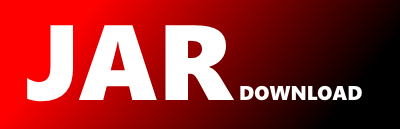
com.jelastic.api.data.response.ObjectResponse Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 33cc6a6ae07e506296d7c14fc5375fc4*/
package com.jelastic.api.data.response;
import com.jelastic.api.Response;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class ObjectResponse extends Response {
protected int id = -1;
protected Map fields = null;
private JSONObject object = null;
public ObjectResponse(int id) {
super(Response.OK);
this.id = id;
}
public ObjectResponse(Map fields) {
super(Response.OK);
setFields(fields);
}
public ObjectResponse() {
super(Response.OK);
}
public ObjectResponse(int result, String error) {
super(result, error);
}
public Map getFields() {
return fields;
}
public void setFields(Map fields) {
this.fields = fields;
toJSON();
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public JSONObject getObject() {
return object;
}
@Override
public JSONObject toJSON() {
JSONObject json = super.toJSON();
try {
if (id > -1) {
json.put("id", id);
}
if (fields != null) {
JSONObject fieldJson = new JSONObject();
for (String field : fields.keySet()) {
Object value = fields.get(field);
fieldJson.put(field, value == null ? JSONObject.NULL : value);
}
object = fieldJson;
json.put("object", fieldJson);
}
} catch (Exception ex) {
ex.printStackTrace();
try {
json.put("result", Response.ERROR_UNKNOWN).put("error", ex.toString());
} catch (JSONException exc) {
}
}
return json;
}
@Override
public ObjectResponse _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
if (json.has("id")) {
this.id = json.getInt("id");
}
if (json.has("object")) {
this.object = json.getJSONObject("object");
Iterator keys = object.keys();
this.fields = new HashMap();
while (keys.hasNext()) {
String key = (String) keys.next();
fields.put(key, object.get(key));
}
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy