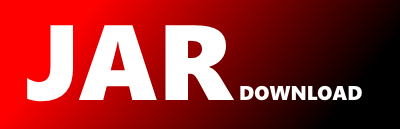
com.jelastic.api.development.persistence.Application Maven / Gradle / Ivy
The newest version!
package com.jelastic.api.development.persistence;
import java.io.Serializable;
import java.util.*;
/**
*
* @author Ruslan Sinitskiy
*/
public class Application implements Serializable {
private int id;
private String appid;
private String name;
private String domain = "";
private String description = "";
private String keywords = "";
private Date date = new Date();
private ApplicationStatus status = ApplicationStatus.ENABLED;
private Map users = new HashMap();
private Set allowSessionFromApp = new TreeSet();
private ApplicationsPool appsPool = null;
private ApplicationConfig appConfig = null;
private Application creatorApp = null;
private User creatorUser = null;
private Application sourceApp = null;
private boolean clonable = false;
public Map getUsers() {
return users;
}
public void setUsers(Map users) {
this.users = users;
}
public Set getAllowSessionFromApp() {
return allowSessionFromApp;
}
public void setAllowSessionFromApp(Set allowSessionFromApp) {
this.allowSessionFromApp = allowSessionFromApp;
}
public Date getDate() {
return date;
}
public void setDate(Date date) {
this.date = date;
}
public String getDescription() {
return description;
}
public void setDescription(String description) {
this.description = description;
}
public String getKeywords() {
return keywords;
}
public void setKeywords(String keywords) {
this.keywords = keywords;
}
public String getDomain() {
return domain;
}
public void setDomain(String domain) {
this.domain = domain;
}
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public String getName() {
return name;
}
public void setName(String name) {
this.name = name;
}
public ApplicationStatus getStatus() {
return status;
}
public void setStatus(ApplicationStatus status) {
this.status = status;
}
public String getAppid() {
return appid;
}
public void setAppid(String appid) {
this.appid = appid;
}
public Application getCreatorApp() {
return creatorApp;
}
public void setCreatorApp(Application creatorApp) {
this.creatorApp = creatorApp;
}
public User getCreatorUser() {
return creatorUser;
}
public void setCreatorUser(User creatorUser) {
this.creatorUser = creatorUser;
}
public ApplicationsPool getAppsPool() {
return appsPool;
}
public void setAppsPool(ApplicationsPool appsPool) {
this.appsPool = appsPool;
}
public ApplicationConfig getAppConfig() {
if (appConfig == null) {
//first version of Structure
ApplicationConfig config = new ApplicationConfig();
config.setAdd_app_id(true);
config.setDiff_db_tables_service(false);
config.setStructure_version(1.0f);
config.setField_prefix("f_");
config.setType_prefix("type_");
return config;
}
return appConfig;
}
public void setAppConfig(ApplicationConfig appConfig) {
this.appConfig = appConfig;
}
public Application getSourceApp() {
return sourceApp;
}
public void setSourceApp(Application sourceApp) {
this.sourceApp = sourceApp;
}
public boolean isClonable() {
return clonable;
}
public void setClonable(boolean clonable) {
this.clonable = clonable;
}
@Override
public boolean equals(Object obj) {
if (obj == null) {
return false;
}
/*if (getClass() != (obj instanceof HibernateProxy ? HibernateProxyHelper.getClassWithoutInitializingProxy(obj) : obj.getClass())) {
return false;
}*/
final Application other = (Application) obj;
if (getId() != other.getId()) {
return false;
}
return true;
}
/**
* Object вместо ApplicationDefinedTypeInterface потому что JAXB ругается на интерфейс -
* ApplicationDefinedTypeInterface is an interface, and JAXB can't handle interfaces
*/
/*
@Transient
private ApplicationDefinedTypeInterface appDefType = null;
public Object getAppDefType() {
return appDefType;
}
public void setAppDefType(Object appDefType) {
this.appDefType = (ApplicationDefinedTypeInterface) appDefType;
}*/
@Override
public String toString() {
String str = super.toString();
str += ":app_id=" + id;
return str;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy