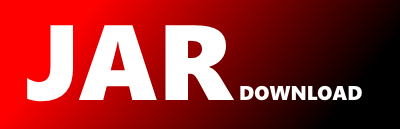
com.jelastic.api.data.response.ObjectsResponse Maven / Gradle / Ivy
The newest version!
/*Server class MD5: df8b273a2e5e17b9826f69be58676f25*/
package com.jelastic.api.data.response;
import com.jelastic.api.Response;
import com.jelastic.api.json.JSONSerializable;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.*;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class ObjectsResponse extends Response implements JSONSerializable {
protected Collection
© 2015 - 2025 Weber Informatics LLC | Privacy Policy