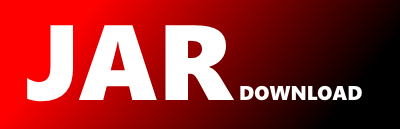
com.jelastic.api.development.response.ApplicationHostsResponse Maven / Gradle / Ivy
The newest version!
/*Server class MD5: 18060704cc030e7252acb3dad57f0a15*/
package com.jelastic.api.development.response;
import com.jelastic.api.Response;
import com.jelastic.api.json.JSONSerializable;
import com.jelastic.api.core.persistence.entity.ApplicationHost;
import org.json.JSONArray;
import org.json.JSONException;
import org.json.JSONObject;
import java.util.Collection;
import java.util.LinkedList;
/**
* @name Jelastic API Client
* @version 8.11.2
* @copyright Jelastic, Inc.
*/
public class ApplicationHostsResponse extends Response implements JSONSerializable {
private Collection hosts = null;
private ApplicationHost optimal = null;
public ApplicationHostsResponse(ApplicationHost optimal, Collection hosts) {
super(Response.OK);
this.optimal = optimal;
this.hosts = hosts;
}
public ApplicationHostsResponse() {
super(Response.OK);
}
public ApplicationHostsResponse(int result) {
super(result);
}
public ApplicationHostsResponse(int result, String error) {
super(result, error);
}
public ApplicationHostsResponse(Response resp) {
this.setResult(resp.getResult());
this.setError(resp.getError());
}
public Collection getHosts() {
return hosts;
}
public void setHosts(Collection hosts) {
this.hosts = hosts;
}
public ApplicationHost getOptimal() {
return optimal;
}
public void setOptimal(ApplicationHost optimal) {
this.optimal = optimal;
}
@Override
public JSONObject toJSON() {
JSONObject json = super.toJSON();
try {
if (optimal != null) {
json.put("optimal", new Host(optimal).toJSON());
}
if (hosts != null) {
JSONArray array = new JSONArray();
for (ApplicationHost host : hosts) {
array.put(new Host(host).toJSON());
}
json.put("hosts", array);
}
} catch (Exception ex) {
ex.printStackTrace();
try {
json.put("result", Response.ERROR_UNKNOWN).put("error", ex.toString());
} catch (JSONException exc) {
}
}
return json;
}
@Override
public ApplicationHostsResponse fromJSON(JSONObject json) {
Response response = super.fromJSON(json);
try {
if (json.has("optimal")) {
optimal = new ApplicationHost();
Host host = new Host().fromJSON(json.getJSONObject("optimal"));
optimal.setDomain(host.domain);
optimal.setIp(host.ip);
}
if (json.has("hosts")) {
JSONArray jsonHosts = json.getJSONArray("hosts");
hosts = new LinkedList();
for (int i = 0; i < jsonHosts.length(); i++) {
Host host = new Host().fromJSON(jsonHosts.getJSONObject(i));
ApplicationHost appHost = new ApplicationHost();
appHost.setDomain(host.domain);
appHost.setIp(host.ip);
hosts.add(appHost);
}
}
} catch (JSONException ex) {
response = new Response(ERROR_UNKNOWN, ex.toString());
}
setResult(response.getResult());
setError(response.getError());
return this;
}
public class Host {
public String domain = null, ip = null, context = null;
public Host() {
}
public Host(ApplicationHost host) {
this(host.getDomain(), host.getIp(), host.getContext());
}
public Host(String domain, String ip, String context) {
this.domain = domain;
this.ip = ip;
this.context = context;
}
public JSONObject toJSON() throws JSONException {
return new JSONObject().put("domain", domain).put("ip", ip).put("context", context);
}
public Host fromJSON(JSONObject json) throws JSONException {
if (json.has("domain")) {
domain = json.getString("domain");
}
if (json.has("ip")) {
ip = json.getString("ip");
}
if (json.has("context")) {
context = json.getString("ip");
}
return this;
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy