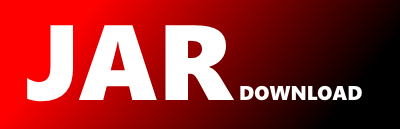
com.jelastic.api.development.response.ScriptEvalResponse Maven / Gradle / Ivy
The newest version!
package com.jelastic.api.development.response;
import com.jelastic.api.Response;
import com.jelastic.api.core.utils.JSONUtils;
import org.json.JSONException;
import org.json.JSONObject;
/**
*
* @author Ruslan Sinitskiy
*/
public class ScriptEvalResponse extends ScriptBuildResponse {
private Object response = null;
private Object debugTimes = null;
public ScriptEvalResponse() {
}
public ScriptEvalResponse(Object response) {
super();
this.response = response;
}
public ScriptEvalResponse(int result, String error) {
super(result, error);
}
public Object getResponse() {
return response;
}
public void setResponse(Object response) {
this.response = response;
}
public Object getDebugTimes() {
return debugTimes;
}
public void setDebugTimes(Object debugTimes) {
this.debugTimes = debugTimes;
}
@Override
public JSONObject toJSON() {
JSONObject json = super.toJSON();
try {
if (response == null) {
json.put("response", JSONObject.NULL);
} else {
json.put("response", toJSON(response));
}
if (debugTimes != null) {
json.put("debugTimes", toJSON(debugTimes));
}
} catch (Exception ex) {
ex.printStackTrace();
try {
json.put("result", ERROR_UNKNOWN).put("error", ex.toString());
} catch (JSONException exc) {
}
}
return json;
}
private Object toJSON(Object response) {
Object respJson = null;
if (response instanceof Response) {
respJson = ((Response) response).toJSON();
} else {
respJson = JSONUtils.toJSON(response);
}
return respJson;
}
@Override
public ScriptEvalResponse _fromJSON(JSONObject json) throws JSONException {
super._fromJSON(json);
if (json.has("response")) {
this.response = json.get("response");
}
if (json.has("debugTimes")) {
this.debugTimes = json.get("debugTimes");
}
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy