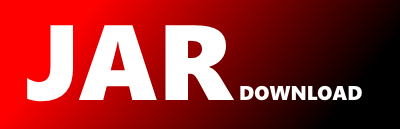
com.jelastic.api.data.po.DockerMetadata Maven / Gradle / Ivy
package com.jelastic.api.data.po;
import com.jelastic.api.core.utils.JSONUtils;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.ArrayList;
import java.util.HashSet;
import java.util.List;
import java.util.Set;
/**
* @author Maksym Shevchuk
*/
public class DockerMetadata implements DBItem {
public static final String DOCKER_VERSION_SEPARATOR = ":";
public static final String DOCKER_LINKS = "dockerLinks";
public static final String DOCKER_NAME = "dockerName";
public static final String DOCKER_HUB_URL = "registryUrl";
public static final String DOCKER_HUB_USER = "registryUser";
public static final String DOCKER_HUB_PASSWORD = "registryPassword";
public static final String DOCKER_TAG = "dockerTag";
public static final String DOCKER_OS = "dockerOs";
public static final String DOCKER_MANIFEST = "dockerManifest";
public static final String DOCKER_VOLUMES = "dockerVolumes";
public static final String DOCKER_VOLUMES_FROM = "dockerVolumesFrom";
public static final String DOCKER_RUN_ARGS = "dockerRunCmd";
public static final String DOCKER_ENTRY_POINT = "dockerEntryPoint";
public static final String DOCKER_ENV_VARS = "dockerEnvVars";
public static final String PROJECTS = "projects";
private JSONObject jsonObject = new JSONObject();
public DockerMetadata(String data) throws JSONException {
_fromString(data);
}
public DockerMetadata(JSONObject data) throws JSONException {
_fromJSON(data);
}
public JSONObject getManifest() {
return (JSONObject) JSONUtils.getFieldValueFromJson(this.jsonObject, DOCKER_MANIFEST);
}
public void setManifest(JSONObject manifest) {
this.jsonObject.put(DOCKER_MANIFEST, manifest);
}
public String getFullName() {
return getName() + DOCKER_VERSION_SEPARATOR + getTag();
}
public String getName() {
return (String) JSONUtils.getFieldValueFromJson(this.jsonObject, DOCKER_NAME);
}
public void setName(String name) {
this.jsonObject.put(DOCKER_NAME, name);
}
public String getHubUrl() {
return (String) JSONUtils.getFieldValueFromJson(this.jsonObject, DOCKER_HUB_URL);
}
public void setHubUrl(String hubUrl) {
this.jsonObject.put(DOCKER_HUB_URL, hubUrl);
}
public String getTag() {
return (String) JSONUtils.getFieldValueFromJson(this.jsonObject, DOCKER_TAG);
}
public void setTag(String tag) {
this.jsonObject.put(DOCKER_TAG, tag);
}
public String getOs() {
return (String) JSONUtils.getFieldValueFromJson(this.jsonObject, DOCKER_OS);
}
public void setOs(String os) {
this.jsonObject.put(DOCKER_OS, os);
}
public String getHubUser() {
return (String) JSONUtils.getFieldValueFromJson(this.jsonObject, DOCKER_HUB_USER);
}
public void setHubUser(String hubUser) {
this.jsonObject.put(DOCKER_HUB_USER, hubUser);
}
public String getHubPassword() {
return (String) JSONUtils.getFieldValueFromJson(this.jsonObject, DOCKER_HUB_PASSWORD);
}
public void setHubPassword(String hubPassword) {
this.jsonObject.put(DOCKER_HUB_PASSWORD, hubPassword);
}
public Set getLinks() {
Set dockerLinkSet = new HashSet();
JSONArray dockerLinkJsonArray = (JSONArray) JSONUtils.getFieldValueFromJson(this.jsonObject, DOCKER_LINKS);
if (dockerLinkJsonArray == null) {
return dockerLinkSet;
}
for (int i = 0; i < dockerLinkJsonArray.length(); i++) {
dockerLinkSet.add(new DockerLink()._fromJSON(dockerLinkJsonArray.getJSONObject(i)));
}
return dockerLinkSet;
}
public void setLinks(Set dockerLinks) {
JSONArray dockerLinkJsonArray = new JSONArray();
for (DockerLink dockerLink : dockerLinks) {
dockerLinkJsonArray.put(dockerLink._toJSON());
}
jsonObject.put(DOCKER_LINKS, dockerLinkJsonArray);
}
public Set getVolumes() {
Set dockerVolumeSet = new HashSet();
JSONArray dockerVolumeJsonArray = (JSONArray) JSONUtils.getFieldValueFromJson(this.jsonObject, DOCKER_VOLUMES);
if (dockerVolumeJsonArray == null) {
return dockerVolumeSet;
}
for (int i = 0; i < dockerVolumeJsonArray.length(); i++) {
dockerVolumeSet.add(dockerVolumeJsonArray.getString(i));
}
return dockerVolumeSet;
}
public void setVolumes(Set dockerVolumes) {
JSONArray dockerVolumeJsonArray = new JSONArray();
for (String dockerVolume : dockerVolumes) {
dockerVolumeJsonArray.put(dockerVolume);
}
jsonObject.put(DOCKER_VOLUMES, dockerVolumeJsonArray);
}
public List getVolumesFrom() throws JSONException {
List dockerVolumeSet = new ArrayList();
JSONArray dockerVolumeJsonArray = (JSONArray) JSONUtils.getFieldValueFromJson(this.jsonObject, DOCKER_VOLUMES_FROM);
if (dockerVolumeJsonArray == null) {
return dockerVolumeSet;
}
for (int i = 0; i < dockerVolumeJsonArray.length(); i++) {
dockerVolumeSet.add(dockerVolumeJsonArray.getString(i));
}
return dockerVolumeSet;
}
public void setVolumesFrom(List dockerVolumes) throws JSONException {
JSONArray dockerVolumeJsonArray = new JSONArray();
for (String dockerVolume : dockerVolumes) {
dockerVolumeJsonArray.put(dockerVolume);
}
jsonObject.put(DOCKER_VOLUMES_FROM, dockerVolumeJsonArray);
}
public String getRaw() {
return jsonObject.toString();
}
private void _fromString(String stringJson) throws JSONException {
JSONObject json = new JSONObject(stringJson);
this._fromJSON(json);
}
@Override
public String toString() {
return this.jsonObject.toString();
}
@Override
public JSONObject _toJSON() {
JSONObject jsonObject = new JSONObject(this.jsonObject.toString());
jsonObject.remove(DOCKER_HUB_USER);
jsonObject.remove(DOCKER_HUB_PASSWORD);
return jsonObject;
}
@Override
public DockerMetadata _fromJSON(JSONObject json) throws JSONException {
this.jsonObject = new JSONObject(json.toString());
return this;
}
public JSONObject toJSON(boolean isExport) {
JSONObject jsonObject;
try {
jsonObject = new JSONObject(this.jsonObject.toString());
} catch (JSONException e) {
e.printStackTrace();
return new JSONObject();
}
if (!isExport) {
jsonObject.remove(DOCKER_HUB_USER);
jsonObject.remove(DOCKER_HUB_PASSWORD);
}
jsonObject.remove(DOCKER_RUN_ARGS);
jsonObject.remove(DOCKER_ENTRY_POINT);
jsonObject.remove(DOCKER_ENV_VARS);
jsonObject.remove(PROJECTS);
return jsonObject;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy