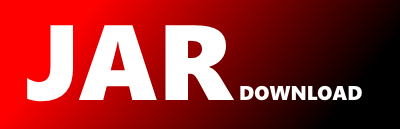
com.jelastic.api.development.persistence.ApplicationConfig Maven / Gradle / Ivy
The newest version!
package com.jelastic.api.development.persistence;
import org.json.JSONException;
import org.json.JSONObject;
import java.io.Serializable;
import java.util.Properties;
/**
*
* @author Ruslan Sinitskiy
*/
public class ApplicationConfig implements Serializable, Cloneable {
private int id;
private float structure_version = 2.7f;
private boolean diff_db_tables_service;
private boolean add_app_id;
private String type_prefix;
private String field_prefix;
private boolean use_access_control;
private boolean log_access_actions;
private String db_engine;
private String db_prefix;
private boolean use_cache_table_meta;
private boolean show_sys_types;
private boolean lazy_load_types;
private int lazy_unload_types_time;
private boolean create_persistence;
private boolean create_resources;
private boolean notify_by_email;
public int getId() {
return id;
}
public void setId(int id) {
this.id = id;
}
public boolean isDiff_db_tables_service() {
return diff_db_tables_service;
}
public void setDiff_db_tables_service(boolean diff_db_tables_service) {
this.diff_db_tables_service = diff_db_tables_service;
}
public boolean isAdd_app_id() {
return add_app_id;
}
public void setAdd_app_id(boolean add_app_id) {
this.add_app_id = add_app_id;
}
public float getStructure_version() {
return structure_version;
}
public void setStructure_version(float structure_version) {
this.structure_version = structure_version;
}
public String getField_prefix() {
return field_prefix;
}
public void setField_prefix(String field_prefix) {
this.field_prefix = field_prefix;
}
public String getType_prefix() {
return type_prefix;
}
public void setType_prefix(String type_prefix) {
this.type_prefix = type_prefix;
}
public boolean isLog_access_actions() {
return use_access_control && log_access_actions;
}
public void setLog_access_actions(boolean log_access_actions) {
this.log_access_actions = log_access_actions;
}
public boolean isUse_access_control() {
return use_access_control;
}
public void setUse_access_control(boolean use_access_control) {
this.use_access_control = use_access_control;
}
public String getDb_engine() {
return db_engine;
}
public void setDb_engine(String db_engine) {
this.db_engine = db_engine;
}
public String getDb_prefix() {
return db_prefix;
}
public void setDb_prefix(String db_prefix) {
this.db_prefix = db_prefix;
}
public boolean isUse_cache_table_meta() {
return use_cache_table_meta;
}
public void setUse_cache_table_meta(boolean use_cache_table_meta) {
this.use_cache_table_meta = use_cache_table_meta;
}
public boolean isShow_sys_types() {
return show_sys_types;
}
public void setShow_sys_types(boolean show_sys_types) {
this.show_sys_types = show_sys_types;
}
public boolean isLazy_load_types() {
return lazy_load_types;
}
public void setLazy_load_types(boolean lazy_load_types) {
this.lazy_load_types = lazy_load_types;
}
public int getLazy_unload_types_time() {
return lazy_unload_types_time;
}
public void setLazy_unload_types_time(int lazy_unload_types_time) {
this.lazy_unload_types_time = lazy_unload_types_time;
}
public boolean isCreate_persistence() {
return create_persistence;
}
public void setCreate_persistence(boolean create_persistence) {
this.create_persistence = create_persistence;
}
public boolean isCreate_resources() {
return create_resources;
}
public void setCreate_resources(boolean create_resources) {
this.create_resources = create_resources;
}
public boolean isNotify_by_email() {
return notify_by_email;
}
public void setNotify_by_email(boolean notify_by_email) {
this.notify_by_email = notify_by_email;
}
@Override
public ApplicationConfig clone() {
ApplicationConfig appConf = new ApplicationConfig();
appConf.setAdd_app_id(add_app_id);
appConf.setDb_engine(db_engine);
appConf.setDb_prefix(db_prefix);
appConf.setDiff_db_tables_service(diff_db_tables_service);
appConf.setField_prefix(field_prefix);
appConf.setLog_access_actions(log_access_actions);
appConf.setStructure_version(structure_version);
appConf.setType_prefix(type_prefix);
appConf.setUse_access_control(use_access_control);
appConf.setUse_cache_table_meta(use_cache_table_meta);
appConf.setShow_sys_types(show_sys_types);
appConf.setLazy_load_types(lazy_load_types);
appConf.setLazy_unload_types_time(lazy_unload_types_time);
//appConf.setSynchro_persist(synchro_persist);
//appConf.setSynchro_code(synchro_code);
appConf.setCreate_persistence(create_persistence);
appConf.setCreate_resources(create_resources);
appConf.setNotify_by_email(notify_by_email);
return appConf;
}
public Properties putToProperties(Properties prop) {
prop.put("structure_version", String.valueOf(getStructure_version()));
prop.put("field_prefix", getField_prefix());
prop.put("type_prefix", getType_prefix());
prop.put("add_app_id", String.valueOf(isAdd_app_id()));
prop.put("diff_db", String.valueOf(isDiff_db_tables_service()));
prop.put("use_access_control", String.valueOf(isUse_access_control()));
prop.put("use_cache_table_meta", String.valueOf(isUse_cache_table_meta()));
prop.put("log_access_actions", String.valueOf(isLog_access_actions()));
prop.put("db_engine", getDb_engine());
prop.put("db_prefix", getDb_prefix());
prop.put("show_sys_types", String.valueOf(isShow_sys_types()));
prop.put("lazy_load_types", String.valueOf(isLazy_load_types()));
prop.put("lazy_unload_types_time", String.valueOf(getLazy_unload_types_time()));
//prop.put("synchro_persist", String.valueOf(isSynchro_persist()));
//prop.put("synchro_code", String.valueOf(isSynchro_code()));
prop.put("create_persistence", String.valueOf(isCreate_persistence()));
prop.put("create_resources", String.valueOf(isCreate_resources()));
prop.put("notify_by_email", String.valueOf(isNotify_by_email()));
return prop;
}
public ApplicationConfig fromJSONObject(JSONObject conf) throws JSONException {
if (conf.has("structure_version")) {
setStructure_version(Float.parseFloat(conf.getString("structure_version")));
}
if (conf.has("field_prefix")) {
setField_prefix(conf.getString("field_prefix"));
}
if (conf.has("type_prefix")) {
setType_prefix(conf.getString("type_prefix"));
}
if (conf.has("add_app_id")) {
setAdd_app_id(conf.getBoolean("add_app_id"));
}
if (conf.has("diff_db")) {
setDiff_db_tables_service(conf.getBoolean("diff_db"));
}
if (conf.has("use_access_control")) {
setUse_access_control(conf.getBoolean("use_access_control"));
}
if (conf.has("use_cache_table_meta")) {
setUse_cache_table_meta(conf.getBoolean("use_cache_table_meta"));
}
if (conf.has("log_access_actions")) {
setLog_access_actions(conf.getBoolean("log_access_actions"));
}
if (conf.has("db_engine")) {
setDb_engine(conf.getString("db_engine"));
}
if (conf.has("db_prefix")) {
setDb_prefix(conf.getString("db_prefix"));
}
if (conf.has("show_sys_types")) {
setShow_sys_types(conf.getBoolean("show_sys_types"));
}
if (conf.has("lazy_load_types")) {
setLazy_load_types(conf.getBoolean("lazy_load_types"));
}
if (conf.has("lazy_unload_types_time")) {
setLazy_unload_types_time(conf.getInt("lazy_unload_types_time"));
}
/*
if (conf.has("synchro_persist")) {
setSynchro_persist(conf.getBoolean("synchro_persist"));
}
if (conf.has("synchro_code")) {
setSynchro_code(conf.getBoolean("synchro_code"));
}*/
if (conf.has("create_persistence")) {
setCreate_persistence(conf.getBoolean("create_persistence"));
}
if (conf.has("create_resources")) {
setCreate_resources(conf.getBoolean("create_resources"));
}
if (conf.has("notify_by_email")) {
setNotify_by_email(conf.getBoolean("notify_by_email"));
}
//сокращение для установки значений для 3-х параметров: create_persistence, create_resources, notify_by_email
if (conf.has("env")) {
boolean isEnv = conf.getBoolean("env");
if (isEnv) {
setCreate_persistence(false);
setCreate_resources(false);
setNotify_by_email(false);
}
}
return this;
}
public boolean isOldDataType() {
return structure_version < 1.2f;
}
public boolean isOldAppSourceDir() {
return structure_version < 1.5f;
}
public boolean isOldScriptAnnotations() {
return structure_version < 1.8f;
}
public boolean isCompileInMemory() {
return structure_version > 1.8f;
}
public boolean isPersistImports() {
return structure_version > 2.0f;
}
public boolean isPosibleLoadLazyLoadTypes() {
return structure_version > 2.1f;
}
public boolean isPersistJavaClassInDB() {
return structure_version > 1.9f;
}
public boolean isRefactoredApiServerPlace() {
return structure_version > 2.3f;
}
public boolean isOrderColumnInList() {
return structure_version > 2.6f;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy