json.getInt(TARGET_NODE_ID));
}
if (json.has(ALIAS)) {
setAlias(json.getString(ALIAS));
}
if (json.has(TYPE)) {
setType(DockerLinkType.valueOf(json.getString(TYPE)));
}
if (json.has(GROUP_ALIAS)) {
setGroupAlias(json.getString(GROUP_ALIAS));
}
} catch (JSONException e) {
e.printStackTrace();
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy