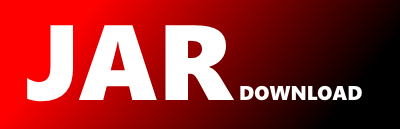
com.jelastic.api.data.po.MountPoint Maven / Gradle / Ivy
The newest version!
/*Server class MD5: b6252ef0d075578f4d58c9b65a4e491f*/
package com.jelastic.api.data.po;
import com.jelastic.api.common.Constants;
import com.jelastic.api.data.SourceAddressType;
import com.jelastic.api.development.response.interfaces.ArrayItem;
import com.jelastic.api.system.persistence.FilePermissionCreator;
import com.jelastic.api.system.persistence.MountPointType;
import org.json.JSONException;
import org.json.JSONObject;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class MountPoint extends ArrayItem {
public static final String NODE_ID = "nodeId";
public static final String SOURCE_NODE_ID = "sourceNodeId";
public static final String SOURCE_HOST = "sourceHost";
public static final String SOURCE_PATH = "sourcePath";
public static final String SOURCE_ADDRESS_TYPE = "sourceAddressType";
public static final String PATH = "path";
public static final String PROTOCOL = "protocol";
public static final String NAME = "name";
public static final String PERMISSION = "permission";
public static final String CREATOR = "creator";
public static final String TYPE = "type";
public static final String READ_ONLY = "readOnly";
private Integer nodeId;
private Integer sourceNodeId;
private String sourceHost;
private String sourcePath;
private String path;
private String protocol;
private String name;
private Boolean readOnly = false;
private FilePermissionCreator creator;
private MountPointType type;
private SourceAddressType sourceAddressType;
public MountPoint() {
}
public MountPoint(Integer nodeId, Integer sourceNodeId, String sourceHost, String sourcePath, String path, String protocol, String name, Boolean readOnly, FilePermissionCreator creator, MountPointType type, SourceAddressType sourceAddressType) {
this.nodeId = nodeId;
this.sourceNodeId = sourceNodeId;
this.sourceHost = sourceHost;
this.sourcePath = sourcePath;
this.path = path;
this.protocol = protocol;
this.name = name;
this.readOnly = readOnly;
this.creator = creator;
this.type = type;
this.sourceAddressType = sourceAddressType;
}
public Integer getSourceNodeId() {
return sourceNodeId;
}
public void setSourceNodeId(Integer sourceNodeId) {
this.sourceNodeId = sourceNodeId;
}
public String getSourceHost() {
return sourceHost;
}
public void setSourceHost(String sourceHost) {
this.sourceHost = sourceHost;
}
public String getSourcePath() {
return sourcePath;
}
public void setSourcePath(String sourcePath) {
this.sourcePath = sourcePath;
}
public String getPath() {
return path;
}
public void setPath(String path) {
this.path = path;
}
public String getProtocol() {
return protocol;
}
public void setProtocol(String protocol) {
this.protocol = protocol;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public Integer getNodeId() {
return nodeId;
}
public void setNodeId(Integer nodeId) {
this.nodeId = nodeId;
}
public FilePermissionCreator getCreator() {
return creator;
}
public void setCreator(FilePermissionCreator creator) {
this.creator = creator;
}
public MountPointType getType() {
return type;
}
public void setType(MountPointType type) {
this.type = type;
}
public Boolean getReadOnly() {
return readOnly;
}
public void setReadOnly(Boolean readOnly) {
this.readOnly = readOnly;
}
public String getPermission() {
return readOnly ? Constants.FILE_READ_ONLY_PERMISSIONS : Constants.FILE_RW_PERMISSIONS;
}
public SourceAddressType getSourceAddressType() {
return sourceAddressType;
}
public void setSourceAddressType(SourceAddressType sourceAddressType) {
this.sourceAddressType = sourceAddressType;
}
public JSONObject _toJSON() throws JSONException {
JSONObject jsonObject = new JSONObject();
jsonObject.put(NODE_ID, nodeId);
jsonObject.put(SOURCE_NODE_ID, sourceNodeId);
jsonObject.put(SOURCE_HOST, sourceHost);
jsonObject.put(SOURCE_PATH, sourcePath);
jsonObject.put(PATH, path);
jsonObject.put(PROTOCOL, protocol);
jsonObject.put(NAME, name);
jsonObject.put(READ_ONLY, readOnly);
jsonObject.put(CREATOR, creator);
jsonObject.put(TYPE, type);
jsonObject.put(PERMISSION, getPermission());
jsonObject.put(SOURCE_ADDRESS_TYPE, sourceAddressType);
return jsonObject;
}
public MountPoint _fromJSON(JSONObject jsonObject) throws JSONException {
if (jsonObject.has(NODE_ID)) {
nodeId = jsonObject.getInt(NODE_ID);
}
if (jsonObject.has(SOURCE_NODE_ID)) {
sourceNodeId = jsonObject.getInt(SOURCE_NODE_ID);
}
if (jsonObject.has(SOURCE_HOST)) {
sourceHost = jsonObject.getString(SOURCE_HOST);
}
if (jsonObject.has(SOURCE_PATH)) {
sourcePath = jsonObject.getString(SOURCE_PATH);
}
if (jsonObject.has(PATH)) {
path = jsonObject.getString(PATH);
}
if (jsonObject.has(PROTOCOL)) {
protocol = jsonObject.getString(PROTOCOL);
}
if (jsonObject.has(NAME)) {
name = jsonObject.getString(NAME);
}
if (jsonObject.has(READ_ONLY)) {
readOnly = jsonObject.getBoolean(READ_ONLY);
}
if (jsonObject.has(CREATOR) && !jsonObject.isNull(CREATOR)) {
creator = FilePermissionCreator.valueOf(jsonObject.getString(CREATOR).toUpperCase());
}
if (jsonObject.has(TYPE) && !jsonObject.isNull(TYPE)) {
type = MountPointType.valueOf(jsonObject.getString(TYPE));
}
if (jsonObject.has(SOURCE_ADDRESS_TYPE) && !jsonObject.isNull(SOURCE_ADDRESS_TYPE)) {
sourceAddressType = SourceAddressType.valueOf(jsonObject.getString(SOURCE_ADDRESS_TYPE).toUpperCase());
}
return this;
}
@Override
public int hashCode() {
return (nodeId + ":" + path).hashCode();
}
@Override
public boolean equals(Object object) {
if (!(object instanceof MountPoint)) {
return false;
}
MountPoint input = (MountPoint) object;
return this.getNodeId().equals(input.getNodeId()) && this.getPath().equals(input.getPath());
}
@Override
public String toString() {
return this.getNodeId() + ":" + this.getPath() + " -> " + this.getSourceNodeId() + ":" + this.getSourcePath();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy