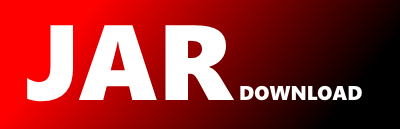
com.jelastic.api.development.response.interfaces.ArrayItem Maven / Gradle / Ivy
The newest version!
package com.jelastic.api.development.response.interfaces;
import com.jelastic.api.core.utils.DateUtils;
import org.json.JSONException;
import org.json.JSONObject;
import java.lang.reflect.Field;
import java.lang.reflect.InvocationTargetException;
import java.lang.reflect.Method;
import java.net.URL;
import java.security.*;
import java.security.cert.Certificate;
import java.text.ParseException;
import java.util.Date;
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
/**
*
* @author Alexey Shponarsky
*/
public abstract class ArrayItem {
public static final String ID = "id";
public void set(final String name, final Object value) {
Permissions perms = new Permissions();
perms.add(new AllPermission());
ProtectionDomain domain = new ProtectionDomain(new CodeSource((URL) null, (Certificate[]) null), perms);
AccessControlContext relaxedAcc = new AccessControlContext(new ProtectionDomain[]{domain});
final Class clazz = getClass();
final Object _this = this;
AccessController.doPrivileged(new PrivilegedAction
© 2015 - 2025 Weber Informatics LLC | Privacy Policy